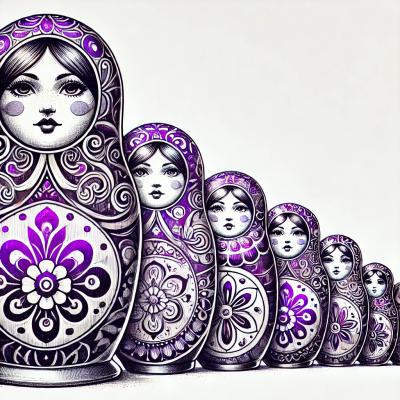
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
The 'unfetch' npm package is a lightweight polyfill for the Fetch API, which allows you to make HTTP requests in environments where the Fetch API is not natively available, such as older browsers or certain JavaScript environments. It is designed to be minimal and efficient, providing the essential functionality of the Fetch API without any additional overhead.
Basic Fetch Request
This feature demonstrates how to make a basic HTTP GET request using 'unfetch'. The response is then converted to JSON and logged to the console.
const fetch = require('unfetch');
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
POST Request
This feature demonstrates how to make an HTTP POST request using 'unfetch'. The request includes headers and a JSON body, and the response is processed similarly to the GET request.
const fetch = require('unfetch');
fetch('https://api.example.com/data', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ key: 'value' })
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Handling Errors
This feature demonstrates how to handle errors in a fetch request using 'unfetch'. It checks if the response is not ok and throws an error, which is then caught and logged.
const fetch = require('unfetch');
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error('Fetch error:', error));
'node-fetch' is a popular package that provides a Fetch API implementation for Node.js environments. It is more feature-rich compared to 'unfetch' and supports additional functionalities like streaming and handling of complex request/response scenarios.
'axios' is a promise-based HTTP client for the browser and Node.js. It offers a more comprehensive set of features compared to 'unfetch', including request and response interceptors, automatic JSON data transformation, and support for canceling requests.
'isomorphic-fetch' is a package that brings the Fetch API to both client and server environments, making it suitable for universal (isomorphic) JavaScript applications. It is similar to 'unfetch' but aims to provide a consistent API across different environments.
Tiny 500b fetch "barely-polyfill"
fetch()
with headers and text/json/xml responses🤔 What's Missing?
- Uses simple Arrays instead of Iterables, since Arrays are iterables
- No streaming, just Promisifies existing XMLHttpRequest response bodies
- Bare-bones
.blob()
implementation - just proxiesxhr.response
This project uses node and npm. Go check them out if you don't have them locally installed.
$ npm install --save unfetch
Then with a module bundler like rollup or webpack, use as you would anything else:
// using ES6 modules
import fetch from 'unfetch'
// using CommonJS modules
var fetch = require('unfetch')
The UMD build is also available on unpkg:
<script src="https://unpkg.com/unfetch/dist/unfetch.umd.js"></script>
This exposes a fetch
global if not already present.
import fetch from 'unfetch'
fetch('/foo.json')
.then( r => r.json() )
.then( data => {
console.log(data)
})
Coming soon!
First off, thanks for taking the time to contribute! Now, take a moment to be sure your contributions make sense to everyone else.
Found a problem? Want a new feature? First of all see if your issue or idea has already been reported. If don't, just open a new clear and descriptive issue.
Pull requests are the greatest contributions, so be sure they are focused in scope, and do avoid unrelated commits.
git clone https://github.com/<your-username>/unfetch
cd unfetch
git checkout -b my-new-feature
npm install
git commit -am 'Add some feature'
git push origin my-new-feature
FAQs
Bare minimum fetch polyfill in 500 bytes
The npm package unfetch receives a total of 2,824,078 weekly downloads. As such, unfetch popularity was classified as popular.
We found that unfetch demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.