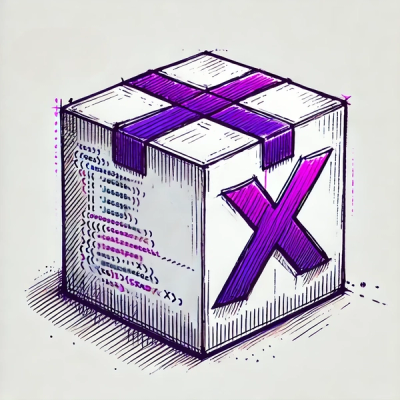
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
toxeus-node-sdk
Advanced tools
toxeus-node-sdk is a JavaScript-based server-side library that lets clients send requests to the Toxeus Solver API to model and solve optimization problems in real-time. The Toxeus Solver API is built on top of the AWS Cloud and uses the AWS Lambda and AW
toxeus-node-sdk is a JavaScript-based server-side library that lets clients send requests to the Toxeus Solver API to model and solve optimization problems in real-time. The Toxeus Solver API is built on top of the AWS Cloud and uses the AWS Lambda and AWS API Gateway services.
The official documentation for toxeus-node-sdk can be found here: https://docs.toxeus.org/docs/toxeus-node-sdk/
Name | Description |
---|---|
toxeus-sdk-demo | GitHub project with example code and demonstrations regarding the usage of the toxeus-node-sdk |
toxeus-cloud-platform | A customer-facing web platform that provides support for user registration, upgrade account, and generating api keys) |
toxeus-user-documentation | The official documentation for Toxeus includes guides for using toxeus-node-sdk, using the Toxeus Cloud Platform, and more. |
toxeus-npm-registry | The official source of Toxeus node packages related to the npm registry |
Name | Description |
---|---|
.vscode | VSCode settings file that contains linter, formatting tool, etc. |
src | Contains the main file where the source code of the program is written. |
src/auth | Contains methods that authenticate users with AWS Cognito and Amplify via their email and password |
src/model | Client and Model classes that contain default parameters and required arguments to match properties sent to the solver handler (Lambda Function) |
src/payload | Contains functions that check the size of a request payload |
src/S3 | Contains functions to read and write items to an S3 Bucket payload |
src/s3-templates | Contains request and response templates for s3 events, such as writing an item to s3 (problem file), and writing a request item to an S3 Bucket (solution file) |
test | A directory that contains all cases of unit tests |
Make sure that you are in toxeus-sdk-demo, then install the toxeus-node-sdk package by running the following command:
npm install toxeus-node-sdk
You will need to provide your toxeus credentials in order to use the toxeus cloud solver API. Install the dotenv module by running the following command in your terminal:
npm install dotenv
Then, perform the following commands to create an '.env' file for your environment variables:
cp .env.example .env
This will copy the .env.example file and create a new '.env' file, where you will input your toxeus username and password in their respective fields:
TOXEUS_USERNAME=email@gmail.com
TOXEUS_PASSWORD=your_password
Note If you don't have a toxeus account, you can create one here: https://solver.toxeus.org/
To setup your environment variables, configure the dotenv package by adding the following line of code at the top of your demo script:
require("dotenv").config();
The toxeus client is the main object that you will use to interact with the toxeus cloud solver API. The toxeus client is created by calling the toxeus function. The toxeus function takes in two parameters: your toxeus username and your toxeus password.
Import the Client module from the toxeus-node-sdk package by adding the following line of code to the top of your demo application:
const { Client } = require('toxeus-node-sdk');
Then, add the line below to the top of your file to create a new toxeus client:
const toxeus = new Client(process.env.TOXEUS_USERNAME, process.env.TOXEUS_PASSWORD);
In order to solve any problem, we need to formulate an optimization model of that problem with decision variables, constraints, and the identify the overall goal of what we're trying to do. In this example, we will be modeling a simple optimization problem with one decision variable, one constraint, and one objective function.
First, import the Model module from the toxeus-node-sdk package by adding the following line of code to the top of your demo application:
const { Model } = require('toxeus-node-sdk');
Then, create a new model by calling the Model constructor. The Model constructor takes in one parameter: the type of problem you are trying to solve. In this example, we are solving an optimization problem, so we will pass in the string "optimization" as the parameter.
const model = new Model(problem_type = "optimization");
An important step to formulating an optimization model is to define the decisions to make with respect to context of the situation involved. The goal is to optimize our decision variables in a way that reaches our objective. Decision variables can take the form of "continuous" or "discrete"
Use the addDecisionVariable method to add a decision variable to your optimization model.
model.addDecisionVariable({
name: "y",
type: "continuous",
lower_bound: 0,
upper_bound: 0.1,
initial_region_to_select_starting_points_from: {
lower_bound: 0,
upper_bound: 0.05
}
});
The next thing we need to do is to set the objective function. The objective function is the function that we want to minimize or maximize.
model.setObjective({
expression: "(1/x+y+z-3)^2+(x+1/y+z-3)^2+(x+y+1/z-3+0+0)^2",
type: "minimize"
});
The setObjectiveFunction function takes in the type of the objective function (either "minimize" or "maximize") and the objective function as parameters:
The next thing we need to do is to add constraints to our model. Constraints are the conditions that our problem must satisfy. For example, a certain type of constraint may include a budget, limited number of workers, number of trucks, etc.
model.addConstraint("x + y + z <= 10");
The addConstraint function takes in the type of the constraint (either "less than", "greater than", or "equal to"), the constraint, and the right-hand side of the constraint as parameters:
toxeus.Solve(model);
const { Client, Model } = require('toxeus-node-sdk');
const toxeus = new Client(TOXEUS_EMAIL, TOXEUS_PASSWORD);
const model = new Model(problem_type = "optimization");
model.problem_name = "Optimization Model";
model.problem_description = "Demonstrating how to send an nse model to the Toxeus API to be solved";
model.problem_tags = ["sne", "problem"];
model.request_type = "asynchronous";
model.number_of_starting_points = 5;
model.maximum_acceptable_error = 10;
model.addDecisionVariable({
name: "x",
type: "continuous",
lower_bound: 10,
upper_bound: 15,
initial_region_to_select_starting_points_from: {
lower_bound: 11,
upper_bound: 14
}
});
model.addDecisionVariable({
name: "y",
type: "continuous",
lower_bound: 0,
upper_bound: 0.1,
initial_region_to_select_starting_points_from: {
lower_bound: 0,
upper_bound: 0.05
}
});
model.addDecisionVariable({
name: "z",
type: "continuous",
lower_bound: 0,
upper_bound: 15,
initial_region_to_select_starting_points_from: {
lower_bound: 0.1,
upper_bound: 10
}
});
model.addConstraint("x + y + z <= 10");
model.setObjective({
expression: "(1/x+y+z-3)^2+(x+1/y+z-3)^2+(x+y+1/z-3+0+0)^2",
type: "minimize"
});
toxeus.Solve(model);
FAQs
t8xeus-node-sdk is a powerful Node.js library for interacting with the Toxeus Cloud Solver API. It enables users to model and solve complex nonlinear systems and optimization problems in real-time. Features include secure authentication, problem modeling,
The npm package toxeus-node-sdk receives a total of 21 weekly downloads. As such, toxeus-node-sdk popularity was classified as not popular.
We found that toxeus-node-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.