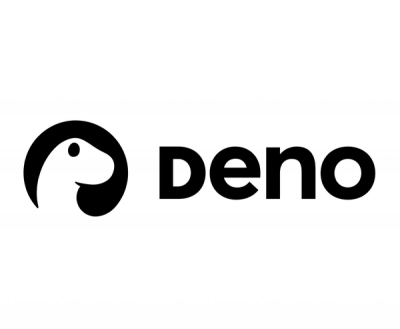
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
ts-guard-decorator
Advanced tools
TypeScript decorator for running a check before running a method.
npm install --save ts-guard-decorator
import guard from 'ts-guard-decorator';
class MyClass {
// Don't run `myFunc` if `window` doesn't exist
@guard(typeof window !== 'undefined')
myFunc() {
// ...
}
}
This is equivalent to writing:
class MyClass {
myFunc() {
if (typeof window === 'undefined') {
return;
}
// ...
}
}
The guard accepts 2 arguments:
true
or false
) indicating whether the method should run.function myGuardFunc(arg1: any, arg2: any): boolean {
return arg1 === arg2;
}
class MyClass {
@guard(true)
myFunc1() {
return true;
} //=> true
@guard(false)
myFunc2() {
return true;
} //=> undefined
@guard(1 === 1)
myFunc3() {
return true;
} //=> true
@guard(1 === 2, 'hello')
myFunc4() {
return true;
} //=> "hello"
@guard(myGuardFunc(1, 1), 'hello')
myFunc5() {
return true;
} //=> true
@guard(myGuardFunc(1, 2), 'hello')
myFunc6() {
return true;
} //=> "hello"
}
FAQs
Decorator for running a check before running a method.
We found that ts-guard-decorator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.