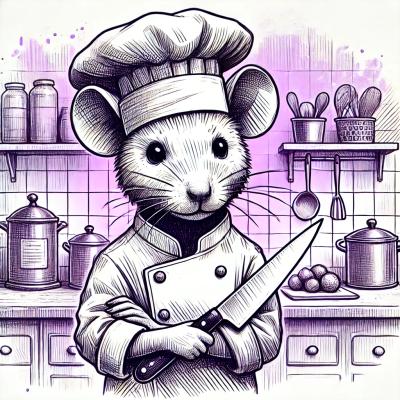
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
typeof-arguments
Advanced tools
typeof-arguments
validates the arguments' types passed through the enclosing function.
of-type
package to check if the given value|object is of expected type.typeof-properties
to validate the object's properties' type.typeof-items
to validate the array's items' typenpm install typeof-arguments
const type = require('typeof-arguments');
typeof-arguments.js
library to the HTML file.The library is located in ./dist/typeof-arguments.js
directory.
It is a webpack&babel bundled cross-browser library version.
The library is accessible as typeofArguments
variable in the global (window) scope.
<head>
<script src='typeof-arguments.js'></script>
<script>
function test(name, age) {
typeofArguments(arguments, ['string', 'number|string|null']);
}
test('Nikola', 26);
</script>
</head>
> git clone https://github.com/devrafalko/typeof-arguments.git
> cd typeof-arguments
> npm install
> npm test //run tests in node
> npm test deep //run tests in node with errors shown
type(actual, expected[, callback])
actual
[Object]It should always indicate the enclosing function arguments
object.
expected
[Array]expected
item's index coheres with the index of actual
argument item passed through the enclosing function.expected
items indicate the expected types of the coherent actual
arguments passed through the enclosing function.test('Nikola', 26);
function test(name, age) {
//the name should be of [String] type
//and the age should be of [Number|String|null] type
type(arguments, ['string', 'number|string|null']);
}
expected
TypesThere are four ways to check the type of the arguments:
null
or undefined
valuesMind, that the
typeof-arguments
library uses theof-type
library as the dependency, to validate the types. If you feel confused how to use the types, see more samples here.
[String]
'null'
, 'undefined'
constructor.name
, eg:'string'
, 'number'
, 'regexp'
, 'array'
, 'object'
, 'boolean'
,'buffer'
, etc.'String'
, 'string'
, 'StRiNg'
checks if the argument is of [String]
type'RegExp'
, 'REGEXP'
, 'regexp'
checks if the argument is of [RegExp]
type|
:
'array|object'
checks if the argument is of [Array]
OR
[Object]
type'undefined|null'
checks if the argument is of undefined
OR
null
typetest('Nikola', 26);
function test() {
type(arguments, ['string', 'number|string|null']);
}
[RegExp]
/null/
, /undefined/
constructor.name
, eg: /String/
, /Number/
, /RegExp/
, /Array/
, /Object/
, /Boolean/
,/Buffer/
, /Promise/
, etc./Str/
, /Err/
, /Reg/
, /B/
/.+Error$/
, /^RegExp$/
,/^[A-Z][a-z]+$/
i
flag:
/string/i
, /regexp/i
, /TYPEERROR/i
(x|y)
expression:
/String|Number/
, /TypeError|Error/
, /(obj|str)/i
test('Nikola', 26);
function test() {
type(arguments, [/string/i, /number|string|null/i]);
}
[Function|Array|null|undefined]
null
, undefined
[Function]
constructor, eg: String
, TypeError
, Promise
, Array
, etc.[String, Object, Array, null]
[null, undefined, Boolean]
test('Nikola', 26);
function test() {
type(arguments, [String, [Number, String, null]]);
}
When you use bundlers or minifiers, use
[String|RegExp]
type wisely as bundlers may change the names of functions|constructors|classes in the output file and eg.type(arguments, ['MyClass']);
that returnstrue
before compilation, may returnfalse
after compilation, if the bundler minifies the'MyClass'
constructor name.
[String] 'arguments'
| [RegExp] /arguments/
'arguments'
or /arguments/
expects the passed argument to be the function's arguments
object[String] 'instance'
| [RegExp] /instance/
'instance'
or /instance/
expects the passed argument to be the instance of the user's class|constructor[]
, 'hello world'
, {}
global
|window
's properties[String] 'objectable'
| [RegExp] /objectable/
'objectable'
or /objectable/
expects the passed argument to be the object that is the instance of the Object
constructor
{}
, []
, new String('hello world')
, new Boolean(1)
'hello world'
, true
, 10
, null
, undefined
[String] 'truthy'
| [RegExp] /truthy/
'truthy'
or /truthy/
expects the passed argument to be like:
'abc'
, true
, 1
, -1
, {}
, []
, function(){}
[String] 'falsy'
| [RegExp] /falsy/
'falsy'
or /falsy/
expects the passed argument to be like:
''
, false
, 0
, null
, undefined
, NaN
[String] 'any'
| [RegExp] /any/
| [Array] []
| [String] ""
type
'any'
or /any/
or empty array []
or empty string ""
expects the passed argument to be of any typecallback
[Function] (optional)Invalid argument [0]. The [Number] argument has been passed, while the argument of type [String] is expected.
Invalid argument [2]. The [null] argument has been passed, while the argument of type matching string expression "boolean" is expected.
Invalid argument [1]. The [null] <<falsy>> argument has been passed, while the argument of type matching string expression "truthy|undefined" is expected.
Invalid argument [0]. The [undefined] argument has been passed, while the argument of type matching regular expression /string/i is expected.
callback
function.throw
statement!callback
function is executed only if at least one argument passed through the enclosing function is of invalid type.callback
function with the following properties:
index
0
, 1
actual
"String"
expected
"Array"
, "Boolean|Number"
, "/array|object/i"
message
textActual
"[undefined] <<falsy>> argument"
textExpected
"argument of type matching regular expression /String|null/"
const type = require('typeof-arguments');
hello('Nikola', 26);
function hello(name, age) {
type(arguments, [String, 'string|number'], (o) => {
console.error(o.message);
/*
console.error('Not good! Use ' + o.expected + ' instead of ' + o.actual + ' for argument ' + o.index);
throw new Error("Aborted! " + o.message);
*/
});
}
The function type()
returns true
when all arguments passed through the enclosing function are of valid types.
The function type()
returns false
when at least one of the arguments passed through the enclosing function is of invalid type.
const type = require('typeof-arguments');
hello('hello', 'world!');
function hello(paramA, paramB) {
const valid = type(arguments, ['string', 'string'], () => { });
if (!valid) return; //stop executing code if at least one argument is of invalid type
//your code here...
}
const type = require('typeof-arguments');
function test(paramA, paramB, paramC) {
type(arguments, ['number|string', 'any', 'null|array']);
}
test('hello', 'it\'s me!', null);
//no errors
test(10, 20, [1, 2, 3]);
//no errors
test(true, 20, null);
//Invalid argument [0]. The [Boolean] argument has been passed, while the argument of type matching string expression "number|string" is expected.
test({ name: 'Nikola' }, false, /test/);
//Invalid argument [0]. The [Object] argument has been passed, while the argument of type matching string expression "number|string" is expected.
//Invalid argument [2]. The [RegExp] argument has been passed, while the argument of type matching string expression "null|array" is expected.
test(10, 20, null, 30, 40, 50, 60, 70);
//no errors
test(10);
//Invalid argument [2]. The [undefined] argument has been passed, while the argument of type matching string expression "null|array" is expected.
const type = require('typeof-arguments');
function test(paramA, paramB) {
type(arguments, ['truthy|string', /(regexp|falsy)/i]);
}
test();
//Invalid argument [0]. The [undefined] <<falsy>> argument has been passed, while the argument of type matching string expression "truthy" is expected.
test('', '');
//Invalid argument [0]. The [String] <<falsy>> argument has been passed, while the argument of type matching string expression "truthy" is expected.
test(1, 0);
//no errors
test(0, 1);
//Invalid argument [0]. The [Number] <<falsy>> argument has been passed, while the argument of type matching string expression "truthy" is expected.
//Invalid argument [1]. The [Number] <<truthy>> argument has been passed, while the argument of type matching regular expression /(regexp|falsy)/i is expected.
test([1, 2, 3], /test/);
//no errors
test('hello', null);
//no errors
const type = require('typeof-arguments');
function test(paramA, paramB) {
type(arguments, [String, 'any', 'any', Number, /((syntax|type)error)|falsy/i]);
}
test();
//Invalid argument [0]. The [undefined] argument has been passed, while the argument of type [String] is expected.
test('Nikola', null, false, 10);
//no errors
test('Nikola', null, false, 10, new TypeError('error'));
//no errors
test('Nikola', null, false, 10, false);
//no errors
test('Nikola');
//Invalid argument [3]. The [undefined] argument has been passed, while the argument of type [Number] is expected.
test('Nikola', true, true, 10, new Error('error'));
//Invalid argument [4]. The [Error] <<truthy>> argument has been passed, while the argument of type matching regular expression /((syntax|type)error)|falsy/i is expected.
const type = require('typeof-arguments');
function test(paramA, paramB) {
type(arguments, ['instance', 'Name', 'object', 'falsy']);
}
class Name{}
class Age{}
const name = new Name();
const age = new Age();
test();
//Invalid argument [0]. The [undefined] argument has been passed, while the argument of type matching string expression "instance" is expected.
test(name, name, {}, null);
//no errors
test(age, name, {}, NaN);
//no errors
test(age, age, {}, false);
//Invalid argument [1]. The [Age] argument has been passed, while the argument of type matching string expression "Name" is expected.
test({}, name, {}, NaN);
//Invalid argument [0]. The [Object] argument has been passed, while the argument of type matching string expression "instance" is expected.
test(name, {}, {}, 0);
//Invalid argument [1]. The [Object] argument has been passed, while the argument of type matching string expression "Name" is expected.
test(age, name, age, NaN);
//Invalid argument [2]. The [Age] argument has been passed, while the argument of type matching string expression "object" is expected.
FAQs
Validate the type of arguments passed through the function.
We found that typeof-arguments demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.