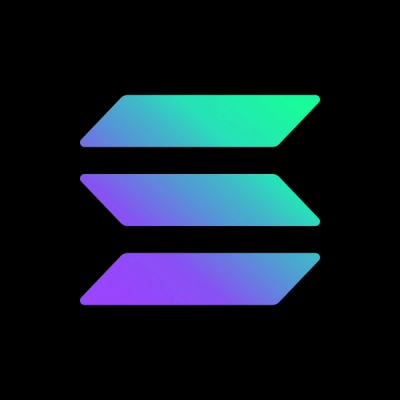
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
UltraX Package is a unique package that allows you to create cool things using simple functions and make it faster, for example there is an invite logger, discord buttons paginator, etc...
UltraX Package is a unique package made by UltraX that allows you to create cool things using simple functions and make it faster, for example there is an invite logger, discord buttons paginator, etc...
To install UltraX package you need:
You need to install Node.js.
You need a package called discord.js.
You need a package called node-fetch to use the Bin
function.
You need a package called canvas to use the welcomeImage
.
Then you can open your application's terminal and type:
$ npm install ultrax
The available functions are:
sleep
- Functions that creates timeout easily and fast.
passGen
- Function to create passwords made by letters and numbers randomly with specified length.
bin
- Function that allows you to bin codes.
ButtonPaginator
- Function to create embed pages using buttons easily.
welcomeImage
- Function that creates a welcome image fully customizable using canvas.
Events:
There is currently only one event called inviteJoin
in the package which allows you to get some informations about the invite such as the inviter, etc...
sleep is a simple function, where is make it easier and faster to make a timeout in your code.
Example:
// Defining the package
const ultrax = require('ultrax')
// Getting the sleep function from the package
const sleep = ultrax.sleep
// this will log "Start!" once i run the application
console.log('Start!')
// using the package sleep function to set a timeout
sleep(5000) // sleep(milliseconds)
// after the 5 seconds (5000 milliseconds) it will log "5 Seconds passed"
console.log('5 Seconds passed')
this is a simple function that can generate passwords using letters and numbers and the password length is custom so you can change it to any length you want!
Example:
// Defining the package
const ultrax = require('ultrax')
// getting the passGen function from the package
const passGen = ultrax.passGen
// this will log the randomly generate password in your terminal
console.log(passGen(6)) // passGen(6) that mean it will generate a password from 6 characters.
// you can change it by changing passGen(here will be the length of the password)
This is a way to bin a code.
Example:
// Defining the package
const ultrax = require('ultrax')
// if it's empty it returns a message saying "what do you want to bin?"
if (!args.join(' ')) return message.channel.send('What do you want to bin?');
else {
// else if everything works fine, we will make a new varible called "bin"
// it will be used to bin the args.join(' ') also known as the message.content
const bin = await ultrax.bin(args.join(' '));
// then here we will send the results!
console.log('Here i binned the code ' + bin)
}
Button Paginator function allows you to create embed pages easily and fast.
styles are:
red
grey
blurple
green
url
Example:
// Defining the package
const ultrax = require('ultrax')
// getting the MessageEmbed from discord.js
const { MessageEmbed } = require("discord.js")
// creating embed1
const embed1 = new MessageEmbed()
.setTitle("1st page embed");
// creating embed2
const embed2 = new MessageEmbed()
.setTitle("2nd page embed");
// creating embed3
const embed3 = new MessageEmbed()
.setTitle("3rd page embed");
// creating embed4
const embed4 = new MessageEmbed()
.setTitle("4th page embed");
// creating the buttons pages
await ultrax.ButtonPaginator(message, [embed1, embed2, embed3, embed4], [{
style: 'green',
label: 'back',
id: 'back' // don't change this line
},
{
style: 'blurple',
label: 'next',
id: 'next' // don't change this line
}
]);
This function is used to create a welcome image using canvas, fully customizable and fast!
The function returns a Promise to Buffer the image and make it an Attachment, so you need to await it.
await welcomeImage()
As we mentioned before its fully customizable, so lets see the parameters and the options available.
Parameters:
Options:
font
{default: "San Serif"}attachmentName
{default: "welcome"}So now lets talk about the correct Syntax for it!
await welcomeImage(background, avatar, text_1, text_2, text_3, color)
The background must be a PNG image's URL.
As example this URL:
https://cdn.discordapp.com/attachments/850808002545319957/859359637106065408/bg.png
The color must be a HEX color's code.
As example this HEX:
#FFFFFF
await welcomeImage(background, avatar, text_1, text_2, text_3, color, {font: "Your Font", attachmentName: 'Hello'})
the
attachmentName
is always PNG, so whatever name you will put it will end with.png
as exampleHello.png
To use custom fonts, you need to install the font (ttf/otf) yourself and use
registerFont()
to register the font and be able to use it. Example:
// Getting registerFont() from canvas
const { registerFont } = require('canvas')
// Registering the font
registerFont('ShadowsIntoLight-Regular.ttf', { family: "Shadows Into Light" });
After all these explanations and examples, lets see a full and nice welcome image function working inside of an guildMemberAdd
event!
Example:
// defining the package
const ultrax = require('ultrax')
// Getting registerFont() from canvas
const { registerFont } = require('canvas')
// Registering the custom font
registerFont('ShadowsIntoLight-Regular.ttf', { family: "Shadows Into Light" });
// Event
Client.on('guildMemberAdd', async member => {
// defining the background as bg
let bg = 'https://cdn.discordapp.com/attachments/850808002545319957/859359637106065408/bg.png'
// defining the member's avatar with "PNG" as format.
let avatar = member.user.displayAvatarURL({ format: "png" })
// defining text_1 (title)
let text1 = "welcome"
// defining text_2 (subtitle)
let text2 = member.user.tag
// defining text_3 (footer)
let text3 = `You're the ${member.guild.memberCount}th member`
// defining the color, here its white
let color = '#ffffff'
// defining the options and setting them
const options = {
font: "Shadows Into Light",
attachmentName: `welcome-${member.id}`
}
// creating the image
const image = await ultrax.welcomeImage(bg, avatar, text1, text2, text3, color, options)
// sending the image:
//channel.send(image)
})
This event is for logging invite uses. This is same as guildMemberAdd
event but this is custom event which has 3 parameters:
member
invite
inviter
Example:
// Defining the package
const ultrax = require("ultrax")
//defining discord
const discord = require('discord.js')
// new discord client
const client = new discord.Client()
// To Get The new event working we need to initilize it by:
ultrax.inviteLogger(client) // put here your discord client
// without above code event won't trigger.
// now below event will work
client.on('inviteJoin', (member, invite, inviter) => {
// results
console.log(`${member.user.tag} joined using invite code ${invite.code} from ${inviter.tag}. Invite was used ${invite.uses} times since its creation.`)
});
In case you have idea's to improve the package, or maybe you found some bugs or you need help, you can contact us from our discord server!
FAQs
UltraX Package is a unique package that allows you to create cool things using simple functions and events.
The npm package ultrax receives a total of 32 weekly downloads. As such, ultrax popularity was classified as not popular.
We found that ultrax demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.