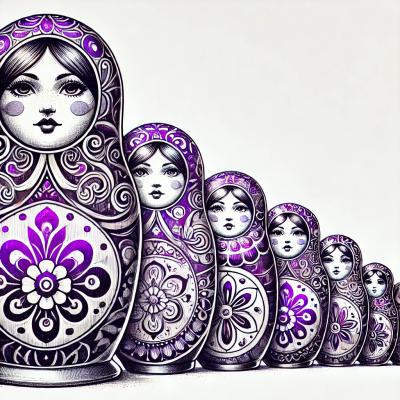
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
A lightweight viritual-dom with a friendly interface.
Many virtual-dom implementations are bulky and are not optimized for immutable data or server side rendering. Currently this is experimental and should not be used in production.
npm install tusk
/** @jsx tusk */
let tusk = require('tusk');
// Using immstruct for example, feel free to replace with immutable.js or others.
let immstruct = require('immstruct');
// Define some initial state for the app.
let struct = immstruct({ i : 0 });
function MyCounter (props, children) {
let { message, cursor } = props;
// Define handlers.
let handleClick = (e)=> cursor.update((state)=> state.set("i", state.get("i") + 1));
// Render the component.
return (
<button onClick={ handleClick }>
{ message } : { cursor.get('i') }
</button>
);
}
function render () {
tusk.render(document.body,
<MyCounter message="Times clicked" cursor={ struct.cursor() }/>
)
}
// Initial render
render()
// We can use the render function to re-render when the state changes.
struct.on("next-animation-frame", render)
// We can also render into a string (Usually for the server).
let HTML = String(<MyCounter type="Times clicked" cursor={ struct.cursor() }/>);
// -> "<button>Times clicked : 0</button>"
node
inside of an HTML Entity
.tusk.render(document.body, <div>Hello World</div>);
// -> document.body.innerHTML === "<div>Hello World</div>"
context
.// renderer must be a function that returns a virtual node.
function MyComponent (props, children, context) {
<div>External data: { context }</div>
}
String(tusk.with(1, ()=> <MyComponent/>));
//-> "<div>External Data: 1</div>"
// Automatically called when using JSX.
let vNode = tusk.createElement("div", { editable: true }, "Hello World");
// Or call tusk directly
let vNode = tusk("div", { editable: true }, "Hello World");
// Render to string on the server.
vNode.toString(); // '<div editable="true">Hello World</div>';
/**
* @params type can also be a function (shown in example above).
*/
In React and many other virtual doms "shouldUpdate" is a common theme for performance. Tusk does not feature shouldUpdate and opts for a more performant, simpler, and well known approach: memoization.
Basically Tusk will never re-render when given the same node twice, meaning the following will only render once. Tusk will also intelegently cloneNodes if memoized nodes are inserted in multiple places throughout the document.
let _ = require("lodash");
let i = 0;
let MyDiv = _.memoize(function () {
console.log(++i);
return (
<div>Hello World</div>
);
});
// creates and renders myDiv.
tusk.render(document.getElementById("component1"), <MyDiv/>);
i; // -> 1
// noop.
tusk.render(document.getElementById("component1"), <MyDiv/>);
i; // -> 1
// Uses #cloneNode on the previously rendered element. (Much faster than creating it).
tusk.render(document.getElementById("component2"), <MyDiv/>);
i; // -> 1
Please feel free to create a PR!
FAQs
Automation library
We found that tusk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.