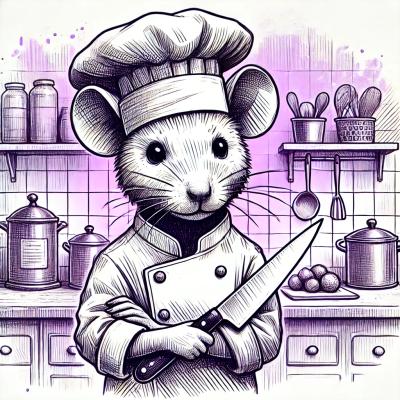
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
typed-signals
Advanced tools
A type-checked signal library written in TypeScript, usable from plain JavaScript as well. This is a TypeScript port of this excellent C++11 version: Performance of a C++11 Signal System. Of course, some changes have been made to make it work with TypeScript.
The original unit tests and additional ones are running automatically on Travis-CI
With version 2, the target is now es2015, so if you want to support older browser, you'll have to ensure that this module is being transpiled to an older es version during your build-process.
npm install typed-signals --save
import { Signal } from "typed-signals";
// Create a new signal, defining the function signature of handlers :
let mySignal = new Signal<(n: number, b: boolean, s: string) => void>();
//Register a handler:
let connection = mySignal.connect((n, b, s)=> console.log(`Called: ${n} ${b} ${s}`));
// Emit a signal:
mySignal.emit(42, true, 'Galactic Gargleblaster');
// Disconnect a handler:
connection.disconnect();
import { Signal, SignalConnections } from "typed-signals";
let mySignal = new Signal<() => void>();
// Disable and re-enable handlers
function handler42() {}
let connection = mySignal.connect(handler42);
connection.enabled = false;
mySignal.emit(); // won't call handler42
connection.enabled = true;
mySignal.emit(); // will call handler42
// Remember multiple connections and disconnect them all at once:
let connections = new SignalConnections();
connections.add(mySignal.connect(()=>{}));
connections.add(mySignal.connect(()=>{}));
connections.add(mySignal.connect(()=>{}));
connections.disconnectAll();
// Or disconnect all handlers of a signal:
mySignal.disconnectAll();
import { Signal } from "typed-signals";
let mySignal = new Signal<() => void>();
// Handlers are called in the order in which they are added:
mySignal.connect(()=>console.log('first'));
mySignal.connect(()=>console.log('second'));
mySignal.disconnectAll();
// Second parameter to connect is an order value. A higher order value means later execution:
mySignal.connect(()=>console.log('second'), 1);
mySignal.connect(()=>console.log('first'), 0);
Collectors can be used to stop processing further handlers depending on the return value of a handler and/or to collect return values of those handlers.
Built-in Collectors:
CollectorLast<THandler extends (...args: any[]) => any>
CollectorUntil0<THandler extends (...args: any[]) => boolean>
CollectorWhile0<THandler extends (...args: any[]) => boolean>
CollectorArray<THandler extends (...args: any[]) => any>
THandler
must be the same function signature as the signal. Here is an example:
import { Signal, CollectorLast } from "typed-signals";
let mySignal = new Signal<() => string>();
let collector = new CollectorLast<() => string>(mySignal);
mySignal.connect(()=> 'Hello World');
mySignal.connect(()=> 'Foo Bar');
collector.emit(); // calls signal.emit();
console.log(collector.getResult()); // 'Foo Bar'
Something not working quite as expected? Do you need a feature that has not been implemented yet? Check the issue tracker and add a new one if your problem is not already listed. Please try to provide a detailed description of your problem, including the steps to reproduce it.
Awesome! If you would like to contribute with a new feature or submit a bugfix, fork this repo and send a pull request. Please, make sure all the unit tests are passing before submitting and add new ones in case you introduced new features.
Typed-Signals has been released under the CC0 Public Domain license, meaning you can use it free of charge, without strings attached in commercial and non-commercial projects. Credits are appreciated but not mandatory.
FAQs
A type checked signal library for TypeScript (and JavaScript)
We found that typed-signals demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.