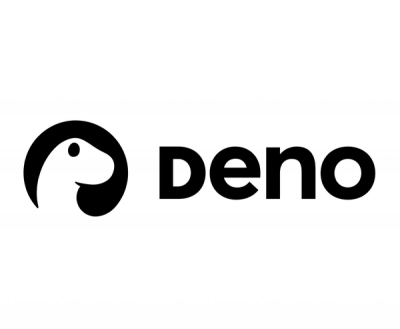
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
ts-event-bus
Advanced tools
Distributed messaging in Typescript
ts-event-bus
is a lightweight distributed messaging system. It allows several modules, potentially distributed over different runtime spaces to communicate through typed messages.
Using ts-event-bus
starts with the declaration of the interface that your components share:
// MyEvents.ts
import { slot, Slot } from 'ts-event-bus'
const MyEvents = {
sayHello: slot<string>(),
getTime: slot<null, string>(),
multiply: slot<{a: number, b: number}, number>(),
ping: slot<void>(),
}
export default MyEvents
Your components will then instantiate an event bus based on this declaration, using whatever channel they may want to communicate on.
If you specify no Channel
, it means that you will exchange events in the same memory space.
For instance, one could connect two node processes over WebSocket:
// firstModule.EventBus.ts
import { createEventBus } from 'ts-event-bus'
import MyEvents from './MyEvents.ts'
import MyBasicWebSocketChannel from './MyBasicWebSocketChannel.ts'
const EventBus = createEventBus({
events: MyEvents,
channels: [ new MyBasicWebSocketChannel('ws://your_host') ]
})
export default EventBus
// secondModule.EventBus.ts
import { createEventBus } from 'ts-event-bus'
import MyEvents from './MyEvents.ts'
import MyBasicWebSocketChannel from './MyBasicWebSocketChannel.ts'
const EventBus = createEventBus({
events: MyEvents,
channels: [ new MyBasicWebSocketChannel('ws://your_host') ]
})
Once connected, the clients can start by using the slots on the event bus
// firstModule.ts
import EventBus from './firstModule.EventBus.ts'
// Triggering an event always returns a promise
EventBus.sayHello('michel').then(() => {
...
})
EventBus.getTime().then((time) => {
...
})
EventBus.multiply({a: 2, b: 5 }).then((result) => {
...
})
EventBus.ping()
// secondModule.ts
import EventBus from './secondModule.EventBus.ts'
EventBus.ping().on(() => {
console.log('pong')
})
EventBus.sayHello.on(name => {
console.log(`${name} said hello!`)
})
// Event subscribers can respond to the event synchronously (by returning a value)
EventBus.getTime.on(() => new Date().toString)
// Or asynchronously (by returning a Promise that resolves with the value).
EventBus.multiply.on(({ a, b }) => new Promise((resolve, reject) => {
AsynchronousMultiplier(a, b, (err, result) => {
if (err) {
return reject(err)
}
resolve(result)
})
}))
Calls and subscriptions on slots are typechecked
EventBus.multiply({a: 1, c: 2}) // Compile error: property 'c' does not exist on type {a: number, b: number}
EventBus.multiply.on(({a, b}) => {
if (a.length > 2) { // Compile error: property 'length' does not exist on type 'number'
...
}
})
You can combine events from different sources.
import { combineEvents } from 'ts-event-bus'
import MyEvents from './MyEvents.ts'
import MyOtherEvents from './MyOtherEvents.ts'
const MyCombinedEvents = combineEvents(
MyEvents,
MyOtherEvents,
)
export default MyCombinedEvents
ts-event-bus
comes with an abstract class GenericChannel.
To implement your own channel create a new class extending GenericChannel
, and call the method given by the abstract class: _connected(), _disconnected(), _error(e: Error) and _messageReceived(data: any).
Basic WebSocket Channel example:
import { GenericChannel } from 'ts-event-bus'
export class MyBasicWebSocketChannel extends GenericChannel {
private _ws: WebSocket | null = null
private _host: string
constructor(host: string) {
super()
this._host = host
this._init()
}
private _init(): void {
const ws = new WebSocket(this._host)
ws.onopen = (e: Event) => {
this._connected()
this._ws = ws
}
ws.onerror = (e: Event) => {
this._ws = null
this._error(e)
this._disconnected()
setTimeout(() => {
this._init()
}, 2000)
}
ws.onclose = (e: CloseEvent) => {
if (ws === this._ws) {
this._ws = null
this._disconnected()
this._init()
}
}
ws.onmessage = (e: MessageEvent) => {
this._messageReceived(e.data)
}
}
}
FAQs
Distributed messaging in Typescript
We found that ts-event-bus demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.