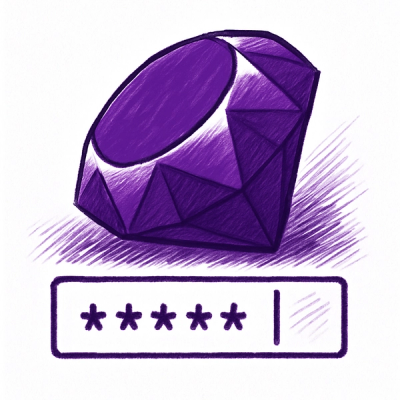
Research
/Security News
60 Malicious Ruby Gems Used in Targeted Credential Theft Campaign
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
use-deep-compare-effect
Advanced tools
It's react's useEffect hook, except using deep comparison on the inputs, not reference equality
The `use-deep-compare-effect` package is a React hook that works similarly to `useEffect`, but it performs a deep comparison of the dependencies to determine if they have changed. This is particularly useful when dealing with complex objects or arrays as dependencies, where shallow comparison would not suffice.
Deep Comparison of Dependencies
This feature allows you to use a deep comparison for the dependencies of the effect. In this example, `useDeepCompareEffect` will only re-run the effect if `complexObject` has changed deeply, not just shallowly.
import { useDeepCompareEffect } from 'use-deep-compare-effect';
function MyComponent({ complexObject }) {
useDeepCompareEffect(() => {
// Effect logic here
console.log('complexObject has changed');
}, [complexObject]);
return <div>Check the console for updates</div>;
}
The `react-use` package is a collection of essential React hooks, including `useDeepCompareEffect`. It offers a wide range of hooks for various use cases, making it a more comprehensive solution compared to `use-deep-compare-effect`, which focuses solely on deep comparison for effects.
The `use-deep-compare` package provides a hook for deep comparison of dependencies, similar to `use-deep-compare-effect`. However, it is more focused on providing a utility for deep comparison rather than being a full-fledged effect hook.
It's React's useEffect hook, except using deep comparison on the inputs, not reference equality
WARNING: Please only use this if you really can't find a way to use
React.useEffect
. There's often a better way to do what you're trying to do than a deep comparison.
React's built-in useEffect
hook has a second argument called
the "dependencies array" and it allows you to optimize when React will call your
effect callback. React will do a comparison between each of the values (via
Object.is
) to determine whether your effect callback should be
called.
The problem is that if you need to provide an object for one of those dependencies and that object is new every render, then even if none of the properties changed, your effect will get called anyway.
This module is distributed via npm which is bundled with node and should be installed as one of your project's dependencies:
npm install --save use-deep-compare-effect
You use it in place of React.useEffect
.
NOTE: Only use this if your values are objects or arrays that contain objects. Otherwise, you should just use
React.useEffect
. In case of "polymorphic" values (eg: sometimes object, sometimes a boolean), useuseDeepCompareEffectNoCheck
, but do it at your own risk, as maybe there can be better approaches to the problem.
NOTE: Be careful when your dependency is an object which contains function. If that function is defined on the object during a render, then it's changed and the effect callback will be called every render. Issue has more context.
Example:
import React from 'react'
import ReactDOM from 'react-dom'
import useDeepCompareEffect from 'use-deep-compare-effect'
function Query({query, variables}) {
// some code...
useDeepCompareEffect(
() => {
// make an HTTP request or whatever with the query and variables
// optionally return a cleanup function if necessary
},
// query is a string, but variables is an object. With the way Query is used
// in the example above, `variables` will be a new object every render.
// useDeepCompareEffect will do a deep comparison and your callback is only
// run when the variables object actually has changes.
[query, variables],
)
return <div>{/* awesome UI here */}</div>
}
Looking to contribute? Look for the Good First Issue label.
Please file an issue for bugs, missing documentation, or unexpected behavior.
Please file an issue to suggest new features. Vote on feature requests by adding a ๐. This helps maintainers prioritize what to work on.
Thanks goes to these people (emoji key):
This project follows the all-contributors specification. Contributions of any kind welcome!
MIT
FAQs
It's react's useEffect hook, except using deep comparison on the inputs, not reference equality
The npm package use-deep-compare-effect receives a total of 735,195 weekly downloads. As such, use-deep-compare-effect popularity was classified as popular.
We found that use-deep-compare-effect demonstrated a not healthy version release cadence and project activity because the last version was released a year ago.ย It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isnโt whitelisted.