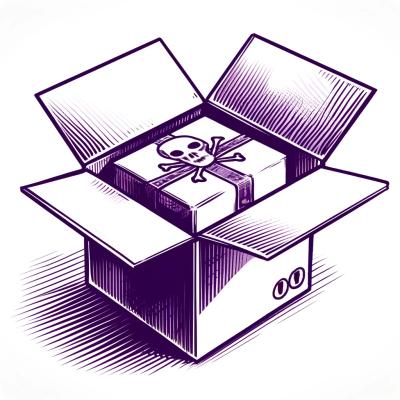
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
tte-api-services
Advanced tools
Using npm:
$ npm i tte-api-services
// In Browser:
var { contentService } = require('tte-api-services');
// or
import { contentService } from 'tte-api-services';
// or
import apiServices from 'tte-api-services';
// In Node.js:
var { contentService } = require('tte-api-services/node');
// or
import { contentService } from 'tte-api-services/node';
// or
import apiServices from 'tte-api-services/node';
Usage:
*
All requests in the services methods are asynchronous
**
Params in the square brackets are non-mandatory
Basket Service in the developer portal
Param | Type |
---|---|
environment | Environment |
[settings] | Settings |
[sourceInformation] | SourceInformation |
interface Settings {
basketApiUrl?: string;
}
interface SourceInformation {
sourceName?: string;
sourceVersion?: string;
viewName?: string;
}
Get basket by reference Returns: Basket - basket model
Param | Type |
---|---|
reference | string |
[channelId] | string |
returnTTId | bool |
Create new basket Returns: Basket - basket model
Param | Type |
---|---|
basketData | RequestBasketData |
returnTTId | bool |
interface RequestBasketData {
channelId: string;
reservations: RequestBasketItemData[];
reference?: string;
checksum?: string;
delivery?: DeliveryData;
createdAt?: string;
expiredAt?: string;
status?: BasketStatus;
coupon?: CouponData;
shopperReference?: string;
shopperCurrency?: string;
exchangeRate?: number;
}
interface RequestBasketItemData {
productId: string;
venueId: string;
quantity: number;
items: RequestSeat[];
date: string; /* date format: 'YYYY-MM-DDTHH:mm:ssZ' */
id?: string;
productName?: string;
productType?: ProductType;
venueName?: string;
adjustedSalePriceInShopperCurrency?: Amount;
faceValueInShopperCurrency?: Amount | null;
adjustmentAmountInShopperCurrency?: Amount;
salePriceInShopperCurrency?: Amount;
feeInShopperCurrency?: Amount | null;
deliveryFeeInShopperCurrency?: Amount | null;
}
interface RequestSeat {
aggregateReference: string;
blockId?: string;
blockName?: string;
row?: string;
number?: string;
locationDescription?: string;
}
interface Amount {
value: number;
currency: string;
decimalPlaces?: number;
}
enum ProductType {
Show = "SHW",
Attraction = "ATT",
Flexitickets = "FLX",
GiftVoucher = "GVC",
Postage = "PST"
}
Get delivery options available for basket Returns: Array<Delivery> - delivery models
Param | Type |
---|---|
basketReference | string |
basketItems | BasketItemsCollection |
[channelId] | string |
Set delivery option Returns: Basket - basket
Param | Type |
---|---|
basket | Basket |
selectedDelivery | DeliveryData |
interface DeliveryData {
method: DeliveryMethod;
charge: Amount;
}
enum DeliveryMethod {
Collection = "collection",
Postage = "postage",
Eticket = "eticket",
Evoucher = "evoucher",
PrintBoxOffice = 'print_box_office',
HandDelivered = 'hand_delivered',
DelayedBarcode = 'delayed_barcode',
Streaming = 'streaming',
Supplier = 'supplier',
}
interface Amount {
value: number;
currency: string;
decimalPlaces?: number;
}
Add item to the basket Returns: Basket - basket
Param | Type |
---|---|
basket | Basket |
basketItems | Array<BasketItemData> |
returnTTId | bool |
interface BasketItemData {
id: string;
productId: string;
productName: string;
productType: ProductType;
venueId: string;
venueName: string;
date: string; /* date format: 'YYYY-MM-DDTHH:mm:ssZ' */
quantity: number;
items: ReservationSeat[];
adjustedSalePriceInShopperCurrency: Amount;
salePriceInShopperCurrency: Amount;
faceValueInShopperCurrency: Amount | null;
adjustmentAmountInShopperCurrency: Amount;
feeInShopperCurrency: Amount | null;
deliveryFeeInShopperCurrency: Amount | null;
linkedReservationId?: number;
seats?: ReservationSeat[];
}
enum ProductType {
Show = "SHW",
Attraction = "ATT",
Flexitickets = "FLX",
GiftVoucher = "GVC",
Postage = "PST"
}
interface ReservationSeat {
aggregateReference: string;
areaId: string;
areaName: string;
row: string;
number: string;
locationDescription: string;
}
interface Amount {
value: number;
currency: string;
decimalPlaces?: number;
}
Replace items in basket with new items Returns: Basket - basket
Param | Type |
---|---|
basket | Basket |
basketItems | Array<BasketItemData> |
returnTTId | bool |
interface BasketItemData {
id: string;
productId: string;
productName: string;
productType: ProductType;
venueId: string;
venueName: string;
date: string; /* date format: 'YYYY-MM-DDTHH:mm:ssZ' */
quantity: number;
items: ReservationSeat[];
adjustedSalePriceInShopperCurrency: Amount;
salePriceInShopperCurrency: Amount;
faceValueInShopperCurrency: Amount | null;
adjustmentAmountInShopperCurrency: Amount;
feeInShopperCurrency: Amount | null;
deliveryFeeInShopperCurrency: Amount | null;
linkedReservationId?: number;
seats?: ReservationSeat[];
}
enum ProductType {
Show = "SHW",
Attraction = "ATT",
Flexitickets = "FLX",
GiftVoucher = "GVC",
Postage = "PST"
}
interface ReservationSeat {
aggregateReference: string;
areaId: string;
areaName: string;
row: string;
number: string;
locationDescription: string;
}
interface Amount {
value: number;
currency: string;
decimalPlaces?: number;
}
Remove item from the basket Returns: Basket - basket
Param | Type |
---|---|
basketReference | string |
itemId | number |
[channelId] | string |
Apply promo code Returns: Basket - basket
Param | Type |
---|---|
basket | Basket |
promoCode | string |
returnTTId | bool |
Remove promo code Returns: Basket - basket
Param | Type |
---|---|
basket | Basket |
returnTTId | bool |
Remove all items from basket Returns: Basket - basket
Param | Type |
---|---|
basketReference | string |
[channelId] | string |
Checkout Service in the developer portal
Param | Type |
---|---|
environment | Environment |
[checkoutApiUrl] | string |
[sourceInformation] | SourceInformation |
interface SourceInformation {
sourceName?: string;
sourceVersion?: string;
viewName?: string;
}
create new Order Returns: Order - new order model
Param | Type |
---|---|
bookingData | ApiBookingData |
[channelId] | string |
interface ApiBookingData {
channelId: string;
billingAddress: ApiAddress;
deliveryMethod: ApiDeliveryMethodCheckout;
redirectUrl: string;
reference: string;
shopper: ApiShopper;
origin?: string;
deliveryCharge?: number;
recipientName?: string;
giftVoucherMessage?: string;
hasFlexiTickets?: boolean;
paymentType?: PaymentType;
deliveryAddress?: ApiAddress;
}
type ApiDeliveryMethodCheckout = 'C' | 'E' | 'M';
interface ApiShopper {
firstName: string;
lastName: string;
email?: string;
title?: string;
telephoneNumber?: string;
externalId?: string;
}
interface ApiAddress {
countryCode: string;
line1?: string;
line2?: string;
postalCode?: string;
state?: string;
city?: string;
countryName?: string;
stateOrProvince?: string;
}
enum PaymentType {
Card = 'card',
Amex = 'amex',
Paypal = 'paypal',
Account = 'account',
Alipay = 'alipay',
Wechatpay = 'wechatpay',
}
Confirm booking Returns: ApiConfirmBooking - API booking confirmation response
Param | Type |
---|---|
reference | string |
channelId | string |
paymentId | string |
[agentDetails] | ApiConfirmBookingAgentDetails |
interface ApiConfirmBookingAgentDetails {
agentId: string;
agentPassword: string;
}
Content Service in the developer portal
Param | Type |
---|---|
environment | Environment |
[settings] | Settings |
[sourceInformation] | SourceInformation |
interface Settings {
contentApiUrl?: string;
contentImagesUrl?: string;
}
interface SourceInformation {
sourceName?: string;
sourceVersion?: string;
viewName?: string;
}
Get list of products Returns: Array<Product> - product models
Param | Type |
---|---|
[page] | number |
[limit] | number |
Get product details Returns: Product - product model
Param | Type |
---|---|
productId | string |
getContentFromV3 | bool |
Get product from V3 details Returns: ProductV3 - product model
Param | Type |
---|---|
productId | string |
Get list of images Returns: Array<Image> - image models
Param | Type |
---|---|
entityType | EntityType |
entityId | string |
[orientation] | ImageOrientation |
enum EntityType {
Products = "products"
}
enum ImageOrientation {
Square = "square",
Landscape = "landscape",
Portrait = "portrait",
Default = "default"
}
Inventory Service in the developer portal
Param | Type |
---|---|
environment | Environment |
[inventoryApiUrl] | string |
[sourceInformation] | SourceInformation |
interface SourceInformation {
sourceName?: string;
sourceVersion?: string;
viewName?: string;
}
Get performance availability Returns: Availability - performance availability models
Param | Type |
---|---|
affiliateId | string |
productId | string |
quantity | number |
date | string (YYYYMMDD) |
time | string (HHmm) |
Get max quantity Returns: number - max quantity
Param | Type |
---|---|
productId | string |
[affiliateId] | string |
Get summary availability Returns: SummaryAvailability - summary availability models
Param | Type |
---|---|
affiliateId | string |
productId | string |
quantity | number |
fromDate | string (YYYYMMDD) |
toDate | string (YYYYMMDD) |
Pricing Service in the developer portal
Param | Type |
---|---|
environment | Environment |
[pricingApiUrl] | string |
[sourceInformation] | SourceInformation |
interface SourceInformation {
sourceName?: string;
sourceVersion?: string;
viewName?: string;
}
Get list of from prices Returns: Array<FromPrices> - from prices models
Param | Type |
---|---|
productId | string |
Venue Service in the developer portal
Param | Type |
---|---|
environment | Environment |
[venueApiUrl] | string |
[sourceInformation] | SourceInformation |
interface SourceInformation {
sourceName?: string;
sourceVersion?: string;
viewName?: string;
}
Get list of seat attributes Returns: Array<SeatAttributes> - seat attribute models
Param | Type |
---|---|
venueId | string |
[performanceDate] | string |
[performanceTime] | string |
performanceDate should have format YYYY-MM-DD, e.g. 2021-12-24 performanceTime should have format HH:MM e.g. 12:30
Get list of seat attributes by seat id Returns: Array<SeatAttributes> - seat attribute models
Param | Type |
---|---|
venueId | string |
seatIdCollection | array |
seatIdCollection should contain array with seat identifier e.g. [STALLS-A13, STALLS-R24]
Get venue details Returns: Object<VenueDetails> - venue details models
Param | Type |
---|---|
venueId | string |
Get chart details Returns: Object<ChartDetails> - chart details models
Param | Type |
---|---|
venueId | string |
[date] | string |
Get venue chart Returns: VenueChart; - venue chart model
Param | Type |
---|---|
venueChartKey | string |
FAQs
[Developer portal](https://developer.encore.co.uk/)
We found that tte-api-services demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.