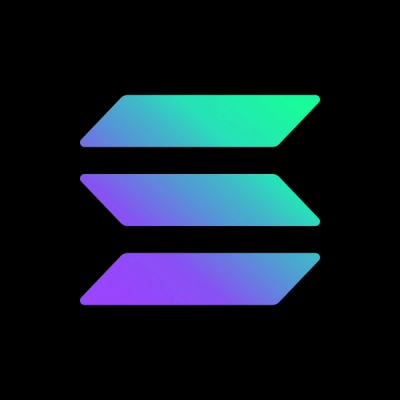
Security News
Supply Chain Attack Detected in @solana/web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Fast, generic JavaScript/node.js utility functions.
npm i utils --save
To get all utils grouped by collection:
var utils = require('utils');
// All utils are on the `_` property
console.log(utils._);
Get a specific collection
var utils = require('utils');
// get only the string utils
var string = utils.string;
Statements : 99.72% ( 359/360 )
Branches : 97.4% ( 187/192 )
Functions : 100% ( 81/81 )
Lines : 99.68% ( 310/311 )
These links take you to the code for each function. There are currently 0 utils in 7 sub-categories:
Returns all of the items in an array after the specified index.
array
{Array}: Collectionn
{Number}: Starting index (number of items to exclude)returns
{Array}: Array exluding n
items.after(['a', 'b', 'c'], 1)
//=> ['c']
Cast the give value
to an array.
val
{*}returns
: {Array}arrayify('abc')
//=> ['abc']
arrayify(['abc'])
//=> ['abc']
Returns all of the items in an array up to the specified number Opposite of <%= after() %
.
array
{Array}n
{Number}returns
{Array}: Array excluding items after the given number.before(['a', 'b', 'c'], 2)
//=> ['a', 'b']
Remove all falsey values from an array.
arr
{Array}returns
: {Array}compact([null, a, undefined, 0, false, b, c, '']);
//=> [a, b, c]
Return the difference between the first array and additional arrays.
a
{Array}b
{Array}returns
: {Array}var a = ['a', 'b', 'c', 'd'];
var b = ['b', 'c'];
diff(a, b);
//=> ['a', 'd']
Returns the first item, or first n
items of an array.
array
{Array}n
{Number}: Number of items to return, starting at 0
.returns
: {Array}first(['a', 'b', 'c', 'd', 'e'], 2)
//=> ['a', 'b']
Recursively flatten an array or arrays. Uses the fastest implementation of array flatten for node.js
array
{Array}returns
{Array}: Flattened arrayflatten(['a', ['b', ['c']], 'd', ['e']]);
//=> ['a', 'b', 'c', 'd', 'e']
Loop over each item in an array and call the given function on every element.
array
{Array}fn
{Function}thisArg
{Object}: Optionally pass a thisArg
to be used as the context in which to call the function.returns
: {Array}forEach(['a', 'b', 'c'], function (ele) {
return ele + ele;
});
//=> ['aa', 'bb', 'cc']
forEach(['a', 'b', 'c'], function (ele, i) {
return i + ele;
});
//=> ['0a', '1b', '2c']
Returns true if the given value
is an array.
value
{Array}: Value to test.isArray(1);
//=> 'false'
isArray([1]);
//=> 'true'
Returns the last item, or last n
items of an array.
array
{Array}n
{Number}: Number of items to return, starting with the last item.returns
: {Array}last(['a', 'b', 'c', 'd', 'e'], 2)
//=> ['d', 'e']
Returns a new array with the results of calling the given function on every element in the array. This is a faster, node.js focused alternative to JavaScript's native array map.
array
{Array}fn
{Function}returns
: {Array}map(['a', 'b', 'c'], function (ele) {
return ele + ele;
});
//=> ['aa', 'bb', 'cc']
map(['a', 'b', 'c'], function (ele, i) {
return i + ele;
});
//=> ['0a', '1b', '2c']
Return an array free of duplicate values. Fastest ES5 implementation.
array
{Array}: The array to uniquifyreturns
{Array}: With all union values.union(['a', 'b', 'c', 'c']);
//=> ['a', 'b', 'c']
Return an array free of duplicate values. Fastest ES5 implementation.
array
{Array}: The array to uniquifyreturns
{Array}: With all unique values.unique(['a', 'b', 'c', 'c']);
//=> ['a', 'b', 'c']
value
{*}target
{*}options
{Object}Returns true
if value
exists in the given string, array
or object. See [any] for documentation.
collection
{Array|Object}string
{*}returns
: {Boolean}Return true if collection
contains value
dir
{String}: Starting directoryreturns
{Array}: Array of files.Try to read the given directory
. Wraps fs.readdirSync()
with
a try/catch, so it fails silently instead of throwing when the
directory doesn't exist.
fp
{String}: File path of the file to requirereturns
{*}: Returns the module function/object, or null
if not found.Try to require the given file, returning null
if not successful
instead of throwing an error.
Returns true if any value exists, false if empty. Works for booleans, functions, numbers, strings, nulls, objects and arrays.
object
{Object}: The object to check for value
value
{*}: the value to look forreturns
{Boolean}: True if any values exists.utils.lang.hasValues('a');
//=> true
utils.lang.hasValues('');
//=> false
utils.lang.hasValues(1);
//=> true
utils.lang.hasValues({a: 'a'}});
//=> true
utils.lang.hasValues({}});
//=> false
utils.lang.hasValues(['a']);
//=> true
Return true if the given value
is an arguments object.
value
{*}: The value to check.returns
: {Boolean}isArguments(arguments)
//=> 'true'
Returns true if the given value is empty, false if any value exists. Works for booleans, functions, numbers, strings, nulls, objects and arrays.
object
{Object}: The object to check for value
value
{*}: the value to look forreturns
{Boolean}: False if any values exists.utils.lang.isEmpty('a');
//=> true
utils.lang.isEmpty('');
//=> false
utils.lang.isEmpty(1);
//=> true
utils.lang.isEmpty({a: 'a'}});
//=> true
utils.lang.isEmpty({}});
//=> false
utils.lang.isEmpty(['a']);
//=> true
Return true if the given value
is an object with keys.
value
{Object}: The value to check.returns
: {Boolean}isObject(['a', 'b', 'c'])
//=> 'false'
isObject({a: 'b'})
//=> 'true'
Return true if the given value
is an object with keys.
value
{Object}: The value to check.returns
: {Boolean}isPlainObject(Object.create({}));
//=> true
isPlainObject(Object.create(Object.prototype));
//=> true
isPlainObject({foo: 'bar'});
//=> true
isPlainObject({});
//=> true
Returns the sum of all numbers in the given array.
array
{Array}: Array of numbers to add up.returns
: {Number}sum([1, 2, 3, 4, 5])
//=> '15'
o
{Object}: The target object. Pass an empty object to shallow clone.objects
{Object}returns
: {Object}Extend o
with properties of other objects
.
Returns a copy of the given obj
filtered to have only enumerable properties that have function values.
obj
{Object}returns
: {Object}functions({a: 'b', c: function() {}});
//=> {c: function() {}}
Return true if key
is an own, enumerable property of the given obj
.
key
{String}obj
{Object}: Optionally pass an object to check.returns
: {Boolean}hasOwn(obj, key);
obj
{Object}returns
{Array}: Array of keys.Return an array of keys for the given obj
. Uses Object.keys
when available, otherwise falls back on forOwn
.
o
{Object}: The target object. Pass an empty object to shallow clone.objects
{Object}returns
: {Object}Recursively combine the properties of o
with the
properties of other objects
.
obj
{Object}returns
: {Array}Returns a list of all enumerable properties of obj
that have function
values
camelCase the characters in string
.
string
{String}: The string to camelcase.returns
: {String}camelcase("foo bar baz");
//=> 'fooBarBaz'
Center align the characters in a string using non-breaking spaces.
str
{String}: The string to reverse.returns
{String}: Centered string.centerAlign("abc");
Like trim, but removes both extraneous whitespace and non-word characters from the beginning and end of a string.
string
{String}: The string to chop.returns
: {String}chop("_ABC_");
//=> 'ABC'
chop("-ABC-");
//=> 'ABC'
chop(" ABC ");
//=> 'ABC'
Count the number of occurrances of a substring within a string.
string
{String}substring
{String}returns
{Number}: The occurances of substring
in string
count("abcabcabc", "a");
//=> '3'
dash-case the characters in string
. This is similar to [slugify], but [slugify] makes the string compatible to be used as a URL slug.
string
{String}returns
: {String}dashcase("a b.c d_e");
//=> 'a-b-c-d-e'
dot.case the characters in string
.
string
{String}returns
: {String}dotcase("a-b-c d_e");
//=> 'a.b.c.d.e'
Truncate a string to the specified length
, and append it with an elipsis, …
.
str
{String}length
{Number}: The desired length of the returned string.ch
{String}: Optionally pass custom characters to append. Default is …
.returns
{String}: The truncated string.ellipsis("<span>foo bar baz</span>", 7);
//=> 'foo bar…'
Replace spaces in a string with hyphens. This
string
{String}returns
: {String}hyphenate("a b c");
//=> 'a-b-c'
Returns true if the value is a string.
val
{String}returns
{Boolean}: True if the value is a string.isString('abc');
//=> 'true'
isString(null);
//=> 'false'
PascalCase the characters in string
.
string
{String}returns
: {String}pascalcase("foo bar baz");
//=> 'FooBarBaz'
path/case the characters in string
.
string
{String}returns
: {String}pathcase("a-b-c d_e");
//=> 'a/b/c/d/e'
Replace occurrences of a
with b
.
str
{String}a
{String|RegExp}: Can be a string or regexp.b
{String}returns
: {String}replace("abcabc", /a/, "z");
//=> 'zbczbc'
Reverse the characters in a string.
str
{String}: The string to reverse.returns
: {String}reverse("abc");
//=> 'cba'
Right align the characters in a string using non-breaking spaces.
str
{String}: The string to reverse.returns
{String}: Right-aligned string.rightAlign(str);
Sentence-case the characters in string
.
string
{String}returns
: {String}sentencecase("foo bar baz.");
//=> 'Foo bar baz.'
snake_case the characters in string
.
string
{String}returns
: {String}snakecase("a-b-c d_e");
//=> 'a_b_c_d_e'
Truncate a string by removing all HTML tags and limiting the result to the specified length
.
str
{String}length
{Number}: The desired length of the returned string.returns
{String}: The truncated string.truncate("<span>foo bar baz</span>", 7);
//=> 'foo bar'
Wrap words to a specified width using word-wrap.
string
{String}: The string with words to wrap.object
{Options}: Options to pass to word-wrapreturns
{String}: Formatted string.wordwrap("a b c d e f", {width: 5, newline: '<br> '});
//=> ' a b c <br> d e f'
-----------------------|-----------|-----------|-----------|-----------|
File | % Stmts | % Branches | % Funcs | % Lines |
---|---|---|---|---|
array/ | 76.24 | 67.19 | 85.71 | 77.32 |
after.js | 100 | 75 | 100 | 100 |
arrayify.js | 100 | 100 | 100 | 100 |
before.js | 100 | 75 | 100 | 100 |
compact.js | 100 | 100 | 100 | 100 |
difference.js | 100 | 100 | 100 | 100 |
first.js | 88.89 | 83.33 | 100 | 88.24 |
flatten.js | 100 | 100 | 100 | 100 |
forEach.js | 12.5 | 0 | 0 | 14.29 |
indexOf.js | 7.69 | 0 | 0 | 8.33 |
isArray.js | 100 | 100 | 100 | 100 |
last.js | 88.89 | 83.33 | 100 | 88.24 |
map.js | 100 | 100 | 100 | 100 |
sort.js | 90.91 | 87.5 | 100 | 90.91 |
union.js | 100 | 100 | 100 | 100 |
unique.js | 100 | 100 | 100 | 100 |
collection/ | 50 | 0 | 0 | 50 | any.js | 100 | 100 | 100 | 100 | contains.js | 42.86 | 0 | 0 | 42.86 | fs/ | 80 | 100 | 100 | 80 | tryReaddir.js | 80 | 100 | 100 | 80 | tryRequire.js | 80 | 100 | 100 | 80 | lang/ | 75 | 50 | 25 | 75 | hasValues.js | 100 | 100 | 100 | 100 | isArguments.js | 50 | 25 | 0 | 50 | isEmpty.js | 66.67 | 100 | 0 | 66.67 | isObject.js | 100 | 100 | 100 | 100 | isPlainObject.js | 100 | 100 | 100 | 100 | math/ | 100 | 100 | 100 | 100 | sum.js | 100 | 100 | 100 | 100 | object/ | 69.57 | 54.55 | 27.27 | 68.66 | extend.js | 100 | 83.33 | 100 | 100 | filter.js | 100 | 100 | 100 | 100 | forIn.js | 100 | 100 | 100 | 100 | forOwn.js | 100 | 100 | 100 | 100 | functions.js | 28.57 | 0 | 0 | 28.57 | hasOwn.js | 100 | 100 | 100 | 100 | keys.js | 33.33 | 50 | 0 | 33.33 | merge.js | 100 | 75 | 100 | 100 | methods.js | 28.57 | 0 | 0 | 28.57 | omit.js | 100 | 100 | 100 | 100 | pick.js | 100 | 100 | 100 | 100 | reduce.js | 100 | 100 | 100 | 100 | some.js | 30 | 0 | 0 | 30 | string/ | 99.27 | 96.77 | 96 | 99.09 | camelcase.js | 100 | 100 | 100 | 100 | centerAlign.js | 100 | 100 | 100 | 100 | chop.js | 100 | 100 | 100 | 100 | count.js | 100 | 100 | 100 | 100 | dashcase.js | 100 | 100 | 100 | 100 | dotcase.js | 100 | 100 | 100 | 100 | ellipsis.js | 100 | 100 | 100 | 100 | hyphenate.js | 100 | 100 | 100 | 100 | index.js | 100 | 100 | 100 | 100 | isString.js | 100 | 100 | 100 | 100 | pascalcase.js | 100 | 100 | 100 | 100 | pathcase.js | 100 | 100 | 100 | 100 | replace.js | 100 | 100 | 100 | 100 | reverse.js | 100 | 100 | 100 | 100 | rightAlign.js | 100 | 100 | 100 | 100 | sentencecase.js | 100 | 100 | 100 | 100 | slugify.js | 100 | 100 | 100 | 100 | snakecase.js | 100 | 100 | 100 | 100 | toString.js | 50 | 0 | 0 | 50 | truncate.js | 100 | 100 | 100 | 100 | wordwrap.js | 100 | 100 | 100 | 100 | -----------------------|-----------|-----------|-----------|-----------|
All files | 84.15 | 75.95 | 72.88 | 83.12 |
---|
Install dev dependencies.
npm i -d && npm test
Pull requests and stars are always welcome. For bugs and feature requests, please create an issue
Jon Schlinkert
Copyright (c) 2015 Jon Schlinkert
Released under the MIT license
This file was generated by verb-cli on March 25, 2015.
{%= reflinks('') %}
FAQs
Fast, generic JavaScript/node.js utility functions.
The npm package utils receives a total of 18,115 weekly downloads. As such, utils popularity was classified as popular.
We found that utils demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.