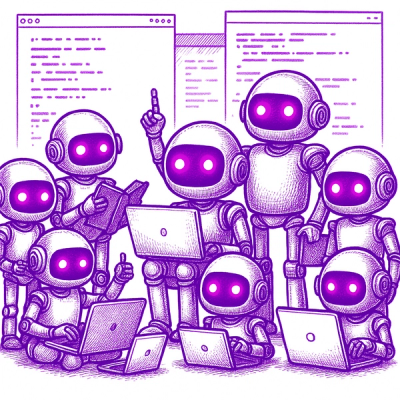
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
typescript-object-utils
Advanced tools
Immutable object manipulation methods
Library can be installed via npm.
$ npm install typescript-object-utils
import {shallowEquals} from "typescript-object-utils";
shallowEquals({a: 1, b: 2}, {a: 1, b: 2});
//=> true
import {merge} from "typescript-object-utils";
const x = {a: 1, b: 2};
const y = {b: 2, c: 3};
const r = merge(x, y); r;
//=> { a: 1, b: 2, c: 3 }
const s = merge(x, x); s;
//=> {a: 1, b: 2}
x === s;
//=> false
import {shallowMerge} from "typescript-object-utils";
const x = {a: 1, b: 2, c: 3};
const y = {c: 5, d: 4};
const z = {a: 1, b: 2};
const r = shallowMerge(x, y); r;
//=> { a: 1, b: 2, c: 5, d: 4 }
const s = shallowMerge(x, x); s;
//=> { a: 1, b: 2, c: 3 }
x === s;
//=> true
const t = shallowMerge(x, z); t;
//=> { a: 1, b: 2, c: 3 }
x === t;
//=> true
import {mergeDeep} from "typescript-object-utils";
const x = {a: 1, b: {c: 3}};
const y = {b: {c: 3, d: 4}};
const z = {b: {c: 3}};
const r = mergeDeep(x, y); r;
//=> { a: 1, b: { c: 3, d: 4 } }
const s = mergeDeep(x, z); s;
//=> { a: 1, b: { c: 3 } }
x === s;
//=> false
import {shallowMergeDeep} from "typescript-object-utils";
const x = {a: 1, b: {c: 3}};
const y = {b: {c: 3, d: 4}};
const z = {b: {c: 3}};
const r = shallowMergeDeep(x, y); r;
//=> { a: 1, b: { c: 3, d: 4 } }
const s = shallowMergeDeep(x, z); s;
//=> { a: 1, b: { c: 3 } }
x === s;
//=> true
typescript-object-utils
use ES6 features, for ES5 compatibility use es6-shim
npm install --save es6-shim @types/es6-shim
and import in main file
// index.ts
require "es6-shim"
FAQs
Immutable object manipulation methods
The npm package typescript-object-utils receives a total of 348 weekly downloads. As such, typescript-object-utils popularity was classified as not popular.
We found that typescript-object-utils demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.