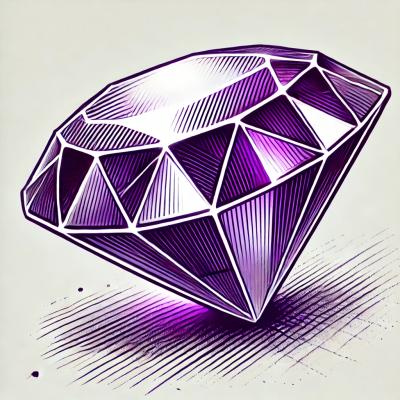
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
utils-indexof
Advanced tools
Returns the first index at which a given element can be found.
$ npm install utils-indexof
var indexOf = require( 'utils-indexof' );
Returns the first index at which a given element can be found.
var arr = [ 4, 3, 2, 1 ];
var idx = indexOf( arr, 3 );
// returns 1
If a searchElement
is not present in an input array
, the function
returns -1
.
var arr = [ 4, 3, 2, 1 ];
var idx = indexOf( arr, 5 );
// returns -1
By default, the implementation searches an input array
beginning from the first element. To start searching from a different element, specify a fromIndex
.
var arr = [ 1, 2, 3, 4, 5, 2, 6 ];
var idx = indexOf( arr, 2, 3 );
// returns 5
If a fromIndex
exceeds the input array
length, the function
returns -1
.
var arr = [ 1, 2, 3, 4, 2, 5 ];
var idx = indexOf( arr, 2, 10 );
// returns -1
If a fromIndex
is less than 0
, the starting index is determined relative to the last index (with the last index being equivalent to fromIndex = -1
).
var arr = [ 1, 2, 3, 4, 5, 2, 6, 2 ];
var idx = indexOf( arr, 2, -4 );
// returns 5
idx = indexOf( arr, 2, -1 );
// returns 7
If fromIndex
is less than 0
and its absolute value exceeds the input array
length, the function
searches the entire input array
.
var arr = [ 1, 2, 3, 4, 5, 2, 6 ];
var idx = indexOf( arr, 2, -10 );
// returns 1
The first argument is not limited to arrays
, but may be any array-like object
.
var str = 'bebop';
var idx = indexOf( str, 'o' );
// returns 3
Search is performed using strict equality comparison. Thus,
var arr = [ 1, [1,2,3], 3 ];
var idx = indexOf( arr, [1,2,3] );
// returns -1
This implementation is not ECMAScript Standard compliant. Notably, the standard specifies that an array
be searched by calling hasOwnProperty
(thus, for most cases, incurring a performance penalty), and the standard does not accommodate a searchElement
equal to NaN
. In this implementation, the following is possible:
// Locate the first element which is NaN...
var arr = [ 1, NaN, 2, NaN ];
var idx = indexOf( arr, NaN );
// returns 1
// Prototype properties may be searched as well...
function Obj() {
this[ 0 ] = 'beep';
this[ 1 ] = 'boop';
this[ 2 ] = 'woot';
this[ 3 ] = 'bap';
this.length = 4;
return this;
}
Obj.prototype[ 2 ] = 'bop';
var obj = new Obj();
idx = indexOf( obj, 'bop' );
// returns -1
delete obj[ 2 ];
idx = indexOf( obj, 'bop' );
// returns 2
var indexOf = require( 'utils-indexof' );
var arr;
var obj;
var str;
var idx;
var i;
// Arrays...
arr = new Array( 10 );
for ( i = 0; i < arr.length; i++ ) {
arr[ i ] = i * 10;
}
idx = indexOf( arr, 40 );
console.log( idx );
// returns 4
// Array-like objects...
obj = {
'0': 'beep',
'1': 'boop',
'2': 'bap',
'3': 'bop',
'length': 4
};
idx = indexOf( obj, 'bap' );
console.log( idx );
// returns 2
// Strings...
str = 'beepboopbop';
idx = indexOf( str, 'o' );
console.log( idx );
// returns 5
To run the example code from the top-level application directory,
$ node ./examples/index.js
This repository uses tape for unit tests. To run the tests, execute the following command in the top-level application directory:
$ make test
All new feature development should have corresponding unit tests to validate correct functionality.
This repository uses Istanbul as its code coverage tool. To generate a test coverage report, execute the following command in the top-level application directory:
$ make test-cov
Istanbul creates a ./reports/coverage
directory. To access an HTML version of the report,
$ make view-cov
This repository uses Testling for browser testing. To run the tests in a (headless) local web browser, execute the following command in the top-level application directory:
$ make test-browsers
To view the tests in a local web browser,
$ make view-browser-tests
Copyright © 2016. Athan Reines.
FAQs
Returns the first index at which a given element can be found.
We found that utils-indexof demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.