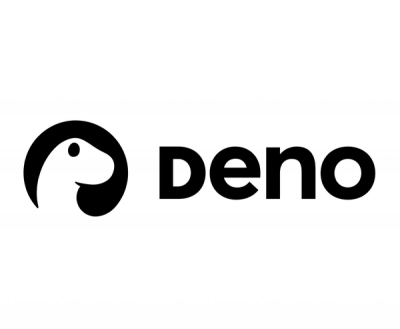
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
The 'util' package is a core Node.js module that provides utilities for programmatic use in JavaScript. It includes a collection of helper functions for various tasks such as formatting strings, inspecting objects, and using inheritance.
format
Formats a string using placeholders and returns it. Similar to printf in C.
const util = require('util');
console.log(util.format('%s:%s', 'foo', 'bar', 'baz')); // 'foo:bar baz'
inspect
Returns a string representation of an object for debugging purposes, with options to show hidden properties and control the depth of inspection.
const util = require('util');
console.log(util.inspect({ foo: 'bar' }, { showHidden: false, depth: null }));
inherits
Allows one constructor to inherit the prototype methods from another, a utility for implementing object-oriented inheritance.
const util = require('util');
function Base() {}
function Derived() {}
util.inherits(Derived, Base);
promisify
Converts a callback-based function to a function that returns a promise, facilitating the use of async/await.
const util = require('util');
const fs = require('fs');
const readFileAsync = util.promisify(fs.readFile);
readFileAsync('example.txt', 'utf8').then(console.log).catch(console.error);
Lodash is a utility library offering a wide range of methods for manipulating objects, arrays, strings, etc. It's more comprehensive than 'util' but doesn't include some of the Node.js-specific utilities like promisify.
Underscore.js is a utility library similar to Lodash, providing functional programming helpers without extending any built-in objects. It's less feature-rich compared to Lodash and doesn't include Node.js-specific utilities.
Chalk is a library for styling terminal strings. It doesn't offer the broad utility functions of 'util' but focuses on a specific area of string styling which 'util' doesn't cover.
Bluebird is a library focused on providing advanced features for promises, such as cancellation, progress, and long stack traces. It complements 'util' by enhancing promise functionality beyond what 'util.promisify' offers.
FAQs
Node.js's util module for all engines
The npm package util receives a total of 22,108,316 weekly downloads. As such, util popularity was classified as popular.
We found that util demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.