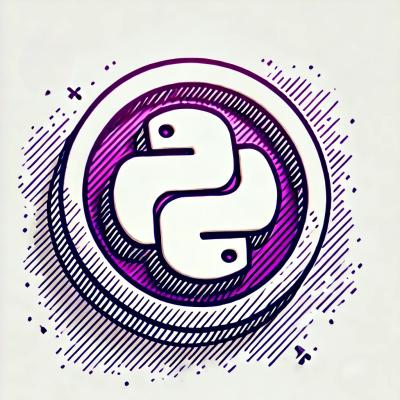
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
A easy to use twitter api client
Tweets.ts is a continuation of project Tweets.js, rewritten in typescript, from now on, use tweets.ts instead of tweets.js (tweets.js will be deprecated in favour of this)
npm i tweets.ts
import { Client } from 'tweets.ts';
const client = new Client({
authorization: {
consumer_key: 'Your Consumer Key',
consumer_secret: 'Your Consumer Secret',
access_token: 'Your access Token',
access_token_secret: 'Your Access Token Secret',
}
});
//... do what ever you want, use tweets.axix.cf for docs
// this is a example
client.getFollowers('typicalninja69', { count: 20 }).then(res => console.log('Here is typicalninja\'s Followers', res)).catch(err => console.log('error occurred', err));
import { StreamClient, Tweet } from 'tweets.ts'
// or...
import { StreamClient, Client, Tweet } from 'tweets.ts'
// if passing the whole client to streamClient (recommended)
const client = new Client({
authorization: {
consumer_key: 'Your Consumer Key',
consumer_secret: 'Your Consumer Secret',
access_token: 'Your access Token',
access_token_secret: 'Your Access Token Secret',
}
});
// ... if passing whole Client to streamClient (recommended)
const streamClient = new StreamClient(client)
/*
else....
const streamClient = new StreamClient({
authorization: {
consumer_key: 'Your Consumer Key',
consumer_secret: 'Your Consumer Secret',
access_token: 'Your access Token',
access_token_secret: 'Your Access Token Secret',
}
})
*/
// this is a must
streamClient.stream({ endPoint: '/statuses/filter', body: { follow: "1238451949000888322", } });
streamClient.on('connected', () => {
console.log('Stream Client is now Connected')
});
streamClient.on('end', (reason: string) => {
console.log('Stream Ended due to:', reason)
});
streamClient.on('tweet', (tweet: Tweet) => {
console.log('New Tweet:')
console.log(tweet)
});
// to end this client
setTimeout(() => {
streamClient.end('Time ended, we don\'t need a stream now');
}, 10000)
const { Client } = require('tweets.ts');
const client = new Client({
authorization: {
consumer_key: 'Your Consumer Key',
consumer_secret: 'Your Consumer Secret',
access_token: 'Your access Token',
access_token_secret: 'Your Access Token Secret',
}
});
//... do what ever you want, use tweets.axix.cf for docs
// this is a example
client.getFollowers('typicalninja69', { count: 20 }).then(res => console.log('Here is typicalninja\'s Followers', res)).catch(err => console.log('error occurred', err));
const { StreamClient } = require('tweets.ts');
// or...
const { StreamClient, Client } = require('tweets.ts')
// if passing the whole client to streamClient (recommended)
const client = new Client({
authorization: {
consumer_key: 'Your Consumer Key',
consumer_secret: 'Your Consumer Secret',
access_token: 'Your access Token',
access_token_secret: 'Your Access Token Secret',
}
});
// ... if passing whole Client to streamClient (recommended)
const streamClient = new StreamClient(client)
/*
else....
const streamClient = new StreamClient({
authorization: {
consumer_key: 'Your Consumer Key',
consumer_secret: 'Your Consumer Secret',
access_token: 'Your access Token',
access_token_secret: 'Your Access Token Secret',
}
})
*/
// this is a must
streamClient.stream({ endPoint: '/statuses/filter', body: { follow: "1238451949000888322", } });
streamClient.on('connected', () => {
console.log('Stream Client is now Connected')
});
streamClient.on('end', (reason) => {
console.log('Stream Ended due to:', reason)
});
streamClient.on('tweet', (tweet) => {
console.log('New Tweet:')
console.log(tweet)
});
// to end this client
setTimeout(() => {
streamClient.end('Time ended, we don\'t need a stream now');
}, 10000)
Raw api is querying the api using builtin methods like post(), get(), you completely controls these methods. so least support is given for usage of this
client is a instance of tweet.ts client
// returns a promise, must be inside a async function, unless you use .then
const request = await client.get({ endPoint: '/endPoint/here/:id', bodyOrParams: { id: 'this will be in the endpoint param', someOtherParam: 'follow' } })
// raw json data
console.log(request);
// returns a promise, must be inside a async function, unless you use .then
const request = await client.post({ endPoint: '/endPoint/here/to/post/:id', bodyOrParams: { id: 'this will be in the endpoint param', someOtherBody: 'this will be in the body' } })
// raw json data
console.log(request);
See this url : Click here
Sadly you cant, in typescript rewrite (tweets.ts) App authentication support was removed, You can use another library like this one for app authentication purpose
You can use our Discord server to get support, join here
👤 typicalninja21
Give a ⭐️ if this project helped you!
This README was generated with ❤️ by readme-md-generator
FAQs
A easy to use twitter api client written in typescript
We found that tweets.ts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.