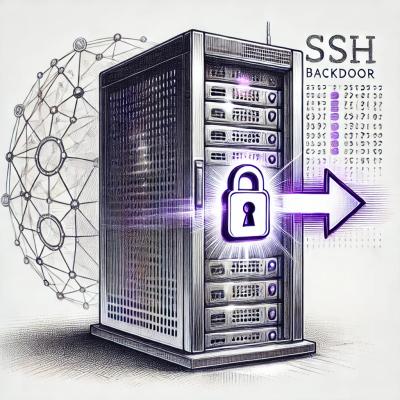
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
typescript-event-emitter
Advanced tools
Versatile and feature-rich TypeScript library for event management, providing a solid foundation for building event-driven applications in TypeScript.
Versatile and feature-rich TypeScript library for event management, providing a solid foundation for building event-driven applications in TypeScript.
* | Version | Supported |
---|---|---|
npm | >= 7.24.0 | :white_check_mark: |
$ npm install --save typescript-event-emitter
After installation, the only thing you need to do is require the module:
import { EventEmitter } from 'typescript-event-emitter';
or
const { EventEmitter } = require('typescript-event-emitter');
And you're ready to create your own EventEmitter instances.
const emitter = new EventEmitter();
let context = { test: 'Some metada' }
const onEventNameEmitted = (eventname:string, data:any) => {
console.log(data === context) // true
console.log(eventname === 'event-name') // true
};
emitter.on('event-name', onEventNameEmitted); // adds listener
emitter.emit('event-name', context); // emits listener
const emitter = new EventEmitter();
const onEventNameEmitted = (eventname:string, data:any) => {
console.log(eventname,data )
};
emitter.on('event-name', onEventNameEmitted);// adds listener
emitter.off('event-name', onEventNameEmitted); // removes listener
First emit:
Second emit (within the 100-millisecond throttle delay):
Third emit (within the 100-millisecond throttle delay):
const emitter = new EventEmitter();
let callCount = 0;
emitter.on(
'throttleEvent',
() => {
callCount++;
},
{ throttle: 100 }
);
emitter.emit('throttleEvent');
emitter.emit('throttleEvent');
emitter.emit('throttleEvent');
First emit:
Second emit (within the debounce delay):
Third emit (within the debounce delay):
So basically for the given example, the listener will be executed after 300 millisecond delay
const emitter = new EventEmitter();
let callCount = 0;
emitter.on(
'debounceEvent',
() => {
callCount++;
},
{ debounce: 100 }
);
emitter.emit('debounceEvent');
emitter.emit('debounceEvent');
emitter.emit('debounceEvent');
const emitter = new EventEmitter();
emitter.on('*', () => { // listener will be executed for both emits, wildcard listens to anything
console.log("Executed")
});
emitter.emit('someEvent');
emitter.emit('namespace.someEvent');
const emitter = new EventEmitter();
emitter.on('namespace1.*', () => { // listener will be executed 2 times, wildcard for namespace listens to anything within that namespace
console.log("Executed 1")
});
emitter.on('namespace2.*', () => { // listener will not be executed
console.log("Executed 2")
});
emitter.emit('other.event1');
emitter.emit('namespace1.event1');
emitter.emit('namespace1.event2');
const emitter = new EventEmitter();
emitter.on('*.someEvent', () => { // wildcard listeners as namespace for event
console.log("Executed")
});
emitter.emit('other.event1'); // No match, no listener executed
emitter.emit('other.someEvent'); // Matches the pattern, listener executed
emitter.emit('namespace1.event1'); // No match, no listener executed
emitter.emit('namespace1.someEvent'); // Matches the pattern, listener executed
const emitter = new EventEmitter();
emitter.on('someEvent', () => {
console.log('Listener');
});
emitter.on('namespace.someEvent', () => {
console.log('Listener');
});
emitter.emit('other.event'); // No match, no listener executed
emitter.emit('namespace.someEvent'); // Matches the pattern, listener executed
emitter.emit('namespace.event'); // No match, no listener executed
const emitter = new EventEmitter();
//last to be executed
emitter.on('priorityEvent', () => {
console.log('Low Priority Listener');
});
//first to be executed
emitter.on(
'priorityEvent',
() => {
console.log('High Priority Listener');
},
{ priority: 2 }
);
//second to be executed
emitter.on(
'priorityEvent',
() => {
console.log('Medium Priority Listener');
},
{ priority: 1 }
);
emitter.emit('priorityEvent');
interface Message {
id: number;
content: string;
messageType: string;
sender: string;
}
const emitter = new EventEmitter();
const currentUser = { username: 'example_user' };
const notificationFilter: EventFilter = (eventName, namespace) => {
if (namespace === 'dm') {
return true;
}
if (eventName === 'notification') {
return true;
}
if (namespace === 'mention' && currentUser.username === eventName) {
return true;
}
return false;
};
const receivedNotifications: Message[] = [];
emitter.on(
'*',
(_event, message: Message) => {
receivedNotifications.push(message); //array will have: directMessage, generalNotification, mentionNotification objects
},
{ filter: notificationFilter }
);
const directMessage: Message = { id: 1, content: 'Hello!', messageType: 'dm', sender: 'user123' };
const generalNotification: Message = {
id: 2,
content: 'General update',
messageType: 'announcement',
sender: 'system'
};
const mentionNotification: Message = {
id: 3,
content: 'You were mentioned!',
messageType: 'mention',
sender: 'other_user'
};
const unrelatedEvent: Message = { id: 4, content: 'Irrelevant event', messageType: 'other', sender: 'unknown' };
emitter.emit('other.event', unrelatedEvent),
emitter.emit('notification', generalNotification),
emitter.emit('dm.newMessage', directMessage),
emitter.emit('mention.example_user', mentionNotification)
const emitter = new EventEmitter();
let flag = false;
emitter.on('asyncEvent', async () => {
return new Promise<void>(resolve => {
setTimeout(() => {
flag = true;
resolve();
}, 100);
});
});
await emitter.emit('asyncEvent');
console.log(flag); // will be true
const emitter = new EventEmitter();
emitter.on('errorEvent', () => {
throw new Error('Listener Error');
});
try {
await emitter.emit('errorEvent');
} catch (error) {
console.log(error) // will be 'Listener Error'
}
const emitter = new EventEmitter();
let firstListenerInvoked = false;
let secondListenerInvoked = false;
emitter.on('errorEvent', () => {
throw new Error('Listener Error');
});
emitter.on('errorEvent', () => {
firstListenerInvoked = true;
});
emitter.on('errorEvent', () => {
secondListenerInvoked = true;
});
emitter.emit('errorEvent'); //all 3 will be fired and event flow won't be disrupted
The global event bus is a singleton that contains an instance of an event emitter. Functionality/features, etc is just a centralized mechanism for communication across different parts of an application.
import { globalEventBus } from 'typescript-event-emitter';
or
const { globalEventBus } = require('typescript-event-emitter');
let context = { test: 'Some metada' }
const onEventNameEmitted = (eventname:string, data:any) => {
console.log(data === context) // true
console.log(eventname === 'event-name') // true
};
globalEventBus.on('event-name', onEventNameEmitted); // adds listener
globalEventBus.emit('event-name', context); // emits listener
const eventEmitter: EventEmitter = new EventEmitter({ separator: ':' }); // setting global separator if not provided it will revert to default "."
eventEmitter.on("namespace:someEvent", () => {});
const eventEmitter: EventEmitter = new EventEmitter(); // default separator '.'
eventEmitter.setGlobalOptions({ separator: "-" }); // sets global separator which can be provided via constructor aswell
eventEmitter.on("namespace-someEvent", () => {});
eventEmitter.off("namespace-someEvent");
eventEmitter.on("namespace:someEvent1", () => {}, { separator: ":" }); // listener separator will be ':'
eventEmitter.off("namespace:someEvent1");
eventEmitter.on("namespace:someEvent2", () => {}, { separator: ":" }); // listener separator will be ':'
eventEmitter.on("namespace-someEvent3", () => {}); // listener separator will be '-' as it was set via setGlobalOptions
eventEmitter.off("namespace:someEvent2");
eventEmitter.off("namespace-someEvent3");
const eventEmitter: EventEmitter = new EventEmitter({ separator: ':' }); // setting global separator if not provided it will revert to default "."
globalEventBus.setGlobalOptions({ separator: "-" }); // sets global separator
globalEventBus.on("namespace:someEvent", () => {}, { separator: ":" }); // listener separator will be ':'
This module is well-tested. You can run:
npm run test
to run the tests under Node.js.
npm run test:nyc
to run the tests under Node.js and get the coverage
Tests are not included in the npm package. If you want to play with them, you must clone the GitHub repository.
Please read our Contribution Guidelines before contributing to this project.
Please read our SECURITY REPORTS
2023-12-12, version 2.0.1
FAQs
Versatile and feature-rich TypeScript library for event management, providing a solid foundation for building event-driven applications in TypeScript.
The npm package typescript-event-emitter receives a total of 8 weekly downloads. As such, typescript-event-emitter popularity was classified as not popular.
We found that typescript-event-emitter demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.