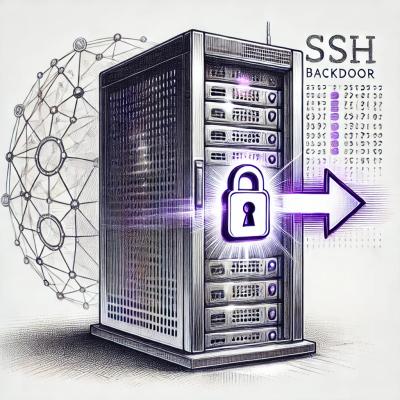
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
ts-create-index
Advanced tools
Creates Typescript ./index.ts file in target directories that imports and exports all sibling files and directories.
Forked from: https://github.com/gajus/create-index
npm i -D ts-create-index
or
yarn add -D ts-create-index
export * from './foo';
(instead of export { default as foo } from './foo.ts';
)TsCreateIndexWebpackPlugin
// webpack.config.js
import { TsCreateIndexWebpackPlugin } from 'ts-create-index';
module.exports = {
plugins: [
// Whenever webpack recompiles, a new index.ts file will be generated in the root of these dirs
new TsCreateIndexWebpackPlugin({ dirs: ['src/components'] }),
]
}
ts-create-index
program creates (and maintains) ES6 ./index.ts
file in target directories that imports and exports sibling files and directories.
> tree ./
./
├── bar.ts
└── foo.ts
0 directories, 2 files
> ts-create-index ./
[13:17:34] Target directories [ './' ]
[13:17:34] Update index: false
[13:17:34] ./index.ts [created index]
[13:17:34] Done
> tree
.
├── bar.ts
├── foo.ts
└── index.ts
0 directories, 3 files
This created index.ts
with:
// @ts-create-index
export * from './bar';
export * from './foo';
Lets create a new file and re-run ts-create-index
:
> touch baz.ts
> tree ./
./
├── bar.ts
├── baz.ts
├── foo.ts
└── index.ts
0 directories, 4 files
> ts-create-index ./
[13:21:55] Target directories [ './' ]
[13:21:55] Update index: false
[13:21:55] ./index.ts [updated index]
[13:21:55] Done
This have updated index.ts
file:
// @ts-create-index
export * from './bar';
export * from './baz';
export * from './foo';
npm install ts-create-index
ts-create-index --help
Options:
--recursive, -r Create/update index files recursively. Halts on any
unsafe "index.ts" files. [boolean] [default: false]
--ignoreUnsafe, -i Ignores unsafe "index.ts" files instead of halting.
[boolean] [default: false]
--ignoreDirectories, -d Ignores importing directories into the index file,
even if they have a safe "index.ts".
[boolean] [default: false]
--update, -u Updates only previously created index files
(recursively). [boolean] [default: false]
--banner Add a custom banner at the top of the index file
[string]
--extensions, -x Allows some extensions to be parsed as valid source.
First extension will always be preferred to homonyms
with another allowed extension.
[array] [default: ["ts"]]
--outputFile, -o Output file [string] [default: "index.ts"] [array] [default: ["ts"]]
Examples:
ts-create-index ./src ./src/utilities Creates or updates an existing
ts-create-index index file in the target
(./src, ./src/utilities) directories.
ts-create-index --update ./src ./tests Finds all ts-create-index index files in
the target directories and descending
directories. Updates found index
files.
ts-create-index ./src --extensions ts tsx Creates or updates an existing
ts-create-index index file in the target
(./src) directory for both .ts and
.tsx extensions.
ts-create-index
Programmaticallyimport {writeIndex} from 'ts-create-index';
/**
* @type {Function}
* @param {Array<string>} directoryPaths
* @throws {Error} Directory "..." does not exist.
* @throws {Error} "..." is not a directory.
* @throws {Error} "..." unsafe index.
* @returns {boolean}
*/
writeIndex;
Note that the writeIndex
function is synchronous.
import {findIndexFiles} from 'ts-create-index';
/**
* @type {Function}
* @param {string} directoryPath
* @returns {Array<string>} List of directory paths that have ts-create-index index file.
*/
findIndexFiles;
Since Gulp can ran arbitrary JavaScript code, there is no need for a separate plugin. See Using ts-create-index
Programmatically.
import {writeIndex} from 'ts-create-index';
gulp.task('ts-create-index', () => {
writeIndex(['./target_directory']);
});
Note that the writeIndex
function is synchronous.
ts-create-index
program will look into the target directory.
If there is no ./index.ts
, it will create a new file, e.g.
// @ts-create-index
Created index file must start with // @ts-create-index\n\n
. This is used to make sure that ts-create-index
does not accidentally overwrite your local files.
If there are sibling files, index file will import
them and export
, e.g.
children-directories-and-files git:(master) ✗ ls -lah
total 0
drwxr-xr-x 5 gajus staff 170B 6 Jan 15:39 .
drwxr-xr-x 10 gajus staff 340B 6 Jan 15:53 ..
drwxr-xr-x 2 gajus staff 68B 6 Jan 15:29 bar
drwxr-xr-x 2 gajus staff 68B 6 Jan 15:29 foo
-rw-r--r-- 1 gajus staff 0B 6 Jan 15:29 foo.ts
Given the above directory contents, ./index.ts
will be:
// @ts-create-index
import * from './bar';
import * from './foo';
export {
bar,
foo
};
When file has the same name as a sibling directory, file import
takes precedence.
Directories that do not have ./index.ts
in themselves will be excluded.
When run again, ts-create-index
will update existing ./index.ts
if it starts with // @ts-create-index\n\n
.
If ts-create-index
is executed against a directory that contains ./index.ts
, which does not start with // @ts-create-index\n\n
, an error will be thrown.
--update
ts-create-index
can ignore files in a directory if ./index.ts
contains special object with defined ignore
property which takes an array
of regular expressions
defined as strings
, e.g.
> cat index.ts
// @ts-create-index {"ignore": ["/baz.ts$/"]}
> tree ./
./
├── bar.ts
├── baz.ts
├── foo.ts
└── index.ts
0 directories, 4 files
Given the above directory contents, after running ts-create-index
with --update
flag, ./index.ts
will be:
// @ts-create-index {"ignore": ["/baz.ts$/"]}
import * from './bar';
import * from './foo';
export {
bar,
foo
};
FAQs
Creates Typescript ./index.ts file in target directories that imports and exports all sibling files and directories.
The npm package ts-create-index receives a total of 227 weekly downloads. As such, ts-create-index popularity was classified as not popular.
We found that ts-create-index demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.