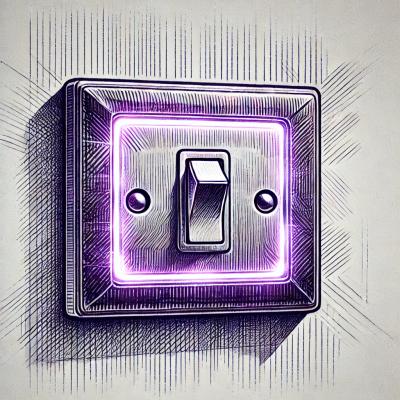
Research
Security News
Kill Switch Hidden in npm Packages Typosquatting Chalk and Chokidar
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Tiny library to improve error handling in TS using Rust-inspired types.
npm install -D ts-result
yarn add --dev ts-result
pnpm add -D ts-result
Result type is convenient to use as a return type of a method that may fail.
In Result<T, E>
:
T
specifies success result type, which can be any typeE
specifies error type, which is constrained by Error | string | void | undefined
.Err()
and Ok()
are used as a return type enforcers.
function mayFail(isFailed: boolean): Result<number, Error> {
if (isFailed) {
return Err(new Error("Function failed"));
}
return Ok(123);
}
As a result we get an object which will either be {ok: true, data: T}
or {ok: false, error: E}
Note: Ok()
and Err()
can be used with no values if T
/E
were specified as void
or undefined
function getEmptyResult(isFailed: boolean): Result<void, void> {
if (isFailed) {
return Err();
}
return Ok();
}
Result<T, E>
can be handled manually:
const result = mayFail(true);
if (!result.ok) {
throw result.error;
}
console.log(result.data);
However it's much easier to use helper methods to quickly handle the result:
throw(message?: string)
- throws an error (with an optional custom error message) or returns an unwrapped resultor(value: T)
- returns an unwrapped result or a back-up valueelse(callback: (error: E) => T)
- returns an unwrapped result or executes callback that returns back-up value which can be based on provided error// -- throw()
mayFail(true).throw(); // returns 123
mayFail(false).throw(); // throws an Error with default message
mayFail(false).throw("My Message"); // throws an Error with "My Message"
// -- or()
mayFail(true).or(100); // returns 123
mayFail(false).or(100); // returns 100
// -- else()
mayFail(true).else((error) => 200); // returns 123
mayFail(false).else((error) => 200); // returns 100 (error can be used for some extra logic)
More elaborate examples:
Result<T, E>
and throw()
for handling JSON parsetype DataType = {
a: number;
b: number;
};
function parse(data: string): Result<DataType, Error> {
let result: DataType;
try {
result = JSON.parse(data);
} catch (err) {
throw new Error("Couldn't parse JSON");
}
return Ok(result);
}
const data: DataType = parse('{"a":100,"b":200}').throw(); // Returns {a: 100, b: 200}
const data: DataType = parse('"a":100,"b":200').throw(); // Throws Error("Couldn't parse JSON")
throw()
to unwrap the value after parsing a numberfunction toNumber(str: string): Result<number, string> {
const result = Number(str);
if (isNaN(result)) {
return Err("Couldn't parse a string");
}
return Ok(result);
}
const myNumber: number = toNumber("Hello").throw(); // Throws an Error
const myNumber: number = toNumber("123").throw(); // Returns 123
or()
to provide a back-up value while obtaining status codefunction getStatusCode(statusCode: number): Result<number, string> {
if (statusCode > 200 && statusCode < 300) {
return Ok(statusCode);
}
return Err("Invalide status code");
}
function obtainStatus(): number {
return getStatusCode(response.statusCode).or(404); // Returns `statusCode` between 201 and 299 or 404
}
else()
and CustomError
to provide a back-up value based on error typeenum ErrorType {
A,
B,
C,
}
class CustomError extends Error {
private errorType: ErrorType;
constructor(type: ErrorType) {
super();
this.errorType = type;
}
get type(): ErrorType {
return this.errorType;
}
}
function myFunction(): Result<void, CustomError> {
//...
}
myFunction().else((error) => {
switch (error.type) {
case ErrorType.A:
return "a";
case ErrorType.B:
return "b";
case ErrorType.C:
return "c";
default:
break;
}
});
FAQs
TypeScript Result
We found that ts-res demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.