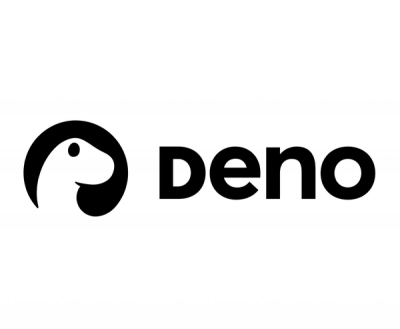
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
The Twilio npm package is a Node.js client library that provides tools to interact with the Twilio API for various communication solutions, including SMS, voice calls, video, chat, and more. It allows developers to programmatically send and receive text messages, make and receive phone calls, handle authentication with two-factor authentication, and work with other Twilio services.
Sending SMS
This feature allows you to send SMS messages to a specified phone number using Twilio's messaging API.
const twilio = require('twilio');
const client = new twilio('ACCOUNT_SID', 'AUTH_TOKEN');
client.messages.create({
body: 'Hello from Twilio!',
to: '+12345678901',
from: '+10987654321'
}).then(message => console.log(message.sid));
Making Voice Calls
This feature enables you to make outbound voice calls to phones around the world with a specified TwiML URL to control the call flow.
const twilio = require('twilio');
const client = new twilio('ACCOUNT_SID', 'AUTH_TOKEN');
client.calls.create({
url: 'http://demo.twilio.com/docs/voice.xml',
to: '+12345678901',
from: '+10987654321'
}).then(call => console.log(call.sid));
Handling Two-Factor Authentication
This feature is used for sending verification tokens for two-factor authentication, enhancing security for user accounts.
const twilio = require('twilio');
const client = new twilio('ACCOUNT_SID', 'AUTH_TOKEN');
client.verify.services('SERVICE_SID')
.verifications
.create({to: '+12345678901', channel: 'sms'})
.then(verification => console.log(verification.status));
Working with Video
This feature allows you to create video rooms where multiple participants can join for video conferencing.
const twilio = require('twilio');
const client = new twilio('ACCOUNT_SID', 'AUTH_TOKEN');
client.video.rooms.create({
uniqueName: 'DailyStandup'
}).then(room => console.log(room.sid));
Nexmo, now known as Vonage API, is a service similar to Twilio that provides APIs for SMS, voice, phone verifications, and other communication services. It offers similar functionality but with different pricing and API design.
Plivo is another cloud communication platform that enables businesses to integrate voice and SMS capabilities into their applications. It is often compared to Twilio in terms of features and services offered.
Bandwidth offers a range of communication APIs, including voice, messaging, and 911 access. It is a direct competitor to Twilio and provides similar services with a focus on enterprise solutions.
Sinch provides cloud-based communication services and APIs for SMS, voice, video, and verification. It competes with Twilio by offering a suite of communication tools for developers.
The documentation for the Twilio API can be found here.
The Node library documentation can be found here.
twilio-node
uses a modified version of Semantic Versioning for all changes. See this document for details.
This library supports the following Node.js implementations:
TypeScript is supported for TypeScript version 2.9 and above.
npm install twilio
or yarn add twilio
Or, if you're wanting to install the latest release candidate use:
npm install twilio@rc
or yarn add twilio@rc
Check out these code examples in JavaScript and TypeScript to get up and running quickly.
twilio-node
supports credential storage in environment variables. If no credentials are provided when instantiating the Twilio client (e.g., const client = require('twilio')();
), the values in following env vars will be used: TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
.
If your environment requires SSL decryption, you can set the path to CA bundle in the env var TWILIO_CA_BUNDLE
.
twilio-node
supports lazy loading required modules for faster loading time. Lazy loading is disabled by default. To enable lazy loading, simply instantiate the Twilio client with the lazyLoading
flag set to true
:
var accountSid = process.env.TWILIO_ACCOUNT_SID; // Your Account SID from www.twilio.com/console
var authToken = process.env.TWILIO_AUTH_TOKEN; // Your Auth Token from www.twilio.com/console
const client = require('twilio')(accountSid, authToken, {
lazyLoading: true
});
To take advantage of Twilio's Global Infrastructure, specify the target Region and/or Edge for the client:
var accountSid = process.env.TWILIO_ACCOUNT_SID; // Your Account SID from www.twilio.com/console
var authToken = process.env.TWILIO_AUTH_TOKEN; // Your Auth Token from www.twilio.com/console
const client = require('twilio')(accountSid, authToken, {
region: 'au1',
edge: 'sydney',
});
Alternatively, specify the edge and/or region after constructing the Twilio client:
const client = require('twilio')(accountSid, authToken);
client.region = 'au1';
client.edge = 'sydney';
This will result in the hostname
transforming from api.twilio.com
to api.sydney.au1.twilio.com
.
There are two ways to enable debug logging in the default HTTP client. You can create an environment variable called TWILIO_LOG_LEVEL
and set it to debug
or you can set the logLevel variable on the client as debug:
var accountSid = process.env.TWILIO_ACCOUNT_SID; // Your Account SID from www.twilio.com/console
var authToken = process.env.TWILIO_AUTH_TOKEN; // Your Auth Token from www.twilio.com/console
const client = require('twilio')(accountSid, authToken, {
logLevel: 'debug'
});
You can also set the logLevel variable on the client after constructing the Twilio client:
const client = require('twilio')(accountSid, authToken);
client.logLevel = 'debug';
See example for a code sample for incoming Twilio request validation.
For an example on how to handle exceptions in this helper library, please see the Twilio documentation.
To use a custom HTTP client with this helper library, please see the Twilio documentation.
The Dockerfile
present in this repository and its respective twilio/twilio-node
Docker image are currently used by Twilio for testing purposes only.
If you need help installing or using the library, please check the Twilio Support Help Center first, and file a support ticket if you don't find an answer to your question.
If you've instead found a bug in the library or would like new features added, go ahead and open issues or pull requests against this repo!
Bug fixes, docs, and library improvements are always welcome. Please refer to our Contributing Guide for detailed information on how you can contribute.
⚠️ Please be aware that a large share of the files are auto-generated by our backend tool. You are welcome to suggest changes and submit PRs illustrating the changes. However, we'll have to make the changes in the underlying tool. You can find more info about this in the Contributing Guide.
If you're not familiar with the GitHub pull request/contribution process, this is a nice tutorial.
If you want to familiarize yourself with the project, you can start by forking the repository and cloning it in your local development environment. The project requires Node.js to be installed on your machine.
After cloning the repository, install the dependencies by running the following command in the directory of your cloned repository:
npm install
You can run the existing tests to see if everything is okay by executing:
npm test
To run just one specific test file instead of the whole suite, provide a JavaScript regular expression that will match your spec file's name, like:
npm run test:javascript -- -m .\*client.\*
FAQs
A Twilio helper library
The npm package twilio receives a total of 1,300,096 weekly downloads. As such, twilio popularity was classified as popular.
We found that twilio demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.