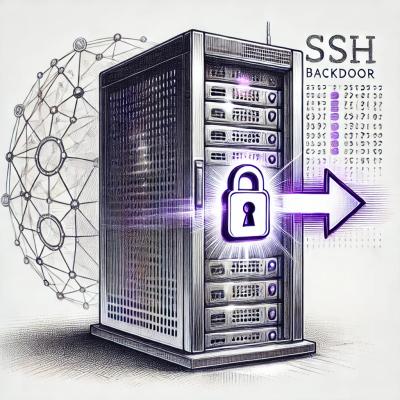
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Up-to-date European VAT Rates
VAT rates stored in JSON
format, with Javascript and PHP classes for easy access.
<script src='https://unpkg.com/vatrates@2/dist/vatrates.min.js'></script>
<!-- or -->
<script src='https://unpkg.com/vatrates@2/dist/vatrates.js'></script>
npm install vatrates
For the PHP package:
composer require staaky/vatrates
Require vatrates or use a script tag to include vatrates.js:
var vatRates = require('vatrates');
<script src='vatrates.js'></script>
An instance created with new VATRates()
gives you several VAT rate functions. It's recommended to always use an isVATCountry()
check before using them, like this:
var VATRates = require('vatrates');
var vatRates = new VATRates();
if (vatRates.isVATCountry('RO')) {
console.log(vatRates.getSuperReducedRate('RO')); // -> undefined
console.log(vatRates.getReducedRates('RO')); // -> [5, 9]
console.log(vatRates.getStandardRate('RO')); // -> 19
console.log(vatRates.getParkingRate('RO')); // -> undefined
}
Default rates are for today, to get rates for a different day pass in a Date
:
var vatRates = new VATRates(new Date('2016-01-01'));
if (vatRates.isVATCountry('RO')) {
console.log(vatRates.getStandardRate('RO')); // -> 20
}
You can also change the date by calling setDate()
on a previously created instance:
var vatRates = new VATRates();
vatRates.setDate(new Date('2015-01-01'));
if (vatRates.isVATCountry('RO')) {
console.log(vatRates.getStandardRate('RO')); // -> 24
}
Another approach is to use getCountry()
, it returns a VATCountry
or undefined
if the country doesn't use VAT. With a VATCountry you'll have all the VAT rate functions and some extra helpers.
var vatRates = new VATRates();
var country;
if ((country = vatRates.getCountry('GB'))) {
console.log(country.getName()); // -> "United Kingdom"
console.log(country.getCode()); // -> "UK"
console.log(country.getCountryCode()); // -> "GB"
console.log(country.getSuperReducedRate()); // -> undefined
console.log(country.getReducedRates()); // -> [5]
console.log(country.getStandardRate()); // -> 20
console.log(country.getParkingRate()); // -> undefined
}
The United Kingdom and Greece use extra non standard country codes "UK" and "EL", these are also accepted.
vatRates.getCountry('UK'); // -> same result as 'GB'
Returns an Array
of all the countries using VAT, as VATCountry
's.
var vatRates = new VATRates();
var countries = vatRates.getCountries();
countries.forEach(function(country) {
console.log(country.getCountryCode() + " has VAT: " + country.getStandardRate());
});
Set the Date
for which to return VAT rates.
var vatRates = new VATRates();
vatRates.setDate(new Date('2015-01-01'));
This is identical to:
var vatRates = new VATRates(new Date('2015-01-01'));
All other functions take this date into account, so make sure to always set the date first.
Returns true
if a country uses VAT, or false
if not.
var vatRates = new VATRates();
console.log(vatRates.isVATCountry('FR')); // -> true
console.log(vatRates.isVATCountry('US')); // -> false
Returns the super reduced rate for a country, or undefined
when none is available.
var vatRates = new VATRates();
if (vatRates.isVATCountry('FR')) {
console.log(vatRates.getSuperReducedRate('FR')); // -> 2.1
});
A VATCountry
returned by getCountry()
offers this method directly.
var vatRates = new VATRates();
var country;
if ((country = vatRates.getCountry('FR'))) {
console.log(country.getName()); // -> "France"
console.log(country.getSuperReducedRate()); // -> 2.1
}
Returns an Array
of reduced rates for a country, or undefined
when none are available.
var vatRates = new VATRates();
if (vatRates.isVATCountry('IE')) {
console.log(vatRates.getReducedRates('IE')); // -> [9, 13.5]
});
A VATCountry
offers this method directly.
var vatRates = new VATRates();
var country;
if ((country = vatRates.getCountry('IE'))) {
console.log(country.getName()); // -> "Ireland"
console.log(country.getReducedRates()); // -> [9, 13.5]
}
Returns the standard rate for a country, or undefined
when none is available.
var vatRates = new VATRates();
if (vatRates.isVATCountry('NL')) {
console.log(vatRates.getStandardRate('NL')); // -> 21
});
A VATCountry
offers this method directly.
var vatRates = new VATRates();
var country;
if ((country = vatRates.getCountry('NL'))) {
console.log(country.getName()); // -> "Netherlands"
console.log(country.getStandardRate()); // -> 21
}
Returns the parking rate for a country, or undefined
when none is available.
var vatRates = new VATRates();
if (vatRates.isVATCountry('LU')) {
console.log(vatRates.getParkingRate('LU')); // -> 14
}
A VATCountry
offers this method directly.
var vatRates = new VATRates();
var country;
if ((country = vatRates.getCountry('LU'))) {
console.log(country.getName()); // -> "Luxembourg"
console.log(country.getParkingRate()); // -> 14
}
After installing through Composer use Staaky\VATRates\VATRates
composer require staaky/vatrates
use Staaky\VATRates\VATRates;
An instance created with new VATRates()
gives you several VAT rate functions. It's recommended to always use an isVATCountry()
check before using them, like this:
$vatRates = new VATRates();
if ($vatRates->isVATCountry('RO')) {
var_dump($vatRates->getSuperReducedRate('RO')); // -> null
var_dump($vatRates->getReducedRates('RO')); // -> [5, 9]
var_dump($vatRates->getStandardRate('RO')); // -> 19
var_dump($vatRates->getParkingRate('RO')); // -> null
}
Default rates are for today, to get rates for a different day pass in a DateTime
:
$vatRates = new VATRates(new DateTime('2016-01-01'));
if ($vatRates->isVATCountry('RO')) {
var_dump($vatRates->getStandardRate('RO')); // -> 20
}
You can also change the date by calling setDate()
on a previously created instance:
$vatRates = new VATRates();
$vatRates->setDate(new DateTime('2015-01-01'));
if ($vatRates->isVATCountry('RO')) {
var_dump($vatRates->getStandardRate('RO')); // -> 24
}
Another approach is to use getCountry()
, it returns a VATCountry
or null
if the country doesn't use VAT. With a VATCountry
you'll have all the VAT rate functions and some extra helpers.
$vatRates = new VATRates();
if (($country = $vatRates->getCountry('GB'))) {
var_dump($country->getName()); // -> "United Kingdom"
var_dump($country->getCode()); // -> "UK"
var_dump($country->getCountryCode()); // -> "GB"
var_dump($country->getSuperReducedRate()); // -> null
var_dump($country->getReducedRates()); // -> [5]
var_dump($country->getStandardRate()); // -> 20
var_dump($country->getParkingRate()); // -> null
}
The United Kingdom and Greece use extra non standard country codes "UK" and "EL", these are also accepted.
$vatRates->getCountry('UK'); // -> same result as 'GB'
Returns an array
of all the countries using VAT, as VATCountry
's.
$vatRates = new VATRates();
$countries = $vatRates->getCountries();
foreach ($countries as $country) {
print_r($country->getCountryCode() . " has VAT: " . $country->getStandardRate() . "\n");
}
Set the date for which to return VAT rates.
$vatRates = new VATRates();
$vatRates->setDate(new DateTime('2015-01-01'));
This is identical to:
$vatRates = new VATRates(new DateTime('2015-01-01'));
All other functions take this date into account, so make sure to always set the date first.
Returns true
if a country uses VAT, or false
if not.
$vatRates = new VATRates();
var_dump($vatRates->isVATCountry('FR')); // -> true
var_dump($vatRates->isVATCountry('US')); // -> false
Returns the super reduced rate for a country, or null
if none is available.
$vatRates = new VATRates();
if ($vatRates->isVATCountry('FR')) {
var_dump($vatRates->getSuperReducedRate('FR')); // -> 2.1
});
A VATCountry
returned by getCountry()
offers this method directly.
$vatRates = new VATRates();
if (($country = $vatRates->getCountry('FR'))) {
var_dump($country->getName()); // -> "France"
var_dump($country->getSuperReducedRate()); // -> 2.1
}
Returns an array
of reduced rates for a country, or null
if none are available.
$vatRates = new VATRates();
if ($vatRates->isVATCountry('IE')) {
var_dump($vatRates->getReducedRates('IE')); // -> [9, 13.5]
}
A VATCountry
offers this method directly.
$vatRates = new VATRates();
if (($country = $vatRates->getCountry('IE'))) {
var_dump($country->getName()); // -> "Ireland"
var_dump($country->getReducedRates()); // -> [9, 13.5]
}
Returns the standard rate for a country, or null
when none is available.
$vatRates = new VATRates();
if ($vatRates->isVATCountry('NL')) {
var_dump($vatRates->getStandardRate('NL')); // -> 21
});
A VATCountry
offers this method directly.
$vatRates = new VATRates();
if (($country = $vatRates->getCountry('NL'))) {
var_dump($country->getName()); // -> "Netherlands"
var_dump($country->getStandardRate()); // -> 21
}
Returns the parking rate for a country, or null
when none is available.
$vatRates = new VATRates();
if ($vatRates->isVATCountry('LU')) {
var_dump($vatRates->getParkingRate('LU')); // -> 14
});
A VATCountry
offers this method directly.
$vatRates = new VATRates();
if (($country = $vatRates->getCountry('LU'))) {
var_dump($country->getName()); // -> "Luxembourg"
var_dump($country->getParkingRate()); // -> 14
}
Use webpack
to create a new build after you've made changes.
webpack
Use gulp
to load up the page in the /example
folder. It shows the output of all files (Javascript, JSON & PHP) and automatically rebuilds with webpack as you modify source code.
gulp
Run unit tests using npm test
, this runs tests for every language.
npm test
Javascript tests are run with Mocha and PHP tests with Peridot. They can be run individually as well:
npm run mocha
npm run peridot
VAT rates are kept up to date manually using data from the European Commission and VATLive.com. Initial historic rates are based on data from jsonvat.com.
If you notice an incorrect rate please create an issue or send a pull request. Future VAT changes can also be added to the JSON
file. If you know of an upcoming change that isn't listed yet, please let me know.
Data on historic VAT rates is also appreciated. This can be hard to track down, especially the non-standard rates.
VATRates is MIT Licensed
FAQs
Up-to-date European VAT Rates
The npm package vatrates receives a total of 1,402 weekly downloads. As such, vatrates popularity was classified as popular.
We found that vatrates demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.