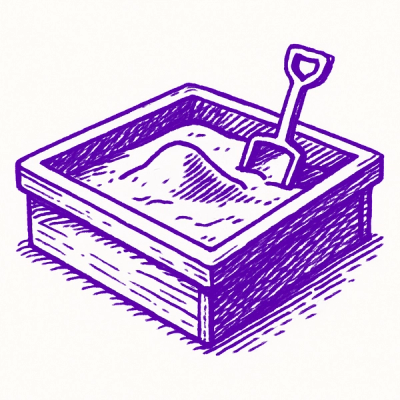
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
typed-qparam
Advanced tools
🔍 Type-safe query parameter manipulation
[!NOTE] See here for documentation on <=v1 features.
npm i typed-qparam
Passing a query parameter key to the qparam
function returns the accessor for that value.
import { extract } from 'typed-qparam'
const qparam = extract(new URL('https://example.com/?foo=bar'))
const foo = qparam('foo')
// output: 'bar'
console.log(foo.get())
// .set() only returns a new URL instance.
// The original URL instance is not changed.
// No navigation of any kind will occur.
// url: new URL('https://example.com/?foo=baz')
const url = foo.set('baz')
// output: 'https://example.com/?foo=baz'
console.log(url.href)
By passing a conversion function as the second argument, you can obtain a value converted to any type. See ts-serde for more information on type guard.
import { extract } from 'typed-qparam'
import { number } from 'typed-qparam/serde'
const qparam = extract(new URL('https://example.com/?num=123'))
const num = qparam('num', {
stringify: (value) => value.toString(),
parse: (str) => parseInt(str)
})
// output 123
console.log(num.get())
// https://example.com/?key=456
const dist = num.set(456)
You can also use the prepared converters in typed-qparam/serde
.
See ts-serde for more information on type guard
import { extract } from 'typed-qparam'
import { number, boolean, enums } from 'typed-qparam/serde'
const qparam = extract(
new URL('https://example.com/?num=123&bool=true&enumerate=b')
)
const num = qparam('num', number)
const bool = qparam('bool', boolean)
const enumerate = qparam(
'enumerate',
enums(
['a', 'b', 'c'],
'a' // fallback default value
)
)
Sometimes you need to handle query parameters with multiple values in the same key, such as ?str=hello&str=world
.
With typed-qparam
, you can treat this as an array.
import { extract, array } from 'svelte-qparam'
import { string, number } from 'svelte-qparam/serde'
const qparam = extract(new URL('https://example.com/?str=hello&str=world'))
const str = qparam('str', array())
// is equivalent to
// const str = qparam('str', array(string))
// if require other typed value
const num = qparam('num', array(number))
// output ['hello', 'world']
console.log(str.get())
// https://example.com/?str=foo&str=bar&str=baz
str.set(['foo', 'bar', 'baz'])
FAQs
🔍 Type-safe query parameter manipulation
The npm package typed-qparam receives a total of 8 weekly downloads. As such, typed-qparam popularity was classified as not popular.
We found that typed-qparam demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.