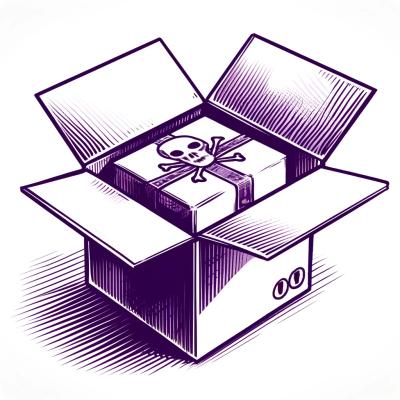
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
A modern type guard utility for Node.js
Requires Node.js v6 LTS or greater.
$ npm install vakt
vakt throws an error when a variable is not of the expected type.
const vakt = require('vakt');
const greet = (name) => {
vakt.check({ name }, 'string');
return `Hello, ${name}!`;
};
greet('Alice'); // => Hello, Alice!
greet(3.14159); // => TypeError: name must be a string
vakt takes advantage of the ES6/ES2015 property value shorthand so you don't have to write the variable twice.
You can also use vakt in Promises, since thrown errors automatically become rejections.
const vakt = require('vakt');
const delay = (ms) => {
return new Promise((resolve, reject) => {
vakt.check({ ms }, 'number');
// if we reach this part, ms is guaranteed to be a number
setTimeout(resolve, ms);
});
};
delay(5000).then(() => {
console.log('5 seconds have passed!');
});
// => 5 seconds have passed!
delay(false).catch((err) => {
console.error(err);
});
// => TypeError: ms must be a number
vakt lets you create your own type checks by providing it with a validation function that returns true or false.
const vakt = require('vakt');
vakt.customTypes['non-negative integer'] = (value) => vakt.is.integer(value) && value >= 0;
const pick = (array, index) => {
vakt.check({ array }, 'array');
vakt.check({ index }, 'non-negative integer');
return array[index];
};
pick([4, 8, 15, 16, 23, 42], 3); // => 16
pick(['rainbows', 'unicorns'], -12.5); // => TypeError: index must be a non-negative integer
vakt also has a handy shorthand for checking multiple variables with one call.
const vakt = require('vakt');
const doMaths = (num1, num2, round) => {
vakt.check([
[{ num1 }, 'number'],
[{ num2 }, 'number'],
[{ round }, 'boolean'],
]);
let value = 1 / (num1 * num2);
if (round) value = Math.round(value);
return value;
};
doMaths(1, 2, false); // => 0.5
doMaths(1, 2, 3) // => TypeError: round must be a boolean
vakt.check(variable, type)
vakt.check([[variable, type], [variable, type], [...]])
variable
Type: object
The variable to check. Should be in the form of { name: value }
.
Since you will practically always want to pass both the name and value of a variable,
you can make use of the ES6/ES2015 property value shorthand to save you some typing: { name }
type
Type: string
The type to validate against, passed as a string.
vakt.types
Type: array
An array containing the types vakt can check out of the box:
[
'array',
'boolean',
'date',
'decimal',
'function',
'integer',
'number',
'object',
'regexp',
'string',
]
vakt.is
Type: object
An instance of the is.js micro check library which vakt uses to perform type checks.
vakt.customTypes
Type: object
An object containing the custom types that have been added to vakt. To define a new custom type you can add a validation function directly to the object. The validation function should return true
or false
.
vakt.customTypes['cat'] = (value) => vakt.is.object(value) && vakt.is.function(value['meow']);
Once you have defined a custom type, you can use it with vakt.check
:
const dog = {
woof () {
console.log('woof!');
},
};
vakt.check({ dog }, 'cat'); // => TypeError: dog must be a cat
vakt.VERSION
Type: string
The version of vakt in semver format.
MIT © Mick Dekkers
FAQs
Unknown package
The npm package vakt receives a total of 0 weekly downloads. As such, vakt popularity was classified as not popular.
We found that vakt demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.