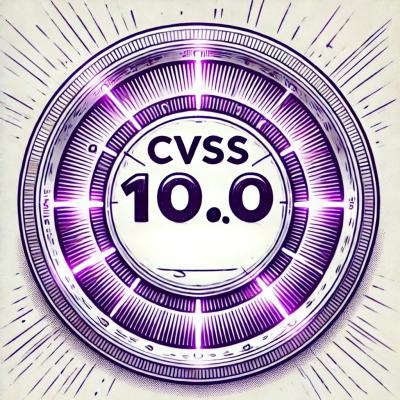
Security News
cURL Project and Go Security Teams Reject CVSS as Broken
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
ts-jackson
Advanced tools
A typescript library to deserialize and serialize json into classes. You can use different path pattern to resolve deeply nested structures. Every path pattern provided by lodash/get|set object is supported. Check out [src/examples](https://github.com/Elj
A typescript library to deserialize and serialize json into classes. You can use different path pattern to resolve deeply nested structures. Every path pattern provided by lodash/get|set object is supported. Check out src/examples for examples.
npm install ts-jackson --save
# or
yarn add ts-jackson
For tsconfig.json file set experimentalDecorators and emitDecoratorMetadata to true to allow support for the decorators:
{
"compilerOptions": {
"emitDecoratorMetadata": true,
"experimentalDecorators": true
}
}
import { JsonProperty, Serializable, deserialize, serialize } from 'typescript-json-serializer';
import { JsonProperty, Serializable, deserialize, serialize } from 'typescript-json-serializer';
To mark your class as serializable wrap your class with Serializable decorator:
@Serializable()
class Class {}
/**
* JsonProperty params
*
* @param {string} path -- path pattern for the property
* supports every pattern provided by lodash/get|set object
* @param {boolean} required throws an Error if json is missing required property
* @param {Function} type Optional. In most cases there is no need to specify
* type explicitly
* @param {Function} elementType due to reflect-metadata restriction one should
* explicitly set elementType property to correctly serialize/deserialize Array
* or Set values
* @param {Function} validate function for validating json values. Throws an error
* if property fails to pass validate check
* @param {Function} deserialize function for custom deserialization
* @param {Function} serialize function for custom serialization
*/
type Params<P> = {
path?: string
required?: boolean
type?: new (...params: Array<unknown>) => unknown
elementType?: new (...params: Array<unknown>) => unknown
validate?: (property: P) => boolean
deserialize?: (jsonValue: unknown) => P
serialize?: (property: P) => unknown
}
@JsonProperty(options: Options | string)
The path property can be set in a few different ways:
// By inferring path from the property name:
class Cat {
@JsonProperty()
name: string
}
// As a string argument
class Track {
@JsonProperty('duration_ms')
durationMs: number
}
// As an Option parameter
class Track {
@JsonProperty({
path: 'duration_ms'
})
durationMs: number
}
Path property supports different formats for resolving deeply nested structures provided by lodash `_.set(object, path, value)
Resolving deeply nested structures:
const trackJson = {
track: {
id: 'some id',
},
}
@Serializable()
class Track {
@JsonProperty('track.id')
readonly id: string
}
const deserialized = deserialize(trackJson, Track)
// Track { id: 'some id' }
serialize(deserialized)
// { track: { id: 'some id' } }
Resolving array structures
const jsonData = {
images: {
items: [
{
height: 300,
url:
'https://i.scdn.co/image/ab67616d0000b27380368f0aa8f90c51674f9dd2',
width: 300,
},
{
height: 640,
url:
'https://i.scdn.co/image/ab67616d00001e0280368f0aa8f90c51674f9dd2',
width: 640,
},
],
},
}
@Serializable()
class Playlist {
@JsonProperty('images.items[1]')
readonly backgroundImage: Image
}
const deserialized = deserialize(jsonData, Playlist)
// Playlist {
// backgroundImage: Image {
// height: 640,
// width: 640,
// url: 'https://i.scdn.co/image/ab67616d00001e0280368f0aa8f90c51674f9dd2'
// }
// }
const serialized = serialize(deserialized)
// {
// images: {
// items: [undefined, {
// height: 640,
// width: 640,
// url: 'https://i.scdn.co/image/ab67616d00001e0280368f0aa8f90c51674f9dd2',
// }],
// },
// }
For more patterns for resolving structures check out lodash/get docs.
/**
* Function to deserialize json to Serializable class
*
* @param {Record<string, unknown>} json
* @param serializableClass Class to which json should be serialized
* @param args an arguments to be provided to constructor.
* For example Cat(readonly name, readonly color)
* deserialize({}, Cat, 'Moon', 'black')
*/
export default function deserialize<T, U extends Array<unknown>>(
json: Record<string, unknown>,
serializableClass: new (...params: [...U]) => T,
...args: U
): T { }
/**
* Function to serialize Serializable class to json
*
* @param {Function} instance serializable instance
* @returns {Record<string, unknown>} json
*/
export default function serialize<
T extends new (...args: unknown[]) => unknown
>(instance: InstanceType<T>): Record<string, unknown> {
const trackJson = {
track: {
id: 'some id',
},
}
@Serializable()
class Track {
@JsonProperty('track.id')
readonly id: string
}
const deserializedClassIntance = deserialize(trackJson, Track)
const serializedJson = serialize(deserializedClassIntance)
// using constructor params:
@Serializable()
class Image {
@JsonProperty({ required: true })
readonly url: string
constructor(readonly width: number, readonly height: number) {
}
}
deserialize({url: 'url'}, Image, 5, 3)
const deserializedClassIntance = deserialize({url: 'some url'}, Image, 5, 4)
const serializedJson = serialize(deserializedClassIntance)
FAQs
`ts-jackson` is a powerful TypeScript library designed for efficient JSON serialization and deserialization into classes. It leverages lodash's path patterns to effortlessly resolve deeply nested structures. Explore the `src/examples` directory for practi
The npm package ts-jackson receives a total of 300 weekly downloads. As such, ts-jackson popularity was classified as not popular.
We found that ts-jackson demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Security News
Biden's executive order pushes for AI-driven cybersecurity, software supply chain transparency, and stronger protections for federal and open source systems.