useFetch
🐶 React hook for making isomorphic http requests
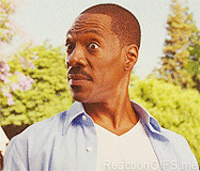
Need to fetch some data? Try this one out. It's an isomorphic fetch hook. That means it works with SSR (server side rendering).
Examples
Installation
yarn add use-http or npm i -S use-http
Usage
Basic Usage
import useFetch from 'use-http'
function Todos() {
const options = {
onMount: true
}
const todos = useFetch('https://example.com/todos', options)
const addTodo = () => {
todos.post({
title: 'no way',
})
}
if (todos.error) return 'Error!'
if (todos.loading) return 'Loading...'
return (
<>
<button onClick={addTodo}>Add Todo</button>
{todos.data.map(todo => (
<div key={todo.id}>{todo.title}</div>
)}
</>
)
}
Destructured methods
var [data, loading, error, request] = useFetch('https://example.com')
var { data, loading, error, request } = useFetch('https://example.com')
request.post({
no: 'way',
})
Relative routes
const request = useFetch({
baseUrl: 'https://example.com'
})
request.post('/todos', {
no: 'way'
})
Helper hooks
import { useGet, usePost, usePatch, usePut, useDelete } from 'use-http'
const [data, loading, error, patch] = usePatch({
url: 'https://example.com',
headers: {
'Content-type': 'application/json; charset=UTF-8'
}
})
patch({
yes: 'way',
})
Abort
const githubRepos = useFetch({
baseUrl: `https://api.github.com/search/repositories?q=`
})
const searchGithubRepos = e => githubRepos.get(encodeURI(e.target.value))
<>
<input onChange={searchGithubRepos} />
<button onClick={githubRepos.abort}>Abort</button>
{githubRepos.loading ? 'Loading...' : githubRepos.data.items.map(repo => (
<div key={repo.id}>{repo.name}</div>
))}
</>
Hooks
Option | Description |
---|
useFetch | The base hook |
useGet | Defaults to a GET request |
usePost | Defaults to a POST request |
usePut | Defaults to a PUT request |
usePatch | Defaults to a PATCH request |
useDelete | Defaults to a DELETE request |
Options
This is exactly what you would pass to the normal js fetch
, with a little extra.
Option | Description | Default |
---|
onMount | Once the component mounts, the http request will run immediately | false |
baseUrl | Allows you to set a base path so relative paths can be used for each request :) | empty string |
const {
data,
loading,
error,
request,
get,
post,
patch,
put,
delete
del,
abort,
} = useFetch({
url: 'https://example.com',
baseUrl: 'https://example.com',
onMount: true
})
or
const [data, loading, error, request] = useFetch({
url: 'https://example.com',
baseUrl: 'https://example.com',
onMount: true
})
const {
get,
post,
patch,
put,
delete
del,
abort,
} = request
Credits
use-http is heavily inspired by the popular http client axios
Feature Requests/Ideas
If you have feature requests, let's talk about them in this issue!
Todos