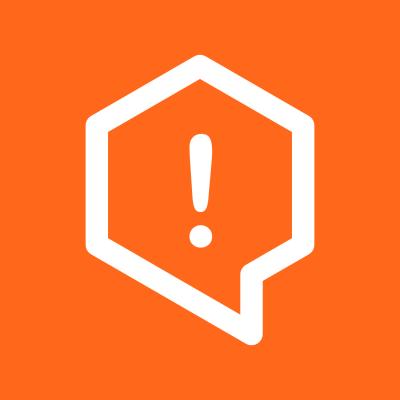
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
tspace-ez-node
Advanced tools
tspace-ez-node is an api framework for node.js highly focused on providing the best developer experience.
Install with npm:
npm install tspace-ez-node --save
import { Application , Router, TContext, TNextFunction } from "tspace-ez-node";
const app = new Application()
app.get('/' , ({ res } : TContext) => {
return res.json({
message : 'hello world!'
});
})
const server = app.createServer()
let port = 3000
server.listen(port , () => {
console.log(`Server is now listening port: ${port}`);
})
server.on('error', (error: NodeJS.ErrnoException) => {
port = Math.floor(Math.random() * 8999) + 1000
server.listen(port)
});
// localhost:3000
// file cat-middleware.ts
export default (ctx : TContext, next: TNextFunction) =>{
console.log('cat middleware globals');
return next();
}
import { Application , Router, TContext, TNextFunction } from "tspace-ez-node";
import CatMiddleware from './cat-middleware.ts'
(async () => {
const app = new Application({
middlewares: [ CatMiddleware ]
// if you want to import a middleware with a directory can you follow the example
middlewares : {
folder : `${__dirname}/middlewares`,
name : /middleware\.(ts|js)$/i
}
})
// or add a middleware
app.use((ctx : TContext , next : TNextFunction) => {
console.log('global middlewares')
return next()
})
app.get('/' , ({ res } : TContext) => {
return res.json({
message : 'hello world!'
});
})
const server = await app.createServerSync()
let port = 3000
server.listen(port , () => {
console.log(`Server is now listening port: ${port}`);
})
server.on('error', (error: NodeJS.ErrnoException) => {
port = Math.floor(Math.random() * 8999) + 1000
server.listen(port)
});
// localhost:3000
})()
import {
Controller ,
Middleware ,
Get ,
Post,
PATCH,
PUT,
DELETE,
WriteHeader ,
Query,
Body,
Params,
Cookies,
Files,
StatusCode
} from 'tspace-ez-node';
import type {
TCookies, TParams,
TRequest, TResponse ,
TQuery, TFiles,
TContext, TNextFunction
} from 'tspace-ez-node';
import CatMiddleware from './cat-middleware.ts'
// file cat-controller.ts
@Controller('/cats')
class CatController {
@Get('/')
@Middleware(CatMiddleware)
@Query('test','id')
@Cookies('name')
public async index({ query , cookies , req } : {
query : TQuery<{ id : string }>
cookies : TCookies<{ name : string}>
req : TRequest
}) {
return {
message : 'index',
query,
cookies
}
}
@Get('/:id')
@Middleware(CatMiddleware)
@Params('id')
public async indexz({ params} : TContext) {
const id = params.id
// find something
return {
params
}
}
}
import { Application , Router, TContext, TNextFunction } from "tspace-ez-node";
import CatController from './cat-controller.ts'
(async () => {
const app = new Application({
controllers: [ CatController ]
// if you want to import a controller with a directory can you follow the example
controllers : {
folder : `${__dirname}/controllers`,
name : /middleware\.(ts|js)$/i
}
})
app.get('/' , ({ res } : TContext) => {
return res.json({
message : 'hello world!'
});
})
const server = await app.createServerSync()
let port = 3000
server.listen(port , () => {
console.log(`Server is now listening port: ${port}`);
})
server.on('error', (error: NodeJS.ErrnoException) => {
port = Math.floor(Math.random() * 8999) + 1000
server.listen(port)
});
// localhost:3000/cats
// localhost:3000/cats/41
})()
import { Application , Router, TContext, TNextFunction } from "tspace-ez-node";
const app = new Application()
const router = new Router()
router.groups('/my',(r) => {
r.get('/cats' , ({ req , res }) => {
return res.json({
message : 'Hello, World!'
})
})
return r
})
router.get('/cats' , ({ req , res }) => {
return res.json({
message : 'Hello, World!'
})
})
app.useRouter(router)
app.get('/' , ({ res } : TContext) => {
return res.json({
message : 'hello world!'
});
})
const server = app.createServer()
let port = 3000
server.listen(port , () => {
console.log(`Server is now listening port: ${port}`);
})
server.on('error', (error: NodeJS.ErrnoException) => {
port = Math.floor(Math.random() * 8999) + 1000
server.listen(port)
});
// localhost:3000/my/cats
// localhost:3000/cats
const app = new Application({
logger : true, // logging
prefixRoute : '/api' // prefix all routes in Router and Controller only!
})
app.get('/' , ({ res } : TContext) => {
return res.json({
message : 'hello world!'
});
})
app.get('/errors', () => {
throw new Error('testing Error handler')
})
// every response should returns following this format response
app.formatResponseHandler((results : any , statusCode : number) => {
return {
success : statusCode < 400,
...results,
code : statusCode
}
})
// every notfound page should returns following this format response
app.notFoundHandler(({ res } : TContext) => {
return res.notFound();
})
// every errors page should returns following this format response
app.errorHandler((err : any , ctx : TContext) => {
ctx.res.status(500)
return ctx.res.json({
success : false,
message : err?.message,
code : 500
});
})
const server = app.createServer()
let port = 3000
server.listen(port , () => {
console.log(`Server is now listening port: ${port}`);
})
server.on('error', (error: NodeJS.ErrnoException) => {
port = Math.floor(Math.random() * 8999) + 1000
server.listen(port)
});
// localhost:3000/*********** // not found
// localhost:3000/errors // errors
// localhost:3000 // format response
FAQs
Fast http build an API, for Node.js
The npm package tspace-ez-node receives a total of 0 weekly downloads. As such, tspace-ez-node popularity was classified as not popular.
We found that tspace-ez-node demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.