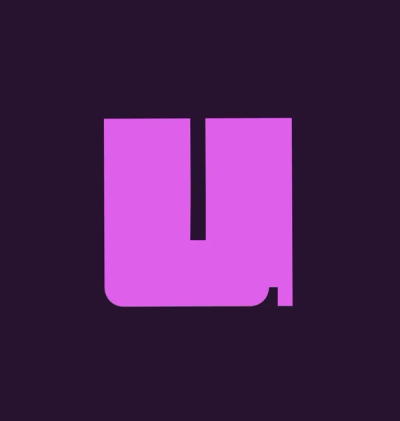
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
use-local-storage-state
Advanced tools
use-local-storage-state
React hook that persist data in local storage
npm install use-local-storage-state
Few other libraries also try to abstract the usage of localStorage into a hook. Here are the reasons why you would consider this one:
JSON.parse()
and JSON.stringify()
to support non string valuesistanbul ignore
storage
event which tracks changes across browser tabs and iframe'simport useLocalStorageState from 'use-local-storage-state'
const [todos, setTodos] = useLocalStorageState('todos', [
'buy milk',
'do 50 push-ups'
])
You can experiment with the example here.
import React, { useState } from 'react'
import useLocalStorageState from 'use-local-storage-state'
export default function Todos() {
const [query, setQuery] = useState('')
const [todos, setTodos] = useLocalStorageState('todos', ['buy milk'])
function onClick() {
setQuery('')
setTodos([...todos, query])
}
return (
<>
<input value={query} onChange={e => setQuery(e.target.value)} />
<button onClick={onClick}>Create</button>
{todos.map(todo => (<div>{todo}</div>))}
</>
)
}
The removeItem()
method will reset the value to its default and will remove the key from the localStorage
. It returns to the same state as when the hook was initially created.
import useLocalStorageState from 'use-local-storage-state'
const [todos, setTodos, { removeItem }] = useLocalStorageState('todos', [
'buy milk',
'do 50 push-ups'
])
function onClick() {
removeItem()
}
isPersistent
There are a few cases when localStorage
isn't available. The isPersistent
property tells you if the data is persisted in localStorage
or in-memory. Useful when you want to notify the user that their data won't be persisted.
import React, { useState } from 'react'
import useLocalStorageState from 'use-local-storage-state'
export default function Todos() {
const [todos, setTodos, { isPersistent }] = useLocalStorageState('todos', ['buy milk'])
return (
<>
{todos.map(todo => (<div>{todo}</div>))}
{!isPersistent && <span>Changes aren't currently persisted.</span>}
</>
)
}
Returns [value, setValue, { removeItem, isPersistent }]
. The first two values are the same as useState()
. The third value contains extra properties specific to localStorage
:
Type: string
The key used when calling localStorage.setItem(key)
and localStorage.getItem(key)
.
⚠️ Be careful with name conflicts as it is possible to access a property which is already in localStorage
that was created from another place in the codebase or in an old version of the application.
Type: any
Default: undefined
The initial value of the data. The same as useState(defaultValue)
property.
If you want to have the same data in multiple components in your code use createLocalStorageStateHook()
instead of useLocalStorageState()
. This avoids:
key
parameter misspellingsimport { createLocalStorageStateHook } from 'use-local-storage-state'
// Todos.tsx
const useTodos = createLocalStorageStateHook('todos', [
'buy milk',
'do 50 push-ups'
])
function Todos() {
const [todos, setTodos] = useTodos()
}
// Popup.tsx
import useTodos from './useTodos'
function Popup() {
const [todos, setTodos] = useTodos()
}
Type: string
The key used when calling localStorage.setItem(key)
and localStorage.getItem(key)
.
⚠️ Be careful with name conflicts as it is possible to access a property which is already in localStorage
that was created from another place in the codebase or in an old version of the application.
Type: any
Default: undefined
The initial value of the data. The same as useState(defaultValue)
property.
These are the best alternatives to my repo I have found so far:
FAQs
React hook that persist data in localStorage
The npm package use-local-storage-state receives a total of 52,040 weekly downloads. As such, use-local-storage-state popularity was classified as popular.
We found that use-local-storage-state demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.