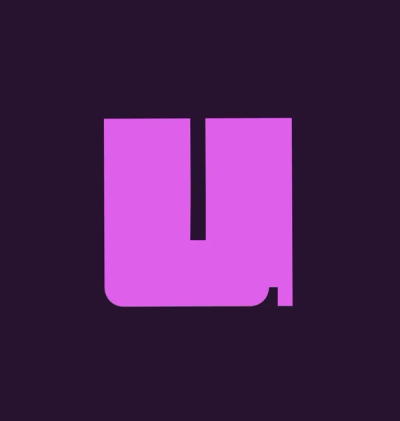
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
tt-ide-cli
Advanced tools
tma
是字节跳动小程序官方提供的命令行工具
建议在全局安装 tma
npm install -g tt-ide-cli
主要用于配置工具的全局代理
Usage: tma set-config [options]
Set tt-ide-cli config
Options:
--proxy <proxy> Set global proxy(配置全局代理)
--default Use default(恢复为默认配置)
const tma = require('tt-ide-cli');
await tma.setConfig({
proxy: 'http://127.0.0.1:8899';
});
在当前目录下,以给定的项目名字创建一个空白的小程序项目
Usage: tma create [options] <project-name>
Create a new project with given name in current folder
Options:
-f, --force Overwrite target directory if it exists
--template <template> rich | empty (default: empty)
--type <type>', 'js | ts (default: js)
--targetDir <targetDir> Target directory
const tma = require('tt-ide-cli');
await tma.create({
projectName: 'projectName',
force: false, // 是否覆盖目标文件夹
template: 'rich', // 'rich' | 'empty'
// rich 为小程序能力展示 DEMO
// empty 为小程序空项目
targetDir: '/path/to/targetDir',
type: 'js', // 'js' | 'ts'
// js 为 js 小程序
// ts 为 ts 小程序
});
在小程序开发者工具中打开给定目录的项目,如果给定的目录不存在,则仅打开开发者工具
Usage: tma open <project-path>
Open target project by path
const tma = require('tt-ide-cli');
await tma.open({
project: {
path: 'projectPath',
},
});
登录到开发者平台
Usage: tma login [options]
Login to the developer platform
Options:
-m, --mobile Login by mobile
-e, --email Login by email
-p, --proxy <proxy> Login with proxy
-h, --help Output usage information
Usage: tma login-e [email] [password]
Login to the developer platform by E-mail
Options:
-p, --proxy <proxy> Login with proxy
-h, --help Output usage information
const tma = require('tt-ide-cli');
await tma.loginByEmail({
email: 'email',
password: 'password',
});
Usage: tma logout
Logout and clear the session.
const tma = require('tt-ide-cli');
await tma.logout();
查看本地项目的文件体积
Usage: tma project-size [--json] entry
Output current project package size information.
Options:
--json Output as JSON string
暂不支持代码调用
将项目上传后,扫码二维码来预览小程序。
Usage: tma preview [options] [entry]
Preview project by remote
Options:
--disable-cache Preview project without local cache
-s, --small Use small QR Code, it may fail in some environments
-c, --copy Copy remote url to clipboard
-p, --proxy <proxy> Preview with proxy
--miniapp-path <path> Miniapp path
--miniapp-query <query> Miniapp query
--miniapp-scene <scene> Miniapp scene
--miniapp-launch-from <launchFrom> Miniapp launchFrom
--miniapp-location <location> Miniapp location
--qrcode-output <qrcodeOutputPath> Qrcode output path
const tma = require('tt-ide-cli');
// previewResult 返回值
interface ProjectQRCode {
expireTime: number; // 二维码过期时间
shortUrl: string; // 二维码短链
originSchema: string; // 二维码 schema
qrcodeSVG?: string; // 二维码 SVG
qrcodeFilePath?: string; // 二维码存储路径
useCache: boolean; // 是否命中并使用缓存
}
const previewResult: ProjectQRCode = await tma.preview({
project: {
path: 'projectPath', // 项目地址
},
page: {
path: '', // 小程序打开页面
query: '', // 小程序打开 query
scene: '', // 小程序打开场景值
launchFrom: '', // 小程序打开场景(未知可填空字符串)
location: '', // 小程序打开位置(未知可填空字符串)
},
qrcode: {
format: 'imageSVG', // imageSVG | imageFile | null | terminal
// imageSVG 用于产出二维码 SVG
// imageFile 用于将二维码存储到某个路径
// terminal 用于将二维码在控制台输出
// null 则不产出二维码
output: '', // 只在 imageFile 生效,填写图片输出绝对路径
options: {
small: false, // 使用小二维码,主要用于 terminal
},
},
cache: true, // 是否使用缓存
copyToClipboard: true, // 是否将产出的二维码链接复制到剪切板
});
把项目上传到开发者平台进行发布
Usage: tma upload [options] [entry]
Upload project to the developer platform
Options:
-v, --app-version <appVersion> App version (eg: [major].[minor].[patch])
-c, --app-changelog <appChangelog> Changelog for this version
-p, --proxy <proxy> Update request proxy
-cp, --copy Copy remote url to clipboard
--qrcode-output <qrcodeOutputPath> Qrcode output path
const tma = require('tt-ide-cli');
// uploadResult 返回值
interface ProjectQRCode {
expireTime: number; // 二维码过期时间
shortUrl: string; // 二维码短链
originSchema: string; // 二维码 schema
qrcodeSVG?: string; // 二维码 SVG
qrcodeFilePath?: string; // 二维码存储路径
useCache: boolean; // 是否命中并使用缓存
}
const uploadResult: ProjectQRCode = await tma.upload({
project: {
path: 'projectPath', // 项目地址
},
qrcode: {
format: 'imageSVG', // imageSVG | imageFile | null | terminal
// imageSVG 用于产出二维码 SVG
// imageFile 用于将二维码存储到某个路径
// terminal 用于将二维码在控制台输出
// null 则不产出二维码
output: '', // 只在 imageFile 生效,填写图片输出绝对路径
options: {
small: false, // 使用小二维码,主要用于 terminal
},
},
copyToClipboard: true, // 是否将产出的二维码链接复制到剪切板
changeLog: 'changelog', // 本次更新日志
version: '1.0.0', // 本次更新版本
needUploadSourcemap: true, // 是否上传后生成 sourcemap,推荐使用 true,否则开发者后台解析错误时将不能展示原始代码
});
查询小程序支持的 host 。
Usage: tma hosts [appid]
Get Audit Host List
tma hosts tt07e3715e98c9xxxx
const tma = require('tt-ide-cli');
await tma.getAuditHostsList({ appid: '' });
小程序提审
推荐首次提审时,到开发者平台上传合规截图;
douyin
toutiao
douyin_lite
tt_lite
tma hosts [appid]
的命令查询Usage: tma audit [options] [appid]
Audit project in the developer platform
Options:
--host <hosts> Host Apps(eg: douyin,toutiao,tt_lite)
--auto-publish <boolean> Auto Publish After Audit
# 使用示范
tma audit --host douyin,toutiao,tt_lite tt07e3715e98c9xxxx
const tma = require('tt-ide-cli');
// 提审
await tma.audit({
appid: '',
host: [], // douyin,toutiao,tt_lite
autoPublish: true, // 是否审核通过后自动发布
defaultSsUrl: '/tmp/picture/default.png',
});
Usage: tma get-meta [options] [appid]
Get appid meta
const tma = require('tt-ide-cli');
// metaResult 返回值
interface MiniappMetaInfo {
version: String; // 线上小程序版本号
}
const metaResult: MiniappMetaInfo = await tma.getMeta({
appid: 'appid',
});
对应开发者工具 构建 NPM 功能
Usage: tma build-npm [options]
Build npm
Options:
--project-path Project path
const tma = require('tt-ide-cli');
await tma.buildNpm({
project: {
path: 'projectPath',
},
});
FAQs
Command line interface for micro app development
The npm package tt-ide-cli receives a total of 94 weekly downloads. As such, tt-ide-cli popularity was classified as not popular.
We found that tt-ide-cli demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.