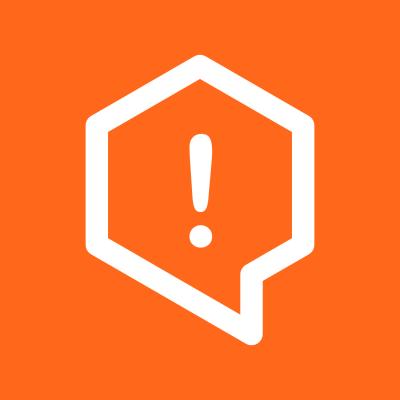
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
transitory
Advanced tools
In-memory cache with LFU eviction, expiration, automatic loading and metrics
Transitory is a in-memory caching library for JavaScript. Transitory provides caches with eviction based on frequency and recency, expiration, automatic loading and metrics.
const transitory = require('transitory');
const cache = transitory()
.maxSize(1000)
.build();
cache.set('key', { value: 10 });
cache.set(1234, 'any value');
const value = cache.get('key');
The caches in this library are designed to have a high hit rate by evicting entries in the cache that are not frequently used. Transitory implements W-TinyLFU as its eviction policy which is a LFU policy that provides good hit rates for many use cases.
See Performance in the wiki for comparisons of the hit rate of Transitory to other libraries.
There are a few basic things that all caches support.
cache.maxSize: number
- the maximum size of the cache or -1
if boundlesscache.size: number
- the number of items in the cachecache.get(key): mixed|null
- get a cached valuecache.getIfPresent(key): mixed|null
- get a cached value without optional loading (see below)cache.has(key): boolean
- check if the given key exists in the cachecache.set(key, value): mixed|null
- set a value in the cache. Returns the previous value or null
cache.delete(key): mixed|null
- delete a value in the cache. Returns the removed value or null
Caches are created via a builder that helps with adding on all requested functionality and returning a cache.
A builder is created by calling the imported function:
const transitory = require('transitory');
const builder = transitory();
Calls on the builder can be chained:
transitory().maxSize(100).loading().build();
Caches can be limited to a certain size. This type of cache will evict the least frequently used items when it reaches its maximum size.
const cache = transitory()
.maxSize(100)
.build();
It is also possible to change how the size of each entry in the cache is calculated. This can be used to create a better cache if your entries vary in their size in memory.
const cache = transitory()
.maxSize(2000)
.withWeigher((key, value) => value.length)
.build();
The size of an entry is evaluated when it is added to the cache so weighing works best with immutable data. Transitory includes a weigher for estimated memory:
const cache = transitory()
.maxSize(5242880)
.withWeigher(transitory.memoryUsageWeigher)
.build();
Limiting the maximum amount of time an entry can exist in the cache can be done
by using expireAfterWrite(timeInMs)
. Entries are lazy evaluated and will
be removed when the values are set or deleted from the cache.
const cache = transitory()
.maxSize(100)
.expireAfterWrite(5000) // 5 seconds
.build();
expireAfterWrite
can also take a function that should return the maximum age
of the entry in milliseconds:
const cache = transitory()
.maxSize(100)
.expireAfterWrite((key, value) => 5000)
.build();
Caches can be made to automatically load values if they are not in the cache. This type of caches relies heavily on the use of promises.
With a global loader:
const cache = transitory()
.withLoader(key => loadSlowData(key))
.done();
cache.get(781)
.then(data => handleLoadedData(data))
.catch(err => handleError(err));
cache.get(1234, specialLoadingFunction)
Without a global loader:
const cache = transitory()
.loading()
.done();
cache.get(781, key => loadSlowData(key))
.then(data => handleLoadedData(data))
.catch(err => handleError(err));
Loading caches can be combined with other things such as maxSize
.
withLoader
on the builder can be used with our without a function that loads
missing items. If provided the function may return a Promise or value.
API extensions for loading caches:
cache.get(key): Promise
- get
always returns a promise that will eventually resolve to the loaded value or failcache.get(key, loader: Function): Promise
- provide a custom function that loads the value if needed, should return a Promise or a value. Example: cache.get(500, key => key / 5)
would resolve to 100.You can track the hit rate of the cache by activating support for metrics:
const cache = transitory()
.metrics()
.done();
const metrics = cache.metrics;
console.log('hitRate=', metrics.hitRate);
console.log('hits=', metrics.hits);
console.log('misses=', metrics.misses);
Caches support a single removal listener that will be notified when items in the cache are removed.
const RemovalCause = transitory.RemovalCause;
const cache = transitory()
.withRemovalListener((key, value, reason) => {
switch(reason) {
case RemovalCause.EXPLICIT:
// The user of the cache requested something to be removed
break;
case RemovalCause.REPLACED:
// A new value was loaded and this value was replaced
break;
case RemovalCause.SIZE:
// A value was evicted from the cache because the max size has been reached
break;
case RemovalCause.EXPIRED:
// A value was removed because it expired due to its autoSuggest
break;
}
})
.build();
FAQs
In-memory cache with high hit rates via LFU eviction. Supports time-based expiration, automatic loading and metrics.
The npm package transitory receives a total of 631 weekly downloads. As such, transitory popularity was classified as not popular.
We found that transitory demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.