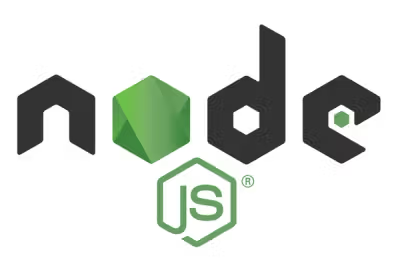
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
tsch-ej-numbers
Advanced tools
API for analyzing EuroJackpot lottery numbers, trends, and probabilities.
This is an API to analyze the EuroJackpot draws.
https://en.wikipedia.org/wiki/Eurojackpot
npm install --save tsch-ej-numbers
export type TRecord = {
date: string;
wn1: number;
wn2: number;
wn3: number;
wn4: number;
wn5: number;
en1: number;
en2: number;
stake: number;
countCl1: number;
quotaCl1: number;
countCl2: number;
quotaCl2: number;
countCl3: number;
quotaCl3: number;
countCl4: number;
quotaCl4: number;
countCl5: number;
quotaCl5: number;
countCl6: number;
quotaCl6: number;
countCl7: number;
quotaCl7: number;
countCl8: number;
quotaCl8: number;
countCl9: number;
quotaCl9: number;
countCl10: number;
quotaCl10: number;
countCl11: number;
quotaCl11: number;
countCl12: number;
quotaCl12: number;
day: string;
};
Function | Version |
---|---|
getRecords | 1.0.x |
getLastDraw | 1.0.x |
getFirstDraw | 1.0.x |
getClassOneDraws | 1.0.x |
getMaxJackpotDraws | 1.0.x |
getWinningNumbersCount | 1.0.x |
getEuroNumbersCount | 1.0.x |
getDecadesCount | 1.0.x |
getLowHighCount | 1.0.x |
getMinMaxQuotaCount | 1.1.x |
Returns all records of available draws.
Code:
import { getRecords } from "tsch-ej-numbers";
console.log(getRecords()); // all records
console.log(getRecords(0)); // all records
console.log(getRecords(10)); // last 10 records
Returns the last current record.
Code:
import { getLastDraw } from "tsch-ej-numbers";
console.log(getLastDraw());
Returns the first record.
Code:
import { getFirstDraw } from "tsch-ej-numbers";
console.log(getFirstDraw());
Returns all records with success in class one.
Code:
import { getClassOneDraws } from "tsch-ej-numbers";
console.log(getClassOneDraws(100));
Returns all records with success in class one and a quota of 120.000.000,00 €.
Code:
import { getMaxJackpotDraws } from "tsch-ej-numbers";
console.log(getMaxJackpotDraws());
console.log(getMaxJackpotDraws(0));
console.log(getMaxJackpotDraws(100));
Returns a key / value array for all winning numbers.
Code:
import { getWinningNumbersCount } from "tsch-ej-numbers";
console.log(getWinningNumbersCount());
console.log(getWinningNumbersCount(0));
console.log(getWinningNumbersCount(100));
Returns a key / value array for all euro numbers.
Code:
import { getEuroNumbersCount } from "tsch-ej-numbers";
console.log(getEuroNumbersCount());
console.log(getEuroNumbersCount(0));
console.log(getEuroNumbersCount(100));
Returns an object for decades with according count.
Code:
import { getDecadesCount } from "tsch-ej-numbers";
console.log(getDecadesCount());
console.log(getDecadesCount(0));
console.log(getDecadesCount(100));
Returns an object for low and high sections with according count.
Code:
import { getLowHighCount } from "tsch-ej-numbers";
console.log(getLowHighCount());
console.log(getLowHighCount(0));
console.log(getLowHighCount(100));
Returns an object for all winning classes (1...12) with all corresponding sub-objects (min/max). These sub-objects return the date and the corresponding value.
Code:
import { getMinMaxQuotaCount } from "tsch-ej-numbers";
console.log(getMinMaxQuotaCount());
console.log(getMinMaxQuotaCount(0));
console.log(getMinMaxQuotaCount(100));
FAQs
API for analyzing EuroJackpot lottery numbers, trends, and probabilities.
The npm package tsch-ej-numbers receives a total of 36 weekly downloads. As such, tsch-ej-numbers popularity was classified as not popular.
We found that tsch-ej-numbers demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.