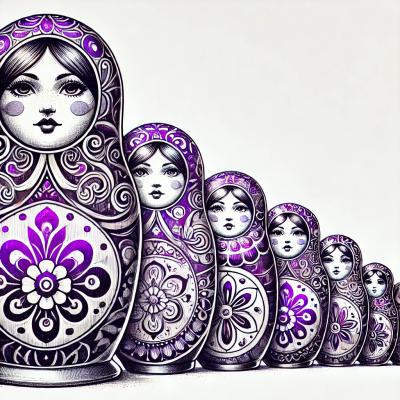
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Simple and lightweight JavaScript library for background parallax with support for picture/img elements.
Simple and lightweight JavaScript library for modern background parallax,
with support for picture elements and any images.
You can install the library via npm/yarn:
npm install ukiyojs
yarn add ukiyojs
or via CDN:
<script src="https://cdn.jsdelivr.net/npm/ukiyojs@1.0.1/dist/ukiyo.min.js"></script>
Import Ukiyo.js:
import Ukiyo from "ukiyojs";
Give the element you want to parallax a cool name to call it in JavaScript.
<img>
element<img class="ukiyo" src="image.jpg">
<picture>
element<picture>
<source srcset="img.webp" type="image/webp">
<img class="ukiyo" src="img.png">
<picture>
<picture>
<source srcset="~" media="(min-width: 1000px)" />
<source srcset="~" media="(min-width: 700px)" />
<img class="ukiyo" src="~">
</picture>
picture
tag element is also supported: set the parallax to the img
tag inside the picture tag.
<div class="ukiyo"></div>
Don't forget to set the
background-image
to the style when you set it in the img tag element.
const image = document.querySelector('.ukiyo');
new Ukiyo(image)
You are now ready to go.
If you want to apply it to more than one element, you need to loop through them as follows:
const images = document.querySelectorAll(".ukiyo");
// You can do the loop in any way you like.
images.forEach(image => {
new Ukiyo(image, {
speed: 2,
scale: 1.25
});
});
Option | Type | Default | Description |
---|---|---|---|
scale | number | 1.5 | Parallax image scaling factor. |
speed | number | 1.5 | Parallax speed. |
willChange | boolean | false | If true, the element will be given a will-change: transform when Parallax is active. |
wrapperClass | string | null | Class name of the automatically generated wrapper element. |
These can be configured with the following JS code:
const parallax = document.querySelector('.image');
new BgParallax(parallax, {
scale: 1.5, // 1~2 is recommended
speed: 1.5, // 1~2 is recommended
willChange: true, // This may not be valid in all cases
wrapperClass: "ukiyo-wrapper"
})
These options can be set individually for an element using the data-u-*
attribute:
<img
data-u-scale="2"
data-u-speed="1.7"
data-u-wrapper-class="wrapper-name"
data-u-willchange
>
Option | Description |
---|---|
data-u-scale ="" | scale option. |
data-u-speed ="" | speed option. |
data-u-willchange | willChange option. Simply attach it to the element to make it valid. |
data-u-wrapper-class ="" | wrapperClass option. |
Option names start with
data-u-*
. Don't forget to prefix the option name with a "u", if u do.
reset()
Reset the instance and recalculate the size and position of the elements etc :
const image = document.querySelector('.image');
const instance = new Ukiyo(image);
instance.reset();
destroy()
Destroy instance:
const image = document.querySelector('.image');
const instance = new Ukiyo(image);
instance.destroy();
IE | Edge | Firefox | Chrome | Opera | Safari | iOS Safari |
---|---|---|---|---|---|---|
❌No Support | ✅Latest | ✅Latest | ✅Latest | ✅Latest | ✅Latest | ✅Latest |
To support older browsers such as IE, you will need to use the Intersection Observer API, Promise
and closest()
polyfills.
If you use img
tag, you will also need to use the object-fit
polyfill.
However, please note that even with polyfill, the Parallax animation does not run smoothly in IE.
FAQs
⛰️ Dynamic, modern, and efficient background parallax effect.
We found that ukiyojs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.