What is tty-table?
The tty-table npm package is used to create and display tables in the terminal. It provides a simple and flexible way to format and present tabular data in a visually appealing manner.
What are tty-table's main functionalities?
Basic Table Creation
This feature allows you to create a basic table with specified headers and rows. The table is then rendered and displayed in the terminal.
const ttyTable = require('tty-table');
const header = [
{ value: 'Name', width: 30, headerColor: 'cyan' },
{ value: 'Age', width: 10, headerColor: 'cyan' }
];
const rows = [
['Alice', 30],
['Bob', 25]
];
const table = ttyTable(header, rows);
console.log(table.render());
Customizing Table Appearance
This feature allows you to customize the appearance of the table, including border style, border color, padding, alignment, and text color.
const ttyTable = require('tty-table');
const header = [
{ value: 'Name', width: 30, headerColor: 'cyan', color: 'white', align: 'left' },
{ value: 'Age', width: 10, headerColor: 'cyan', color: 'white', align: 'right' }
];
const rows = [
['Alice', 30],
['Bob', 25]
];
const options = {
borderStyle: 'solid',
borderColor: 'blue',
paddingBottom: 0,
headerAlign: 'center',
align: 'center',
color: 'white'
};
const table = ttyTable(header, rows, options);
console.log(table.render());
Adding Footers
This feature allows you to add footers to the table, which can be used to display summary information or totals.
const ttyTable = require('tty-table');
const header = [
{ value: 'Name', width: 30, headerColor: 'cyan' },
{ value: 'Age', width: 10, headerColor: 'cyan' }
];
const rows = [
['Alice', 30],
['Bob', 25]
];
const footer = [
{ value: 'Total', colspan: 1, align: 'right' },
{ value: '2', align: 'right' }
];
const table = ttyTable(header, rows, { footer });
console.log(table.render());
Other packages similar to tty-table
cli-table
cli-table is another npm package for creating tables in the terminal. It offers similar functionality to tty-table but with a different API. cli-table is known for its simplicity and ease of use, making it a popular choice for basic table creation.
table
The table package provides a more advanced and flexible way to create tables in the terminal. It supports a wide range of customization options and is suitable for more complex table layouts. Compared to tty-table, the table package offers more features but may have a steeper learning curve.
easy-table
easy-table is a lightweight package for creating tables in the terminal. It focuses on simplicity and ease of use, making it a good choice for quick and straightforward table creation. While it may not offer as many customization options as tty-table, it is very easy to get started with.
tty-table 电传打字台

Display your data in a table using a terminal, browser, or browser console.
See here for complete example list
Terminal (Static)
examples/styles-and-formatting.js

Terminal (Streaming)
$ node examples/data/fake-stream.js | tty-table --format json --header examples/config/header.js
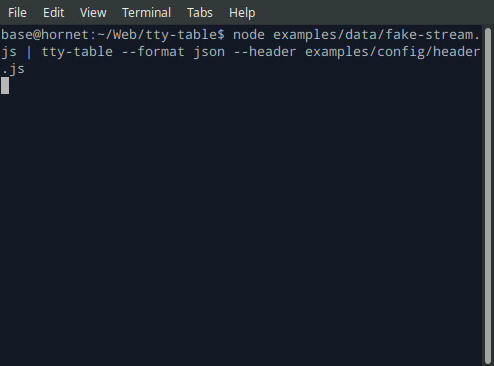
- See the built-in help for the terminal version of tty-table with:
$ tty-table -h
Browser & Browser Console

Working Example in Browser
Note that neither ASCI colors nor default borders are rendered in the browser.
An alternative border style, as shown below, should be used by setting the following option:
borderStyle : "dashed"
API Reference
Param | Type | Description |
---|
alias | string | Text to display in column header cell |
align | string | default: "center" |
color | string | default: terminal default color |
footerAlign | string | default: "center" |
footerColor | string | default: terminal default color |
formatter | function(cellValue, columnIndex, rowIndex, rowData, inputData) | Runs a callback on each cell value in the parent column |
headerAlign | string | default: "center" |
headerColor | string | default: terminal's default color |
marginLeft | integer | default: 0 |
marginTop | integer | default: 0 |
width | string || integer | default: "auto" |
paddingBottom | integer | default: 0 |
paddingLeft | integer | default: 1 |
paddingRight | integer | default: 1 |
paddingTop | integer | default: 0 |
value | string | Name of the property to display in each cell when data passed as an array of objects |
Example
let header = [
{
alias: "my items",
value: "item",
headerColor: "cyan",
color: "white",
align: "left",
paddingLeft: 5,
width: 30
},
{
value: "price",
color: "red",
width: 10,
formatter: function(cellValue) {
var str = `$${cellValue.toFixed(2)}`
if(value > 5) {
str = chalk.underline.green(str)
}
return str
}
}
]
rows array
Example
const rows = [
["hamburger",2.50],
]
const rows = [
{
item: "hamburger",
price: 2.50
}
]
Example
const footer = [
"TOTAL",
(cellValue, columnIndex, rowIndex, rowData, inputData) => {
return rowData.reduce((prev, curr) => {
return prev + curr[1]
}, 0)
},
(cellValue, columnIndex, rowIndex, rowData, inputData) => {
let total = rowData.reduce((prev, curr) => {
return prev + ((curr[2] === "yes") ? 1 : 0)
}, 0)
return `${ (total / rowData.length * 100).toFixed(2) }%`
}
]
options object
Param | Type | Description |
---|
borderStyle | string | default: "solid". "solid", "dashed", "none" |
borderColor | string | default: terminal default color |
color | string | default: terminal default color |
compact | boolean | default: false Removes horizontal lines when true. |
defaultErrorValue | mixed | default: '�' |
defaultValue | mixed | default: '?' |
errorOnNull | boolean | default: false |
truncate | mixed | default: false When this property is set to a string, cell contents will be truncated by that string instead of wrapped when they extend beyond of the width of the cell. For example if: "truncate":"..." the cell will be truncated with "..." |
Example
const options = {
borderStyle: 1,
borderColor: "blue",
headerAlign: "center",
align: "left",
color: "white",
truncate: "..."
}
Table.render() ⇒ String
Add method to render table to a string
Example
const out = Table(header,rows,options).render()
console.log(out);
Installation
$ npm install tty-table -g
$ npm install tty-table
<script src="tty-table.bundle.min.js"></script>
<script>
const Table = require('tty-table');
...
</script>
Running tests
$ npm test
Saving the output of new unit tests
$ npm run save-tests
node script.js --color=always
Dev Tips
- To generate vim tags (make sure jsctags is installed globally)
$ npm run tags
- To generate vim tags on file save
$ npm run watch-tags
Pull Requests
Pull requests are encouraged!
- Please remember to add a unit test when necessary
- Please format your commit messages according to the "Conventional Commits" specification
If you aren't familiar with Conventional Commits, here's a good article on the topic
TL/DR:
- feat: a feature that is visible for end users.
- fix: a bugfix that is visible for end users.
- chore: a change that doesn't impact end users (e.g. chances to CI pipeline)
- docs: a change in the README or documentation
- refactor: a change in production code focused on readability, style and/or performance.
License
MIT License
Copyright 2015-2020, Tecfu.