useFetch
🐶 React hook for making isomorphic http requests
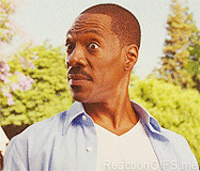
Need to fetch some data? Try this one out. It's an isomorphic fetch hook. That means it works with SSR (server side rendering).
Examples
Installation
yarn add use-http
Usage
import useFetch from 'use-http'
function App() {
const options = {
method: 'POST',
body: {},
}
var [data, loading, error] = useFetch('https://example.com', options)
var { data, loading, error } = useFetch('https://example.com', options)
if (error) {
return 'Error!'
}
if (loading) {
return 'Loading!'
}
return (
<code>
<pre>{data}</pre>
</code>
)
}
Or you can use one of the nice helper hooks. All of them accept the second options
parameter.
import { useGet, usePost, usePatch, usePut, useDelete } from 'use-http'
function App() {
const [data, loading, error] = useGet('https://example.com')
if (error) {
return 'Error!'
}
if (loading) {
return 'Loading!'
}
return (
<code>
<pre>{data}</pre>
</code>
)
}
Hooks
Option | Description |
---|
useFetch | The base hook |
useGet | Defaults to a GET request |
usePost | Defaults to a POST request |
usePut | Defaults to a PUT request |
usePatch | Defaults to a PATCH request |
useDelete | Defaults to a DELETE request |
Options
Option | Description |
---|
options | This is exactly what you would pass to the normal js fetch |