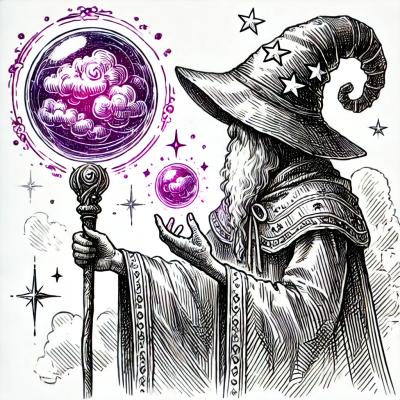
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Upcast is a low-level JavaScript type checking and casting library. Upcast simplifies type-checking and converts between types in a more sensible and predictable way than using plain ol' JavaScript.
Current Version: 1.0.4
Automated Build Status:
Node Support: 0.6, 0.8, 0.10
Browser Support: Android Browser 2.2–4.2, Firefox 3.6, Firefox 4–19, Google Chrome 14–25, Internet Explorer 6–10, Mobile Safari iOS 3–6, Opera 12.10, Safari 5–6
You can use Upcast on the server side with Node.js and npm:
$ npm install upcast
On the client side, you can either install Upcast through Bower/Component:
$ bower install upcast
$ component install rowanmanning/upcast
or by simply including upcast.js
in your page:
<script src="path/to/lib/upcast.js"></script>
In Node.js or using Component, you can include Upcast in your script by using require:
var upcast = require('upcast');
Upcast also works with AMD-style module loaders, just specify it as a dependency.
If you're just including with a <script>
, upcast
is available as a global variable.
Upcast exposes three simple functions:
Get the type of an object. This accepts a single argument:
val: (mixed) The object to get the type of.
Types in Upcast are different to typeof
in what is reported for arrays and null
. See the example below:
upcast.type([]); // 'array'
upcast.type(true); // 'boolean'
upcast.type(function () {}); // 'function'
upcast.type(null); // 'null'
upcast.type(123); // 'number'
upcast.type({}); // 'object'
upcast.type('foo'); // 'string'
upcast.type(undefined); // 'undefined'
Check whether an object is of a given type. This accepts two arguments:
val: (mixed) The object to check the type of.
type: (string) The type to check for. One of array
, boolean
, function
, null
, number
, object
, string
or undefined
.
This function follows the same rules outlined in upcast.type
and allows you to use type aliases.
upcast.is('foo', 'string'); // true
upcast.is(123, 'string'); // false
upcast.is([], 'array'); // true
upcast.is([], 'object'); // false
upcast.is(null, 'null'); // true
upcast.is(null, 'object'); // false
Convert an object to a specific type. This accepts two arguments:
val: (mixed) The object to convert.
type: (string) The type to convert to. One of array
, boolean
, function
, null
, number
, object
, string
or undefined
.
The way types are converted aims to be sensible and allow easy switching back-and-forth of common types. For example, switching between strings and arrays is quite fluid:
upcast.to('foo', 'array'); // ['f', 'o', 'o']
upcast.to(['f', 'o', 'o'], 'string'); // 'foo'
You can use type aliases with this function. The examples below illustrate the way types are converted.
Converting to an array from a boolean, function, number or object simply wraps the value in an array:
upcast.to(123, 'array'); // [123]
Strings are handled differently, an array is returned with each character in the string as an item:
upcast.to('foo', 'array'); // ['f', 'o', 'o']
Null and undefined are converted to an empty array:
upcast.to(null, 'array'); // []
Boolean conversion simply converts to true
or false
based on whether the value is truthy or not. The only case where this doesn't follow JavaScript's standard behaviour is with empty arrays which are converted to false
:
upcast.to([1, 2, 3], 'boolean') // true
upcast.to([], 'boolean') // false
When converting to a function, the original value is simply wrapped in a new function. This function returns the original value:
upcast.to('foo', 'function'); // function () { return 'foo'; }
As expected, converting to null will always return null
:
upcast.to('foo', 'null'); // null
Converting to a number from a boolean, function, null or object simply calls Number
with the original value as an argument, returning the expected value:
upcast.to('true', 'number'); // 1
Arrays and strings are handled differently, an array is joined to create a string, then evaluated with parseInt
; strings are simply evaluated with parseInt
:
upcast.to([1, 2, 3], 'number'); // 123
upcast.to('123', 'number'); // 123
upcast.to('foo', 'number'); // 0
Undefined is converted to 0
rather than NaN
:
upcast.to(undefined, 'number'); // 0
Converting to an object simply calls Object
with the value as a first argument. The following are equivalent:
upcast.to('foo', 'object');
Object('foo');
Converting to a string from a boolean, function, number or object simply returns the value added to an empty string, using JavaScript's default type conversion:
upcast.to(true, 'string'); // 'true'
upcast.to(123, 'string'); // '123'
Arrays are handled differently, they are joined with an empty string:
upcast.to(['f', 'o', 'o'], 'string'); // 'foo'
Null and undefined are converted to an empty string rather than 'null'
and 'undefined'
:
upcast.to(null, 'string'); // ''
As expected, converting to undefined will always return undefined
:
upcast.to('foo', 'undefined'); // undefined
The is
and to
functions allow you to use aliases to certain core types. The following are equivalent:
upcast.is([], 'array');
upcast.is([], 'arr');
upcast.is([], 'a');
The aliases available by default are:
arr
, a
bool
, b
fn
, f
num
, n
obj
, o
str
, s
To develop Upcast, you'll need to clone the repo and install dependencies with make deps
. If you're on Windows, you'll also need to install Make for Windows.
Once you're set up, you can run the following commands:
$ make deps # Install dependencies
$ make lint # Run JSHint with the correct config
$ make test # Run unit tests in Node
$ make test-server # Run a server for browser unit testing (visit localhost:3000)
When no build target is specified, make will run deps lint test
. This means you can use the following command for brevity:
$ make
Code with lint errors or no/failing tests will not be accepted, please use the build tools outlined above.
Upcast is licensed under the MIT license.
FAQs
Upcast is a low-level JavaScript type checking and casting library
The npm package upcast receives a total of 10,445 weekly downloads. As such, upcast popularity was classified as popular.
We found that upcast demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.