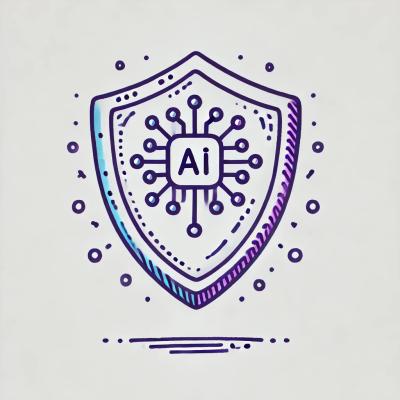
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
twitch-api-v5
Advanced tools
Simple module to use Twitch's API v5 for all endpoints. For details on optional parameters or required authentication scopes please follow the links to the Twitch docs.
This module also includes undocumented endpoints (such as the chatters endpoint to view which users are currently in a channels chat), these should be used at your own risk and are should be expected to change or be removed at any time.
npm install twitch-api-v5 --save
var api = require('twitch-api-v5');
api.clientID = 'Twitch app client-id';
api.user.getByID({ userID: '12826' }, (err, res) => {
if(err) {
console.log(err);
} else {
console.log(res);
/* Example response
{
display_name: 'Twitch',
_id: '12826',
name: 'twitch',
type: 'user',
...
}
*/
}
});
api.feed.createPost({ auth: 'OAuth ...', channelID: '12826', post: 'New Post!' }, (err, res) => {
...
});
To enable debugging of the HTTP requests, use api.debug = true;
Note: The authorization code needed for getAccessToken is obtained by steps 1 and 2 of the Authorization Code Flow described in the Twitch Docs.
Function | Auth Scope | Required Parameters |
---|---|---|
api.auth.getAccessToken | none | clientSecret, redirectURI, code |
api.auth.checkToken | any | auth |
api.auth.refreshToken | none | clientSecret, refreshToken |
Function | Auth Scope | Required Parameters |
---|---|---|
api.bits.cheermotes | none | none |
Function | Auth Scope | Required Parameters |
---|---|---|
api.feed.getPosts | any (optional) | channelID |
api.feed.getPost | any (optional) | postID |
api.feed.createPost | channel_feed_edit | auth, channelID, post |
api.feed.deletePost | channel_feed_edit | auth, channelID, postID |
api.feed.createReaction | channel_feed_edit | auth, channelID, postID, emoteID |
api.feed.deleteReaction | channel_feed_edit | auth, channelID, postID, emoteID |
api.feed.getComments | any (optional) | channelID, postID |
api.feed.createComment | channel_feed_edit | auth, channelID, postID, comment |
api.feed.deleteComment | channel_feed_edit | auth, channelID, postID, commentID |
api.feed.createCommentReaction | channel_feed_edit | auth, channelID, postID, commentID, emoteID |
api.feed.deleteCommentReaction | channel_feed_edit | auth, channelID, postID, commentID, emoteID |
Function | Auth Scope | Required Parameters |
---|---|---|
api.channels.channel | channel_read | auth |
api.channels.channelByID | none | channelID |
api.channels.updateChannel | channel_editor | channelID, and at least one of status, game, delay or channel_feed_enabled |
api.channels.editors | channel_read | auth, channelID |
api.channels.followers | none | channelID |
api.channels.teams | none | channelID |
api.channels.subs | channel_subscriptions | auth, channelID |
api.channels.checkSub | channel_check_subscription | auth, channelID, userID |
api.channels.videos | none | channelID |
api.channels.startAd | channel_commercial | auth, channelID, duration |
api.channels.resetStreamKey | channel_stream | auth, channelID |
api.channels.getCommunity | none | channelID |
api.channels.setCommunity | channel_editor | auth, channelID, communityID |
api.channels.leaveCommunity | channel_editor | auth, channelID |
Function | Auth Scope | Required Parameters |
---|---|---|
api.chat.badges | none | channelID |
api.chat.emoteSet | none | none |
api.chat.emotes | none | none |
Function | Auth Scope | Required Parameters |
---|---|---|
api.clips.getClip | none | slug |
api.clips.top | none | none |
api.clips.followed | user_read | auth |
Function | Auth Scope | Required Parameters |
---|---|---|
api.collections.getMetadata | none | collectionID |
api.collections.getCollection | none | collectionID |
api.collections.getByChannel | none | channelID |
api.collections.create | collections_edit | auth, channelID, title |
api.collections.update | collections_edit | auth, collectionID, title |
api.collections.createThumbnail | collections_edit | auth, collectionID, itemID |
api.collections.delete | collections_edit | auth, collectionID |
api.collections.addItem | collections_edit | auth, collectionID, videoID |
api.collections.delItem | collections_edit | auth, collectionID, itemID |
api.collections.moveItem | collections_edit | auth, collectionID, itemID, position |
Function | Auth Scope | Required Parameters |
---|---|---|
api.communities.getByName | none | name |
api.communities.getByID | none | communityID |
api.communities.update | communities_edit | auth, communityID |
api.communities.top | none | none |
api.communities.bans | communities_moderate | auth, communityID |
api.communities.addBan | communities_moderate | auth, communityID, userID |
api.communities.unBan | communities_moderate | auth, communityID, userID |
api.communities.createAvatar | communities_edit | auth, communityID, avatar_image |
api.communities.deleteAvatar | communities_edit | auth, communityID |
api.communities.createCover | communities_edit | auth, communityID, cover_image |
api.communities.deleteCover | communities_edit | auth, communityID |
api.communities.mods | communities_edit | auth, communityID |
api.communities.addMod | communities_edit | auth, communityID, userID |
api.communities.delMod | communities_edit | auth, communityID, userID |
api.communities.getPermissions | any | auth, communityID |
api.communities.report | none | channelID, communityID |
api.communities.timeouts | communities_moderate | auth, communityID |
api.communities.addTimeout | communities_moderate | auth, communityID, userID, duration |
api.communities.delTimeout | communities_moderate | auth, communityID, userID |
Function | Auth Scope | Required Parameters |
---|---|---|
api.games.top | none | none |
Function | Auth Scope | Required Parameters |
---|---|---|
api.ingests.serverList | none | none |
Function | Auth Scope | Required Parameters |
---|---|---|
api.search.channels | none | query |
api.search.games | none | query |
api.search.streams | none | query |
Function | Auth Scope | Required Parameters |
---|---|---|
api.streams.channel | none | channelID |
api.streams.live | none | none |
api.streams.summary | none | none |
api.streams.featured | none | none |
api.streams.followed | user_read | auth |
Function | Auth Scope | Required Parameters |
---|---|---|
api.teams.getAll | none | none |
api.teams.getTeam | none | team |
Function | Auth Scope | Required Parameters |
---|---|---|
api.users.user | user_read | auth |
api.users.userByID | none | userID |
api.users.usersByName | none | users |
api.users.userEmotes | user_subscriptions | auth, userID |
api.users.checkSub | user_subscriptions | auth, userID, channelID |
api.users.follows | none | userID |
api.users.checkFollow | none | userID, channelID |
api.users.followChannel | user_follows_edit | auth, userID, channelID |
api.users.unfollowChannel | user_follows_edit | auth, userID, channelID |
api.users.blockList | user_blocks_read | auth, userID |
api.users.blockUser | user_blocks_edit | auth, sourceUserID, targetUserID |
api.users.unblockUser | user_blocks_edit | auth, sourceUserID, targetUserID |
api.users.createVHS | viewing_activity_read | auth, identifier |
api.users.checkVHS | user_read | auth |
api.users.deleteVHS | viewing_activity_read | auth |
Function | Auth Scope | Required Parameters |
---|---|---|
api.videos.getVideo | none | videoID |
api.videos.top | none | none |
api.videos.followed | none | none |
api.videos.create | channel_editor | auth, channelID, title |
api.videos.upload | none | content-length, videoData, videoID, part, token |
api.videos.complete | none | videoID, token |
api.videos.update | channel_editor | auth, videoID |
api.videos.delete | channel_editor | auth, videoID |
Warning: These endpoints are undocumented and should be expected to change or cease to function at any point!
Function | Auth Scope | Required Parameters | Description |
---|---|---|---|
api.other.chatters | none | channelName | Usernames of people in chat in the specified channel |
api.other.hosts | none | channelID | Channels that are hosting the specified channelID |
api.other.hosting | none | channelID | Who the specified channelID is hosting |
api.other.subsTo | user_subscriptions | auth, channelName | Who a user is subbed to |
api.other.randomStream | none | none | Random stream |
api.other.getUser | none | channelName | Provides user data not returned in the documented endpoint |
api.other.chatProperties | none | channelName | Chat properties, such as subs only, rules etc... |
api.other.product | none | channelName | Channels subscription program details |
api.other.panels | none | channelName | Info on each of a channels panels |
api.other.playlist | none | channelID | Info on a currently running playlist |
api.other.followedHosting | none | channelName | Followed channels that are hosting someone else |
api.other.followedGames | none | channelName | Games that a user follows |
api.other.followedGamesLive | none | channelName | Live games that a user follows |
api.other.checkFollowGame | none | channelName, game | Check if a user follows a game |
api.other.badges | none | none | All chat badges |
api.other.subBadges | none | channelID | A channels sub badges |
api.other.recentChat | none | channelID | Recent chat in the specified channel |
api.other.cs | none | none | List of CS streams with extra details |
api.other.csMaps | none | none | List of CS maps and their viewers |
FAQs
Module for Twitch's v5 API
We found that twitch-api-v5 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.