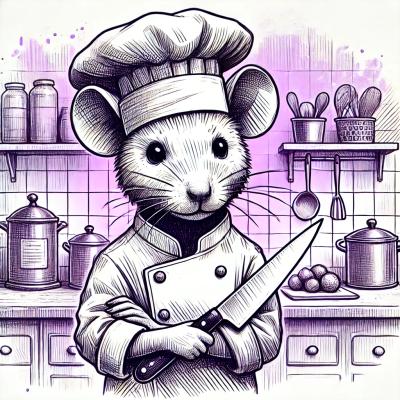
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
value-equal
Advanced tools
The value-equal npm package is a simple utility for checking the equality of two values. It is primarily used to determine if two values are equivalent in terms of their actual content, rather than being the same reference. This is particularly useful in scenarios where deep comparison of objects, arrays, or other complex data structures is required.
Deep Equality Check
This feature allows you to check if two values are equivalent, looking deeply into the structure of objects or arrays. It returns true if the values are equivalent, otherwise false.
const valueEqual = require('value-equal');
console.log(valueEqual({ a: 1 }, { a: 1 })); // true
console.log(valueEqual([1, 2], [1, 2])); // true
console.log(valueEqual('hello', 'hello')); // true
console.log(valueEqual(42, 42)); // true
console.log(valueEqual(null, null)); // true
console.log(valueEqual(undefined, undefined)); // true
console.log(valueEqual({ a: 1 }, { a: 2 })); // false
deep-equal is another npm package that provides deep comparison functionality. It is similar to value-equal but offers more detailed options for comparison, such as strict mode checks and handling of prototype properties.
lodash.isequal is a method from the popular Lodash library that performs a deep comparison between two values to determine if they are equivalent. It supports a wider range of data types and has optimizations for large datasets, making it more versatile than value-equal.
value-equal
determines if two JavaScript values are equal using Object.prototype.valueOf
.
In many instances when I'm checking for object equality, what I really want to know is if their values are equal. This is good for:
localStorage
window.history.state
valuesUsing npm:
$ npm install --save value-equal
Then with a module bundler like webpack, use as you would anything else:
// using ES6 modules
import valueEqual from 'value-equal';
// using CommonJS modules
var valueEqual = require('value-equal');
The UMD build is also available on unpkg:
<script src="https://unpkg.com/value-equal"></script>
You can find the library on window.valueEqual
.
valueEqual(1, 1); // true
valueEqual('asdf', 'asdf'); // true
valueEqual('asdf', new String('asdf')); // true
valueEqual(true, true); // true
valueEqual(true, false); // false
valueEqual({ a: 'a' }, { a: 'a' }); // true
valueEqual({ a: 'a' }, { a: 'b' }); // false
valueEqual([1, 2, 3], [1, 2, 3]); // true
valueEqual([1, 2, 3], [2, 3, 4]); // false
That's it. Enjoy!
FAQs
Are these two JavaScript values equal?
The npm package value-equal receives a total of 3,056,077 weekly downloads. As such, value-equal popularity was classified as popular.
We found that value-equal demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.