File & Image Uploader
(With Integrated Cloud Storage)
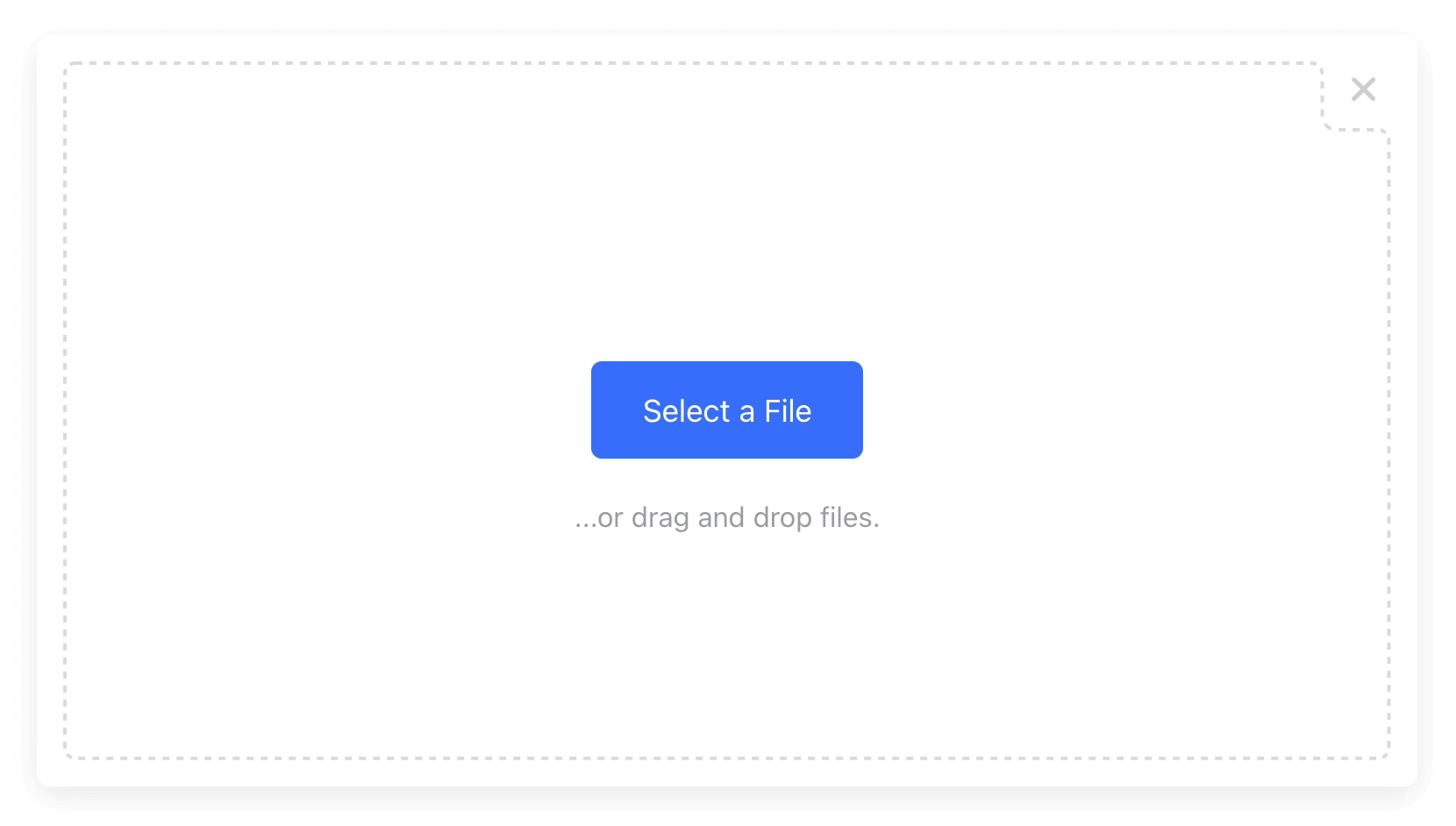
Supports: single & multi-file uploads, modal & inline views, localization, mobile, and more...
Installation
Install via NPM:
npm install uploader
Or via YARN:
yarn add uploader
Or via a <script>
tag:
<script src="https://js.upload.io/uploader/v1"></script>
Usage
Initialize
Initialize once at the start of your application:
const { Uploader } = require("uploader");
const uploader = new Uploader({
apiKey: "free"
});
Open the Modal
With JavaScript β Try on CodePen:
uploader.open({ multi: true }).then(files => {
if (files.length === 0) {
console.log('No files selected.')
} else {
console.log('Files uploaded:');
console.log(files.map(f => f.fileUrl));
}
}).catch(err => {
console.error(err);
});
Or with HTML β Try on CodePen:
<button data-upload-config='{ "multi": true }'
data-upload-complete='alert(
`Files uploaded:\n${event.files.map(x => x.fileUrl).join("\n")}`
)'>
Upload Files...
</button>
Note: you still need to initialize the Uploader when using data-*
attributes.
Get the Result
With JavaScript:
.open()
returns Promise<Array<UploaderResult>>
:
{
fileUrl: "https://upcdn.io/FW25...",
editedFile: undefined,
originalFile: {
accountId: "FW251aX",
file: { ... },
fileId: "FW251aXa9ku...",
fileUrl: "https://upcdn.io/FW25...",
fileSize: 12345,
mime: "image/jpeg",
suggestedOptimization: {
transformationUrl: "https://upcdn.io/..",
transformationSlug: "thumbnail"
},
tags: [
{ name: "tag1", searchable: true },
{ name: "tag2", searchable: true },
...
]
}
}
Or with HTML:
<a data-upload-complete="console.log(JSON.stringify(event.files))">
Upload a file...
</a>
- The
data-upload-complete
attribute is fired on completion. - The
event.files
array contains the uploaded files. - The above example opens an Uploader which logs the same output as the JavaScript example.
π More Examples
Creating an Image Upload Button
With JavaScript β Try on CodePen:
uploader
.open({
multi: false,
mimeTypes: ["image/jpeg", "image/png", "image/webp"],
editor: {
images: {
cropShape: "circ",
cropRatio: 1 / 1
}
}
})
.then(files => alert(JSON.stringify(files)));
Or with HTML β Try on CodePen:
<button data-upload-complete='alert(JSON.stringify(event.files))'
data-upload-config='{
"multi": false,
"mimeTypes": ["image/jpeg", "image/png", "image/webp"],
"editor": {
"images": {
"cropShape": "circ",
"cropRatio": 1
}
}
}'>
Upload an Image...
</button>
Creating a "Single File" Upload Button
With JavaScript β Try on CodePen:
uploader.open().then(files => alert(JSON.stringify(files)));
Or with HTML β Try on CodePen:
<button data-upload-complete='alert(JSON.stringify(event.files))'>
Upload a Single File...
</button>
Creating a "Multi File" Upload Button
With JavaScript β Try on CodePen:
uploader.open({ multi: true }).then(files => alert(JSON.stringify(files)));
Or with HTML β Try on CodePen:
<button data-upload-config='{ "multi": true }'
data-upload-complete='alert(JSON.stringify(event.files))'>
Upload Multiple Files...
</button>
Creating a Dropzone
You can use Uploader as a dropzone β rather than a modal β by specifying layout: "inline"
and a container:
With JavaScript β Try on CodePen:
uploader.open({
multi: true,
layout: "inline",
container: "#example_div_id",
onUpdate: (files) => console.log(files)
})
Or with HTML β Try on CodePen:
<div data-upload-config='{ "multi": true }'
data-upload-complete="console.log(event.files)"
style="position: relative; width: 450px; height: 300px;">
</div>
Note:
- You must set
position: relative
, width
and height
on the container div
. - The
Finish
button is hidden by default in this mode (override with "showFinishButton": true
). - When using the HTML approach:
- The
container
& layout: "inline"
config options are automatically set. - The
data-upload-complete
callback is fired every time the list of uploaded files changes. - The
data-upload-finalized
callback is fired when Finish
is clicked (if visible, see comment above).
π SPA Support
Uploader is SPA-friendly β even when using data-*
attributes to render your widgets.
Uploader automatically observes the DOM for changes, making the data-upload-complete
attribute safe for SPAs that introduce elements at runtime.
Β» React Example on CodePen Β«
π API Support
Uploader is powered by Upload.io's File Upload API β an easy-to-consume API that provides:
- File uploading.
- File listing.
- File deleting.
- File access control.
- File TTL rules / expiring links.
- And more...
Uploading a "Hello World"
file is as simple as:
curl --data "Hello World" \
-u apikey:free \
-X POST "https://api.upload.io/v1/files/basic"
Note: Remember to set -H "Content-Type: mime/type"
when uploading other file types!
Read the File Upload API docs Β»
β‘ Need a Lightweight Client Library?
Uploader is built on Upload.js β the fast 7KB client library for Upload.io's File Upload API.
Use Upload.js if you already have a UI, and just need to implement file upload functionality.
Upload.js provides:
- End-to-end file upload functionality (zero config β all you need is an Upload API key, e.g.
"free"
.) - Small 7KB package size (including all dependencies).
- Progress smoothing (using a built-in exponential moving average (EMA) algorithm).
- Automatic file chunking (for large file support).
- Cancellation (for in-progress file uploads).
- And more...
Try Upload.js on CodePen Β»
βοΈ Configuration
All configuration is optional.
With JavaScript:
uploader
.open({
container: "body",
layout: "modal",
locale: myCustomLocale,
maxFileSizeBytes: 1024 ** 2,
mimeTypes: ["image/jpeg"],
multi: false,
onUpdate: files => {},
showFinishButton: true,
showRemoveButton: true,
tags: ["profile_picture"],
editor: {
images: {
crop: true,
cropRatio: 4 / 3,
cropShape: "rect"
}
},
})
.then(files => alert(files))
Or with HTML:
<button data-upload-complete='alert(event.files)'
data-upload-config='{
"container": "body",
"layout": "modal",
"multi": false
}'>
Upload a File...
</button>
π³οΈ Localization
Default is EN_US:
const myCustomLocale = {
"error!": "Error!",
"done": "Done",
"addAnotherFile": "Add another file...",
"cancel": "cancel",
"cancelled!": "cancelled",
"continue": "Continue",
"crop": "Crop",
"finish": "Finished",
"finishIcon": true,
"maxSize": "File size limit:",
"next": "Next",
"orDragDropFile": "...or drag and drop a file.",
"orDragDropFiles": "...or drag and drop files.",
"pleaseWait": "Please wait...",
"removed!": "removed",
"remove": "remove",
"skip": "Skip",
"unsupportedFileType": "File type not supported.",
"uploadFile": "Select a File",
"uploadFiles": "Select Files"
}
π· Resizing & Cropping Images
Given an uploaded image URL:
https://upcdn.io/W142hJkHhVSQ5ZQ5bfqvanQ
Resize with:
https://upcdn.io/W142hJkHhVSQ5ZQ5bfqvanQ/thumbnail
Auto-crop with:
https://upcdn.io/W142hJkHhVSQ5ZQ5bfqvanQ/thumbnail-square
π― Features
Uploader is the file & image upload widget for Upload.io: the file upload service for developers.
Core features:
- Beautifully clean UI widget.
- Single & Multi-File Uploads.
- Fluid Layout & Mobile-Friendly.
- Image Cropping.
- Localization.
- Integrated File Hosting:
- Files stored on Upload.io for 4 hours with the
"free"
API key. - Files hosted via the Upload CDN: 100 locations worldwide.
Available with an account:
- Permanent Storage.
- Unlimited Daily Uploads. (The
"free"
API key allows 100 uploads per day per IP.) - Extended CDN Coverage. (Files served from 300+ locations worldwide.)
- Upload & Download Authentication. (Supports federated auth via your own JWT authorizer.)
- File & Folder Management Console.
- Expiring Links.
- Custom CNAME.
- Advanced Upload Control:
- Rate Limiting.
- Traffic Limiting.
- File Size Limiting.
- IP Blacklisting.
- File Type Blacklisting.
- And More...
Create an Upload.io account Β»
Building From Source
Please read: BUILD.md
Contribute
If you would like to contribute to Uploader:
- Add a GitHub Star to the project (if you're feeling generous!).
- Determine whether you're raising a bug, feature request or question.
- Raise your issue or PR.
License
MIT