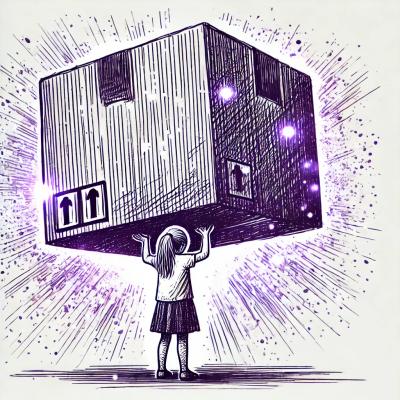
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
umi-plugin-mobx
Advanced tools
😍 use mobx-state-tree
with umi gracefully.
umi/dynamic
.umi-plugin-dva
, so you just export a state tree node by default.yarn add umi-plugin-mobx
Add plugin to .umirc.js
file, to ignore the model folders which are named stores
or other custom name, you need to install umi-plugin-routes
to tell umi
to ignore them.
// .umirc.js
export default {
plugins: [
['umi-plugin-mobx', {
modelName: 'store', // or "stores", defaults to "store", you can set "model" like dva.
exclude: [/^\$/, (filename) => filename.includes('__')]
}],
['umi-plugin-routes', {
exclude: [/stores/] // ignore **/stores/**/*.*, you can set /models/ like dva.
}]
]
}
yarn add umi-plugin-routes
[Deprecated] You can also just use page.jsx
or page.tsx
to skip umijs
dirctory resolving.
interface PluginOptions {
modelName?: string;
exclude?: Excludes;
}
type Excludes = (RegExp | TestFn)[];
type TestFn = (filename: string) => boolean;
Mobx config documents
// src/mobx.ts
// or src/mobx.js
export function config() {
return {
enforceActions: true // or 'strict' for strict-mode
};
}
Create a state-tree node.
// src/stores/user.ts
import { types, flow } from 'mobx-state-tree';
export const Item = types
.model('item', {
key: types.string,
content: types.string
});
type ItemSnapshotType = typeof Item.SnapshotType;
const User = types
.model({
firstName: types.string,
lastName: types.string,
age: types.number,
list: types.array(Item)
})
.views((self) => ({
get name() {
return self.firstName + ' ' + self.lastName;
}
}))
.volatile((self) => ({
uid: 0
}))
.actions((self) => ({
changeFirstName(str: string) {
self.firstName = str;
},
addListItemAsync: flow(function* addListItemAsync() {
const currentUid = self.uid++;
const item: ItemSnapshotType = yield new Promise<ItemSnapshotType>((resolve) => setTimeout(() => {
resolve({
key: 'item-' + currentUid,
content: 'this is content...'
});
}, 1000));
self.list.push(Item.create(item));
})
}))
export type UserType = typeof User.Type;
/**
* YOU SHOULD EXPORT A STATE TREE NODE BY DEFAULT!
**/
export default User.create({
firstName: 'Heskey',
lastName: 'Baozi',
age: 20,
list: []
});
Create an observer and inject the state-tree node.
// src/pages/about.tsx
import * as React from 'react';
import { observer, inject } from 'mobx-react';
import { UserType } from '../stores/user';
import { observable, action, computed } from 'mobx';
interface AboutProps {
user?: UserType;
}
@inject('user')
@observer
export default class About extends React.Component<AboutProps> {
@observable
count = 15;
@action
handleChangeInput: React.ChangeEventHandler<HTMLInputElement> = (e) => {
this.props.user!.changeFirstName(e.currentTarget.value)
}
@action
up = () => {
console.log('click to ', this.count);
this.count++;
}
handleClickAddItem: React.MouseEventHandler<HTMLButtonElement> = async () => {
const { user } = this.props;
await user!.addListItemAsync();
console.log('add, uid = ', user!.uid);
}
@computed
get List() {
const { user } = this.props;
return user!.list.map((item) => (
<li key={ item.key }>
[{ item.key }]: { item.content }
</li>
));
}
render() {
return (
<div>
<h1>About</h1>
<p>Name: { this.props.user!.name }</p>
<input type="text" value={ this.props.user!.firstName } onChange={ this.handleChangeInput } />
<p>count: { this.count }</p>
<button onClick={ this.up }>count++</button>
<p>List: <button onClick={ this.handleClickAddItem }>Add Item</button></p>
<ul>
{ this.List }
</ul>
</div>
);
}
}
FAQs
Use mobx-state-tree gracefully with umi.
The npm package umi-plugin-mobx receives a total of 1 weekly downloads. As such, umi-plugin-mobx popularity was classified as not popular.
We found that umi-plugin-mobx demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.