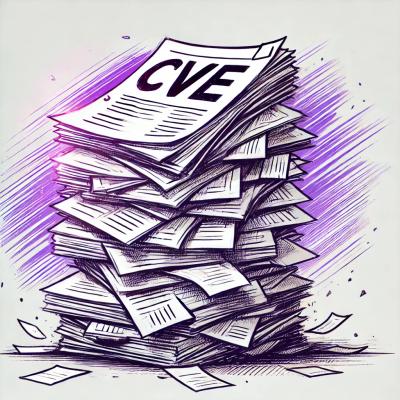
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
tslint-eslint-rules
Advanced tools
You want to code in TypeScript but miss all the rules available in ESLint?
Now you can combine both worlds by using this TSLint plugin!
WARN: this project is still under development.
You can see what rules were already migrated in the eslint_tslint.json file
npm install --save-dev tslint-eslint-rules
tslint-eslint-rules
folder:tslint [file] -r node_modules/tslint-eslint-rules/dist/rules
gulp-tslint
)gulp
.src(/* [files] */)
.pipe(tslint({
rulesDirectory: 'node_modules/tslint-eslint-rules/dist/rules'
}));
grunt-tslint
)grunt.initConfig({
/* other configurations */
tslint: {
options: {
configuration: grunt.file.readJSON('tslint.json'),
rulesDirectory: 'node_modules/tslint-eslint-rules/dist/rules'
},
files: {
src: [/* [files] */]
}
}
})
Open File > Settings
and follow the example below:
linter-tslint
)It's not possible to use custom rules using the linter-tslint
plugin at this moment.
We already created an issue for them, but unfortunately, the codebase is in CoffeeScript, and we can't help with a pull request.
SublimeLinter-contrib-tslint
)In your Packages/User/SublimeLinter.sublime-settings
file, you can configure tslint
options, similar to the example bellow:
{
"user": {
"linters": {
"tslint": {
"args": [
"--r=node_modules/tslint-eslint-rules/dist/rules"
]
}
}
}
}
Then, in your tslint.json
file, insert the rules as described below.
The list below shows all the existing ESLint rules and the similar rules available in TSLint.
The following rules point out areas where you might have made mistakes.
comma-dangle => trailing-comma (native)
Description: disallow or enforce trailing commas (recommended)
Usage
"trailing-comma": [
true,
{
"multiline": "always",
"singleline": "never"
}
]
no-cond-assign => no-conditional-assignment (native)
Description: disallow assignment in conditional expressions (recommended)
Usage
"no-conditional-assignment": true
no-console => no-console (native)
Description: disallow use of console
in the node environment (recommended)
Usage
"no-console": [
true,
"debug",
"info",
"time",
"timeEnd",
"trace"
]
no-constant-condition => no-constant-condition (tslint-eslint-rules)
Description: disallow use of constant expressions in conditions (recommended)
Usage
"no-constant-condition": true
no-control-regex => no-control-regex (tslint-eslint-rules) TODO
Description: disallow control characters in regular expressions (recommended)
Usage
"no-control-regex": true
no-debugger => no-debugger (native)
Description: disallow use of debugger
(recommended)
Usage
"no-debugger": true
no-dupe-args => not applicable to TypeScript
no-dupe-keys => no-duplicate-key (native)
Description: disallow duplicate keys when creating object literals (recommended)
Usage
"no-duplicate-key": true
no-duplicate-case => no-duplicate-case (tslint-eslint-rules)
Description: disallow a duplicate case label. (recommended)
Usage
"no-duplicate-case": true
no-empty-character-class => no-empty-character-class (tslint-eslint-rules) TODO
Description: disallow the use of empty character classes in regular expressions (recommended)
Usage
"no-empty-character-class": true
no-empty => no-empty (native)
Description: disallow empty statements (recommended)
Usage
"no-empty": true
no-ex-assign => no-ex-assign (tslint-eslint-rules) TODO
Description: disallow assigning to the exception in a catch
block (recommended)
Usage
"no-ex-assign": true
no-extra-boolean-cast => no-extra-boolean-cast (tslint-eslint-rules)
Description: disallow double-negation boolean casts in a boolean context (recommended)
Usage
"no-extra-boolean-cast": true
no-extra-parens => no-extra-parens (tslint-eslint-rules) TODO (low priority)
Description: disallow unnecessary parentheses
Usage
"no-extra-parens": [
true,
"functions"
]
"no-extra-parens": [
true,
"all"
]
no-extra-semi => no-extra-semi (tslint-eslint-rules)
Description: disallow unnecessary semicolons (recommended)
Usage
"no-extra-semi": true
no-func-assign => not applicable to TypeScript
no-inner-declarations => no-inner-declarations (tslint-eslint-rules) TODO
Description: disallow function or variable declarations in nested blocks (recommended)
Usage
"no-inner-declarations": [
true,
"functions"
]
"no-inner-declarations": [
true,
"both"
]
no-invalid-regexp => no-invalid-regex (tslint-eslint-rules) TODO
Description: disallow invalid regular expression strings in the RegExp
constructor (recommended)
Usage
"no-invalid-regexp": true
no-irregular-whitespace => no-irregular-whitespace (tslint-eslint-rules) TODO
Description: disallow irregular whitespace outside of strings and comments (recommended)
Usage
"no-irregular-whitespace": true
no-negated-in-lhs => not applicable to TypeScript
in
expression (recommended)no-obj-calls => not applicable to TypeScript
Math
and JSON
) as functions (recommended)no-regex-spaces => no-regex-spaces (tslint-eslint-rules) TODO
Description: disallow multiple spaces in a regular expression literal (recommended)
Usage
"no-regex-spaces": true
no-sparse-arrays => no-sparse-arrays (tslint-eslint-rules)
Description: disallow sparse arrays (recommended)
Usage
"no-sparse-arrays": true
no-unexpected-multiline => no-unexpected-multiline (tslint-eslint-rules) TODO
Description: Avoid code that looks like two expressions but is actually one
Usage
"no-unexpected-multiline": true
no-unreachable => no-unreachable (native)
Description: disallow unreachable statements after a return, throw, continue, or break statement (recommended)
Usage
"no-unreachable": true
use-isnan => use-isnan (tslint-eslint-rules)
Description: disallow comparisons with the value NaN
(recommended)
Usage
"use-isnan": true
valid-jsdoc => valid-jsdoc (tslint-eslint-rules) TODO
Description: Ensure JSDoc comments are valid
Usage
"valid-jsdoc": [
true,
{
"prefer": {
"return": "returns"
},
"requireReturn": false,
"requireParamDescription": true,
"requireReturnDescription": true,
"matchDescription": "^[A-Z][A-Za-z0-9\\s]*[.]$"
}
]
valid-typeof => valid-typeof (tslint-eslint-rules)
Description: Ensure that the results of typeof are compared against a valid string (recommended)
Usage
"valid-typeof": true
These are rules designed to prevent you from making mistakes. They either prescribe a better way of doing something or help you avoid footguns.
Description: Enforces getter/setter pairs in objects
Usage
"accessor-pairs": [
true,
{
}
]
Description: treat var
statements as if they were block scoped
Usage
"block-scoped-var": [
true,
{
}
]
Description: specify the maximum cyclomatic complexity allowed in a program
Usage
"complexity": [
true,
{
}
]
Description: require return
statements to either always or never specify values
Usage
"consistent-return": [
true,
{
}
]
Description: specify curly brace conventions for all control statements
Usage
"curly": [
true,
{
}
]
Description: require default
case in switch
statements
Usage
"default-case": [
true,
{
}
]
Description: enforces consistent newlines before or after dots
Usage
"dot-location": [
true,
{
}
]
Description: encourages use of dot notation whenever possible
Usage
"dot-notation": [
true,
{
}
]
Description: require the use of ===
and !==
Usage
"eqeqeq": [
true,
{
}
]
Description: make sure for-in
loops have an if
statement
Usage
"guard-for-in": [
true,
{
}
]
Description: disallow the use of alert
, confirm
, and prompt
Usage
"no-alert": [
true,
{
}
]
Description: disallow use of arguments.caller
or arguments.callee
Usage
"no-caller": [
true,
{
}
]
Description: disallow lexical declarations in case clauses
Usage
"no-case-declarations": [
true,
{
}
]
Description: disallow division operators explicitly at beginning of regular expression
Usage
"no-div-regex": [
true,
{
}
]
Description: disallow else
after a return
in an if
Usage
"no-else-return": [
true,
{
}
]
Description: disallow use of labels for anything other than loops and switches
Usage
"no-empty-label": [
true,
{
}
]
Description: disallow use of empty destructuring patterns
Usage
"no-empty-pattern": [
true,
{
}
]
Description: disallow comparisons to null without a type-checking operator
Usage
"no-eq-null": [
true,
{
}
]
Description: disallow use of eval()
Usage
"no-eval": [
true,
{
}
]
Description: disallow adding to native types
Usage
"no-extend-native": [
true,
{
}
]
Description: disallow unnecessary function binding
Usage
"no-extra-bind": [
true,
{
}
]
Description: disallow fallthrough of case
statements (recommended)
Usage
"no-fallthrough": [
true,
{
}
]
Description: disallow the use of leading or trailing decimal points in numeric literals
Usage
"no-floating-decimal": [
true,
{
}
]
Description: disallow the type conversions with shorter notations
Usage
"no-implicit-coercion": [
true,
{
}
]
Description: disallow use of eval()
-like methods
Usage
"no-implied-eval": [
true,
{
}
]
Description: disallow this
keywords outside of classes or class-like objects
Usage
"no-invalid-this": [
true,
{
}
]
Description: disallow Usage
of __iterator__
property
Usage
"no-iterator": [
true,
{
}
]
Description: disallow use of labeled statements
Usage
"no-labels": [
true,
{
}
]
Description: disallow unnecessary nested blocks
Usage
"no-lone-blocks": [
true,
{
}
]
Description: disallow creation of functions within loops
Usage
"no-loop-func": [
true,
{
}
]
Description: disallow the use of magic numbers
Usage
"no-magic-numbers": [
true,
{
}
]
Description: disallow use of multiple spaces
Usage
"no-multi-spaces": [
true,
{
}
]
Description: disallow use of multiline strings
Usage
"no-multi-str": [
true,
{
}
]
Description: disallow reassignments of native objects
Usage
"no-native-reassign": [
true,
{
}
]
Description: disallow use of new operator for Function
object
Usage
"no-new-func": [
true,
{
}
]
Description: disallows creating new instances of String
,Number
, and Boolean
Usage
"no-new-wrappers": [
true,
{
}
]
Description: disallow use of the new
operator when not part of an assignment or comparison
Usage
"no-new": [
true,
{
}
]
Description: disallow use of octal escape sequences in string literals, such as var foo = "Copyright \251";
Usage
"no-octal-escape": [
true,
{
}
]
Description: disallow use of octal literals (recommended)
Usage
"no-octal": [
true,
{
}
]
Description: disallow reassignment of function parameters
Usage
"no-param-reassign": [
true,
{
}
]
Description: disallow use of process.env
Usage
"no-process-env": [
true,
{
}
]
Description: disallow Usage
of __proto__
property
Usage
"no-proto": [
true,
{
}
]
Description: disallow declaring the same variable more than once (http://eslint.org/docs/rules/recommended)
Usage
"no-redeclare": [
true,
{
}
]
Description: disallow use of assignment in return
statement
Usage
"no-return-assign": [
true,
{
}
]
Description: disallow use of javascript:
urls.
Usage
"no-script-url": [
true,
{
}
]
Description: disallow comparisons where both sides are exactly the same
Usage
"no-self-compare": [
true,
{
}
]
Description: disallow use of the comma operator
Usage
"no-sequences": [
true,
{
}
]
Description: restrict what can be thrown as an exception
Usage
"no-throw-literal": [
true,
{
}
]
Description: disallow Usage of expressions in statement position
Usage
"no-unused-expressions": [
true,
{
}
]
Description: disallow unnecessary .call()
and .apply()
Usage
"no-useless-call": [
true,
{
}
]
Description: disallow unnecessary concatenation of literals or template literals
Usage
"no-useless-concat": [
true,
{
}
]
Description: disallow use of the void
operator
Usage
"no-void": [
true,
{
}
]
Description: e.g. TODO
or FIXME
Usage
"no-warning-comments": [
true,
{
}
]
Description: disallow use of the with
statement
Usage
"no-with": [
true,
{
}
]
Description: require use of the second argument for parseInt()
Usage
"radix": [
true,
{
}
]
Description: require declaration of all vars at the top of their containing scope
Usage
"vars-on-top": [
true,
{
}
]
Description: require immediate function invocation to be wrapped in parentheses
Usage
"wrap-iife": [
true,
{
}
]
Description: require or disallow Yoda conditions
Usage
"yoda": [
true,
{
}
]
These rules relate to using strict mode.
Description: controls location of Use Strict Directives
Usage
"strict": [
true,
{
}
]
These rules have to do with variable declarations.
Description: enforce or disallow variable initializations at definition
Usage
"init-declarations": [
true,
{
}
]
Description: disallow the catch clause parameter name being the same as a variable in the outer scope
Usage
"no-catch-shadow": [
true,
{
}
]
Description: disallow deletion of variables (recommended)
Usage
"no-delete-var": [
true,
{
}
]
Description: disallow labels that share a name with a variable
Usage
"no-label-var": [
true,
{
}
]
Description: disallow shadowing of names such as arguments
Usage
"no-shadow-restricted-names": [
true,
{
}
]
Description: disallow declaration of variables already declared in the outer scope
Usage
"no-shadow": [
true,
{
}
]
Description: disallow use of undefined when initializing variables
Usage
"no-undef-init": [
true,
{
}
]
Description: disallow use of undeclared variables unless mentioned in a /*global */
block (recommended)
Usage
"no-undef": [
true,
{
}
]
Description: disallow use of undefined
variable
Usage
"no-undefined": [
true,
{
}
]
Description: disallow declaration of variables that are not used in the code (recommended)
Usage
"no-unused-vars": [
true,
{
}
]
Description: disallow use of variables before they are defined
Usage
"no-use-before-define": [
true,
{
}
]
These rules are specific to JavaScript running on Node.js or using CommonJS in the browser.
Description: enforce return
after a callback
Usage
"callback-return": [
true,
{
}
]
Description: enforce require()
on top-level module scope
Usage
"global-require": [
true,
{
}
]
Description: enforce error handling in callbacks
Usage
"handle-callback-err": [
true,
{
}
]
Description: disallow mixing regular variable and require declarations
Usage
"no-mixed-requires": [
true,
{
}
]
Description: disallow use of new
operator with the require
function
Usage
"no-new-require": [
true,
{
}
]
Description: disallow string concatenation with __dirname
and __filename
Usage
"no-path-concat": [
true,
{
}
]
Description: disallow process.exit()
Usage
"no-process-exit": [
true,
{
}
]
Description: restrict Usage of specified node modules
Usage
"no-restricted-modules": [
true,
{
}
]
Description: disallow use of synchronous methods
Usage
"no-sync": [
true,
{
}
]
These rules are purely matters of style and are quite subjective.
Description: enforce spacing inside array brackets
Usage
"array-bracket-spacing": [
true,
{
}
]
Description: disallow or enforce spaces inside of single line blocks
Usage
"block-spacing": [
true,
{
}
]
Description: enforce one true brace style
Usage
"brace-style": [
true,
{
}
]
Description: require camel case names
Usage
"camelcase": [
true,
{
}
]
Description: enforce spacing before and after comma
Usage
"comma-spacing": [
true,
{
}
]
Description: enforce one true comma style
Usage
"comma-style": [
true,
{
}
]
Description: require or disallow padding inside computed properties
Usage
"computed-property-spacing": [
true,
{
}
]
Description: enforce consistent naming when capturing the current execution context
Usage
"consistent-this": [
true,
{
}
]
Description: enforce newline at the end of file, with no multiple empty lines
Usage
"eol-last": [
true,
{
}
]
Description: require function expressions to have a name
Usage
"func-names": [
true,
{
}
]
Description: enforce use of function declarations or expressions
Usage
"func-style": [
true,
{
}
]
Description: this option enforces minimum and maximum identifier lengths (variable names, property names etc.)
Usage
"id-length": [
true,
{
}
]
Description: require identifiers to match the provided regular expression
Usage
"id-match": [
true,
{
}
]
Description: specify tab or space width for your code
Usage
"indent": [
true,
{
}
]
Description: specify whether double or single quotes should be used in JSX attributes
Usage
"jsx-quotes": [
true,
{
}
]
Description: enforce spacing between keys and values in object literal properties
Usage
"key-spacing": [
true,
{
}
]
Description: disallow mixed 'LF' and 'CRLF' as linebreaks
Usage
"linebreak-style": [
true,
{
}
]
Description: enforce empty lines around comments
Usage
"lines-around-comment": [
true,
{
}
]
Description: specify the maximum depth callbacks can be nested
Usage
"max-nested-callbacks": [
true,
{
}
]
Description: require a capital letter for constructors
Usage
"new-cap": [
true,
{
}
]
Description: disallow the omission of parentheses when invoking a constructor with no arguments
Usage
"new-parens": [
true,
{
}
]
Description: require or disallow an empty newline after variable declarations
Usage
"newline-after-var": [
true,
{
}
]
Description: disallow use of the Array
constructor
Usage
"no-array-constructor": [
true,
{
}
]
Description: disallow use of the continue
statement
Usage
"no-continue": [
true,
{
}
]
Description: disallow comments inline after code
Usage
"no-inline-comments": [
true,
{
}
]
Description: disallow if
as the only statement in an else
block
Usage
"no-lonely-if": [
true,
{
}
]
Description: disallow mixed spaces and tabs for indentation (recommended)
Usage
"no-mixed-spaces-and-tabs": [
true,
{
}
]
Description: disallow multiple empty lines
Usage
"no-multiple-empty-lines": [
true,
{
}
]
Description: disallow negated conditions
Usage
"no-negated-condition": [
true,
{
}
]
Description: disallow nested ternary expressions
Usage
"no-nested-ternary": [
true,
{
}
]
Description: disallow the use of the Object
constructor
Usage
"no-new-object": [
true,
{
}
]
Description: disallow use of certain syntax in code
Usage
"no-restricted-syntax": [
true,
{
}
]
Description: disallow space between function identifier and application
Usage
"no-spaced-func": [
true,
{
}
]
Description: disallow the use of ternary operators
Usage
"no-ternary": [
true,
{
}
]
Description: disallow trailing whitespace at the end of lines
Usage
"no-trailing-spaces": [
true,
{
}
]
Description: disallow dangling underscores in identifiers
Usage
"no-underscore-dangle": [
true,
{
}
]
Description: disallow the use of ternary operators when a simpler alternative exists
Usage
"no-unneeded-ternary": [
true,
{
}
]
Description: require or disallow padding inside curly braces
Usage
"object-curly-spacing": [
true,
{
}
]
Description: require or disallow one variable declaration per function
Usage
"one-var": [
true,
{
}
]
Description: require assignment operator shorthand where possible or prohibit it entirely
Usage
"operator-assignment": [
true,
{
}
]
Description: enforce operators to be placed before or after line breaks
Usage
"operator-linebreak": [
true,
{
}
]
Description: enforce padding within blocks
Usage
"padded-blocks": [
true,
{
}
]
Description: require quotes around object literal property names
Usage
"quote-props": [
true,
{
}
]
Description: specify whether backticks, double or single quotes should be used
Usage
"quotes": [
true,
{
}
]
Description: Require JSDoc comment
Usage
"require-jsdoc": [
true,
{
}
]
Description: enforce spacing before and after semicolons
Usage
"semi-spacing": [
true,
{
}
]
Description: require or disallow use of semicolons instead of ASI
Usage
"semi": [
true,
{
}
]
Description: sort variables within the same declaration block
Usage
"sort-vars": [
true,
{
}
]
Description: require a space after certain keywords
Usage
"space-after-keywords": [
true,
{
}
]
Description: require or disallow a space before blocks
Usage
"space-before-blocks": [
true,
{
}
]
Description: require or disallow a space before function opening parenthesis
Usage
"space-before-function-paren": [
true,
{
}
]
Description: require a space before certain keywords
Usage
"space-before-keywords": [
true,
{
}
]
Description: require or disallow spaces inside parentheses
Usage
"space-in-parens": [
true,
{
}
]
Description: require spaces around operators
Usage
"space-infix-ops": [
true,
{
}
]
Description: require a space after return
, throw
, and case
Usage
"space-return-throw-case": [
true,
{
}
]
Description: require or disallow spaces before/after unary operators
Usage
"space-unary-ops": [
true,
{
}
]
Description: require or disallow a space immediately following the //
or /*
in a comment
Usage
"spaced-comment": [
true,
{
}
]
Description: require regex literals to be wrapped in parentheses
Usage
"wrap-regex": [
true,
{
}
]
These rules are only relevant to ES6 environments.
Description: require braces in arrow function body
Usage
"arrow-body-style": [
true,
{
}
]
Description: require parens in arrow function arguments
Usage
"arrow-parens": [
true,
{
}
]
Description: require space before/after arrow function's arrow
Usage
"arrow-spacing": [
true,
{
}
]
Description: verify calls of super()
in constructors
Usage
"constructor-super": [
true,
{
}
]
Description: enforce spacing around the *
in generator functions
Usage
"generator-star-spacing": [
true,
{
}
]
Description: disallow arrow functions where a condition is expected
Usage
"no-arrow-condition": [
true,
{
}
]
Description: disallow modifying variables of class declarations
Usage
"no-class-assign": [
true,
{
}
]
Description: disallow modifying variables that are declared using const
Usage
"no-const-assign": [
true,
{
}
]
Description: disallow duplicate name in class members
Usage
"no-dupe-class-members": [
true,
{
}
]
Description: disallow use of this
/super
before calling super()
in constructors.
Usage
"no-this-before-super": [
true,
{
}
]
Description: require let
or const
instead of var
Usage
"no-var": [
true,
{
}
]
Description: require method and property shorthand syntax for object literals
Usage
"object-shorthand": [
true,
{
}
]
Description: suggest using arrow functions as callbacks
Usage
"prefer-arrow-callback": [
true,
{
}
]
Description: suggest using const
declaration for variables that are never modified after declared
Usage
"prefer-const": [
true,
{
}
]
Description: suggest using Reflect methods where applicable
Usage
"prefer-reflect": [
true,
{
}
]
Description: suggest using the spread operator instead of .apply()
.
Usage
"prefer-spread": [
true,
{
}
]
Description: suggest using template literals instead of strings concatenation
Usage
"prefer-template": [
true,
{
}
]
Description: disallow generator functions that do not have yield
Usage
"require-yield": [
true,
{
}
]
The following rules are included for compatibility with JSHint and JSLint. While the names of the rules may not match up with the JSHint/JSLint counterpart, the functionality is the same.
Description: specify the maximum depth that blocks can be nested
Usage
"max-depth": [
true,
{
}
]
Description: specify the maximum length of a line in your program
Usage
"max-len": [
true,
{
}
]
Description: limits the number of parameters that can be used in the function declaration.
Usage
"max-params": [
true,
{
}
]
Description: specify the maximum number of statement allowed in a function
Usage
"max-statements": [
true,
{
}
]
Description: disallow use of bitwise operators
Usage
"no-bitwise": [
true,
{
}
]
Description: disallow use of unary operators, ++
and --
Usage
"no-plusplus": [
true,
{
}
]
Bugs, rules requests, doubts etc., open a Github Issue.
If you didn't find the rule, you can also create an ESLint custom rule for TSLint:
npm isntall
gulp
to run the tests and watch for file changes./src/test/rules
and your rule in ./src/rules
with the convetion:
Rule
suffix)RuleTests
suffix)MIT
FAQs
Improve your TSLint with the missing ESLint Rules
The npm package tslint-eslint-rules receives a total of 116,101 weekly downloads. As such, tslint-eslint-rules popularity was classified as popular.
We found that tslint-eslint-rules demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.