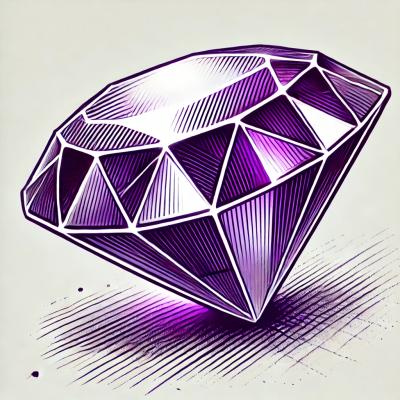
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
transparency
Advanced tools
Transparency is a minimal template engine for browsers. It maps JSON objects to DOM elements with zero configuration.
Transparency is a (client-side) template engine which binds data to DOM with zero configuration. Just call .render(data)
.
<div id="template">
<span class="greeting"></span>
<span class="name"></span>
</div>
var hello = {
greeting: 'Hello',
name: 'world!'
};
$('#template').render(hello);
<div id="template">
<span class="greeting">Hello</span>
<span class="name">world!</span>
</div>
(*) Take with a grain of salt, as "real-world performance" isn't that easy to define or measure. Anyway, jsperf.com should give an idea: http://jsperf.com/transparency-vs-handlebars-finite-list/3 http://jsperf.com/transparency-vs-handlebars-infinite-list/3 http://jsperf.com/dom-vs-innerhtml-based-templating/366
If interested, see other performance tests at browser
folder. See also
Frequently asked questions.
Demo website with interactive examples.
Get the compiled and minified version and include it to your application. jQuery is optional, but if you happen to use it, Transparency registers itself as a plugin.
Node users can also install via NPM:
npm install transparency
<script src="js/jquery-1.7.1.min.js"></script>
<script src="js/transparency.min.js"></script>
For server-side use, see spec
folder and the awesome jsdom for the details.
Transparency binds JavaScript objects to DOM a element by id
, class
,name
attribute and
data-bind
attribute. Values are escaped before rendering.
Template:
<div id="container">
<div id="hello"></div>
<div class="goodbye"></div>
<input type="text" name="greeting" />
<button class="hi-button" data-bind="hi-label"></button>
</div>
Javascript:
var hello = {
hello: 'Hello',
goodbye: '<i>Goodbye!</i>',
greeting: 'Howdy!',
'hi-label': 'Terve!' // Finnish i18n
};
// with jQuery
$('#container').render(hello);
// ..or without
Transparency.render(document.getElementById('container'), hello);
Result:
<div class="container">
<div id="hello">Hello</div>
<div class="goodbye">lt;i>Goodbye!</i></div>
<input type="text" name="greeting" value="Howdy!" />
<button class="hi-button" data-bind="hi-label">Terve!</button>
</div>
Template:
<ul id="activities">
<li class="activity"></li>
</ul>
Javascript:
var activities = [
{activity: 'Jogging'},
{activity: 'Gym'},
{activity: 'Sky Diving'},
];
$('#activities').render(activities);
// or
Transparency.render(document.getElementById('activities'), activities);
Result:
<ul id="activities">
<li class="activity">Jogging</li>
<li class="activity">Gym</li>
<li class="activity">Sky Diving</li>
</ul>
Template:
<div>
<div class="comments">
<span></span>
</div>
</div>
Javascript:
var comments = ["That rules", "Great post!"]
$('.comments').render(comments);
Result:
<div>
<div class="comments">
<span>That rules</span>
<span>Great post!</span>
</div>
</div>
listElement
classTemplate:
<div>
<div class="comments">
<label>comment</label><span class="listElement"></span>
</div>
</div>
Javascript:
var comments = ["That rules", "Great post!"]
$('.comments').render(comments);
Result:
<div>
<div class="comments">
<label>comment</label><span class="listElement">That rules</span>
<label>comment</label><span class="listElement">Great post!</span>
</div>
</div>
Template:
<div class="container">
<h1 class="title"></h1>
<p class="post"></p>
<div class="comments">
<div class="comment">
<span class="name"></span>
<span class="text"></span>
</div>
</div>
</div>
Javascript:
var post = {
title: 'Hello World',
post: 'Hi there it is me',
comments: [ {
name: 'John',
text: 'That rules'
}, {
name: 'Arnold',
text: 'Great post!'
}
]
};
$('.container').render(post);
Result:
<div class="container">
<h1 class="title">Hello World</h1>
<p class="post">Hi there it is me</p>
<div class="comments">
<div class="comment">
<span class="name">John</span>
<span class="text">That rules</span>
</div>
<div class="comment">
<span class="name">Arnold</span>
<span class="text">Great post!</span>
</div>
</div>
</div>
Template:
<div class="person">
<div class="firstname"></div>
<div class="lastname"></div>
<div class="address">
<div class="street"></div>
<div class="zip"><span class="city"></span></div>
</div>
</div>
Javascript:
var person = {
firstname: 'John',
lastname: 'Wayne',
address: {
street: '4th Street',
city: 'San Francisco',
zip: '94199'
}
};
$('.person').render(person);
Result:
<div class="container">
<div class="firstname">John</div>
<div class="lastname">Wayne</div>
<div class="address">
<div class="street">4th Street</div>
<div class="zip">94199<span class="city">San Francisco</span></div>
</div>
</div>
Directives are used for manipulating text or html values and setting element attributes.
In addition to having an access to the current data object through this
, directives also receive
index number and current element as a parameter, which makes it easy to, e.g., add even
and odd
classes or
hide elements.
The return value of a directive function can be either string or object. If the return value is string, it is assigned
to the matching elements as text content. If the return value is an object, keys can be either text
, html
or any
valid element attribute, e.g., class
, src
or href
. Values are assigned accordingly to the matching elements.
If both text
and html
are present, html
overrides the text content.
Template:
<div class="person">
<span class="name"></span>
<a class="email"></a>
</div>
Javascript:
person = {
firstname: 'Jasmine',
lastname: 'Taylor',
email: 'jasmine.tailor@example.com'
};
directives =
name: function(element, index) { return this.firstname + " " + this.lastname; }
email: function(element, index) { return {href: "mailto:" + this.email}; }
};
$('.person').render(person, directives);
Result:
<div class="person">
<span class="name">Jasmine Taylor</span>
<a class="email" href="mailto:jasmine.tailor@example.com">jasmine.tailor@example.com</a>
</div>
Template:
<div class="person">
<span class="name"></span>
<span class="email"></span>
<div class="friends">
<div class="friend">
<span class="name"></span>
<span class="email"></span>
</div>
</div>
</div>
Javascript:
person = {
firstname: 'Jasmine',
lastname: 'Taylor',
email: 'jasmine.taylor@example.com',
friends: [ {
firstname: 'John',
lastname: 'Mayer',
email: 'john.mayer@example.com'
}, {
firstname: 'Damien',
lastname: 'Rice',
email: 'damien.rice@example.com'
}
]
};
nameDecorator = function() { return {html: "<b>" + this.firstname + " " + this.lastname + "</b>"}; };
directives = {
name: nameDecorator,
friends: {
name: nameDecorator
}
};
$('.person').render(person, directives);
Result:
<div class="person">
<span class="name"><b>Jasmine Taylor</b></span>
<span class="email">jasmine.taylor@example.com</span>
<div class="friends">
<div class="friend">
<span class="name"><b>John Mayer</b></span>
<span class="email">john.mayer@example.com</span>
</div>
<div class="friend">
<span class="name"><b>Damien Rice</b></span>
<span class="email">damien.rice@example.com</span>
</div>
</div>
</div>
http://leonidas.github.com/transparency/ is great place to fiddle around with your data and templates.
To enable debug mode, call .render
with a {debug: true}
config and open the javascript console.
$('container').render(data, {}, {debug: true});
You need node.js 0.6.x and npm.
Install dependencies:
npm install
npm install -g uglify-js
npm install -g coffee-script
Run tests
npm test
Run tests during development for more verbose assertion output
node_modules/jasmine-node/bin/jasmine-node --coffee --verbose spec
Generate Javascript libs
cake build
Use debugger statement to debug spec scripts.
All the following are appreciated, in an asceding order of preference
In case the contribution is going to change Transparency API, please create a ticket first in order to discuss and agree on design.
There's an article regarding the original design and implementation. It might be worth reading as an introduction.
FAQs
Transparency is a minimal template engine for browsers. It maps JSON objects to DOM elements with zero configuration.
The npm package transparency receives a total of 0 weekly downloads. As such, transparency popularity was classified as not popular.
We found that transparency demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.