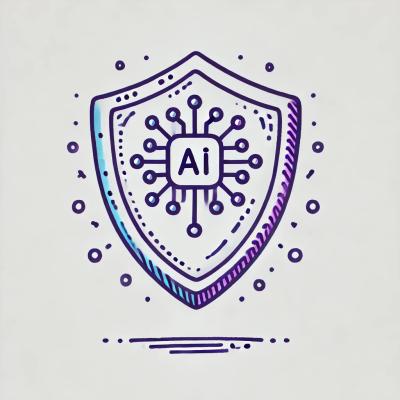
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
npm i use-http
url
and options
useFetch
import useFetch from 'use-http'
function Todos() {
const options = { // accepts all `fetch` options
onMount: true // will fire on componentDidMount
}
const todos = useFetch('https://example.com/todos', options)
function addTodo() {
todos.post({
title: 'no way',
})
}
return (
<>
<button onClick={addTodo}>Add Todo</button>
{request.error && 'Error!'}
{request.loading && 'Loading...'}
{(todos.data || []).length > 0 && todos.data.map(todo => (
<div key={todo.id}>{todo.title}</div>
)}
</>
)
}
useFetch
var [data, loading, error, request] = useFetch('https://example.com')
// want to use object destructuring? You can do that too
var { data, loading, error, request } = useFetch('https://example.com')
useFetch
⚠️ baseUrl
is no longer supported, it is now only url
var request = useFetch({ url: 'https://example.com' })
// OR
var request = useFetch('https://example.com')
request.post('/todos', {
no: 'way'
})
useGet
, usePost
, usePatch
, usePut
, useDelete
import { useGet, usePost, usePatch, usePut, useDelete } from 'use-http'
const [data, loading, error, patch] = usePatch({
url: 'https://example.com',
headers: {
'Accept': 'application/json; charset=UTF-8'
}
})
patch({
yes: 'way',
})
useFetch
const githubRepos = useFetch({
url: `https://api.github.com/search/repositories?q=`
})
// the line below is not isomorphic, but for simplicity we're using the browsers `encodeURI`
const searchGithubRepos = e => githubRepos.get(encodeURI(e.target.value))
<>
<input onChange={searchGithubRepos} />
<button onClick={githubRepos.abort}>Abort</button>
{githubRepos.loading ? 'Loading...' : githubRepos.data.items.map(repo => (
<div key={repo.id}>{repo.name}</div>
))}
</>
useFetch
const QUERY = `
query Todos($userID string!) {
todos(userID: $userID) {
id
title
}
}
`
function App() {
const request = useFetch('http://example.com')
const getTodosForUser = id => request.query(QUERY, { userID: id })
return (
<>
<button onClick={() => getTodosForUser('theUsersID')}>Get User's Todos</button>
{request.loading ? 'Loading...' : <pre>{request.data}</pre>}
</>
)
}
useFetch
The Provider
allows us to set a default url
, options
(such as headers) and so on.
const MUTATION = `
mutation CreateTodo($todoTitle string) {
todo(title: $todoTitle) {
id
title
}
}
`
function App() {
const [todoTitle, setTodoTitle] = useState('')
const request = useFetch('http://example.com')
const createtodo = () => request.mutate(MUTATION, { todoTitle })
return (
<>
<input onChange={e => setTodoTitle(e.target.value)} />
<button onClick={createTodo}>Create Todo</button>
{request.loading ? 'Loading...' : <pre>{request.data}</pre>}
</>
)
}
Provider
using the GraphQL useMutation
and useQuery
import { useQuery } from 'use-http'
export default function QueryComponent() {
// can also do it this way:
// const [data, loading, error, query] = useQuery`
// or this way:
// const { data, loading, error, query } = useQuery`
const request = useQuery`
query Todos($userID string!) {
todos(userID: $userID) {
id
title
}
}
`
const getTodosForUser = id => request.query({ userID: id })
return (
<>
<button onClick={() => getTodosForUser('theUsersID')}>Get User's Todos</button>
{request.loading ? 'Loading...' : <pre>{request.data}</pre>}
</>
)
}
import { useMutation } from 'use-http'
export default function MutationComponent() {
const [todoTitle, setTodoTitle] = useState('')
// can also do it this way:
// const request = useMutation`
// or this way:
// const { data, loading, error, mutate } = useMutation`
const [data, loading, error, mutate] = useMutation`
mutation CreateTodo($todoTitle string) {
todo(title: $todoTitle) {
id
title
}
}
`
const createTodo = () => mutate({ todoTitle })
return (
<>
<input onChange={e => setTodoTitle(e.target.value)} />
<button onClick={createTodo}>Create Todo</button>
{loading ? 'Loading...' : <pre>{data}</pre>}
</>
)
}
These props are defaults used in every request inside the <Provider />
. They can be overwritten individually
import { Provider } from 'use-http'
import QueryComponent from './QueryComponent'
import MutationComponent from './MutationComponent'
function App() {
const options = {
headers: {
Authorization: 'Bearer:asdfasdfasdfasdfasdafd'
}
}
return (
<Provider url='http://example.com' options={options}>
<QueryComponent />
<MutationComponent />
<Provider/>
)
}
Hook | Description |
---|---|
useFetch | The base hook |
useGet | Defaults to a GET request |
usePost | Defaults to a POST request |
usePut | Defaults to a PUT request |
usePatch | Defaults to a PATCH request |
useDelete | Defaults to a DELETE request |
useQuery | For making a GraphQL query |
useMutation | For making a GraphQL mutation |
This is exactly what you would pass to the normal js fetch
, with a little extra.
Option | Description | Default |
---|---|---|
onMount | Once the component mounts, the http request will run immediately | false |
url | Allows you to set a base path so relative paths can be used for each request :) | empty string |
const {
data,
loading,
error,
request,
get,
post,
patch,
put,
delete // don't destructure `delete` though, it's a keyword
del, // <- that's why we have this (del). or use `request.delete`
abort,
query, // GraphQL
mutate, // GraphQL
} = useFetch({
// accepts all `fetch` options such as headers, method, etc.
url: 'https://example.com', // used to be `baseUrl`
onMount: true
})
or
const [data, loading, error, request] = useFetch({
// accepts all `fetch` options such as headers, method, etc.
url: 'https://example.com', // used to be `baseUrl`
onMount: true
})
const {
get,
post,
patch,
put,
delete // don't destructure `delete` though, it's a keyword
del, // <- that's why we have this (del). or use `request.delete`
abort,
query, // GraphQL
mutate, // GraphQL
} = request
If you have feature requests, let's talk about them in this issue!
useMutation
+ useQuery
loading
statetimeout
debounce
retry: 3
which would specify the amount of times it should retry before erroring outoptions
(as 2nd param) to all hooks except useMutation
and useQuery
is an object, if not invariant
/throw error const user = useFetch()
user
.headers({
auth: jwt
})
.get()
import React, { Suspense, unstable_ConcurrentMode as ConcurrentMode, useEffect } from 'react'
function WithSuspense() {
const suspense = useFetch('https://example.com')
useEffect(() => {
suspense.read()
}, [])
if (!suspense.data) return null
return <pre>{suspense.data}</pre>
}
function App() (
<ConcurrentMode>
<Suspense fallback="Loading...">
<WithSuspense />
</Suspense>
</ConcurrentMode>
)
const App = () => {
const [todoTitle, setTodoTitle] = useState('')
// if there's no <Provider /> used, useMutation works this way
const mutation = useMutation('http://example.com', `
mutation CreateTodo($todoTitle string) {
todo(title: $todoTitle) {
id
title
}
}
`)
// ideally, I think it should be mutation.write({ todoTitle }) since mutation ~= POST
const createTodo = () => mutation.read({ todoTitle })
if (!request.data) return null
return (
<>
<input onChange={e => setTodoTitle(e.target.value)} />
<button onClick={createTodo}>Create Todo</button>
<pre>{mutation.data}</pre>
</>
)
}
FAQs
- useFetch - managed state, request, response, etc. [](https://codesandbox.io/s/usefetch-request-response-managed-state-ruyi3?file=/src/index.js) [](https://w
The npm package use-http receives a total of 12,786 weekly downloads. As such, use-http popularity was classified as popular.
We found that use-http demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.