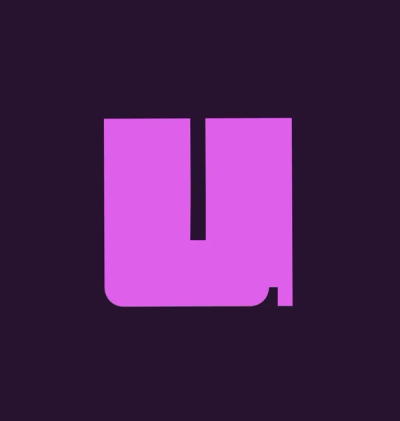
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
typedconverter
Advanced tools
Convert object into classes match with TypeScript type annotation
Convert object into classes match with TypeScript type annotation
import createConverter from "typedconverter";
const convert = createConverter()
const numb = await convert("12345", Number) //return number 12345
const numb = await convert("YES", Boolean) //return true
const numb = await convert("2019-1-1", Date) //return date 1/1/2019
Expected type can be specified in the configuration, than you can omit expected type on the second parameter of the convert
function. Useful when you want to covert several times without specifying expected type.
import createConverter from "typedconverter";
const convert = createConverter({type: Number})
const numb = await convert("12345")
const numb1 = await convert("-12345")
const numb2 = await convert("12345.123")
TypedConvert uses tinspector to get type metadata, so it aware about TypeScript type annotation.
import createConverter from "typedconverter";
import reflect from "tinspector"
const convert = createConverter()
@reflect.parameterProperties()
class AnimalClass {
constructor(
public id: number,
public name: string,
public deceased: boolean,
public birthday: Date
) { }
}
//return instance of AnimalClass with appropriate properties type
const data = await convert({
id: "200",
name: "Mimi",
deceased: "ON",
birthday: "2018-1-1" },
AnimalClass)
Convert into array by providing array of type in the expected type.
import createConverter from "typedconverter";
const convert = createConverter()
const numb = await convert(["1", "2", "-3"], [Number])
Nested child array need to be decorate for TypeScript added design data type
import createConverter from "typedconverter";
@reflect.parameterProperties()
class Tag {
constructor(
public name: string,
) { }
}
@reflect.parameterProperties()
class Animal {
constructor(
public name: string,
@reflect.array(Tag)
public tags: Tags
) { }
}
const convert = createConverter()
//tags is instance of Tag class
const numb = await convert({name: "Mimi", tags: [{name: "Susi"}, {name: "Lorem"}]}, Animal)
Useful when converting data from url encoded, where single value could be a single array.
const convert = createConverter({ guessArrayElement: true })
const b = await convert("1", [Number]) //ok
Provided custom converter on the configuration
import createConverter from "typedconverter";
const convert = createConverter({
type: Boolean,
converters: [{ type: Boolean, converter: async x => new ConversionResult("Custom Boolean") }]
})
const numb = await convert("True") //result: "Custom Boolean"
Visitors executed after conversion process traverse through properties / array element. Invocation can be multiple and run in sequence the last sequence will execute the converter. Visitors work like Plumier middleware
Signature of Visitor is like below:
type Visitor = (value: any, invocation: ConverterInvocation) => Promise<ConversionResult>
Visitor is a function receive two parameters value
and invocation
.
value
is current value traversedinvocation
next invocationFAQs
Convert object into classes match with TypeScript type annotation
We found that typedconverter demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.