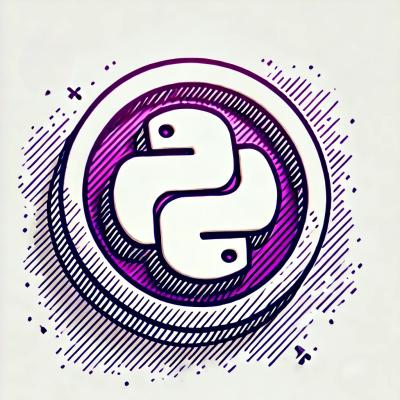
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
The lightweight JSX compatible templating engine.
JSX is powerful compared to other templating engines but it has some warts. It has some abstractions that simply don't make sense for creating html (ala className). "VDO" provides a JSX interface that is specifically designed for rendering html, not DOM.
npm install vdo
bower install vdo
/** @jsx vdo */
const vdo = require('vdo');
function MyPartial (attrs, children) {
return <span class="custom" data-value={attrs.value}/>;
}
const html = (
<div class="root">
<MyPartial value="1"/>
</div>
);
document.body.innerHTML = html;
element
is a vdo virtual element.vdo.isElement(<div/>); // true
context
.// renderer must be a function that returns a virtual node.
function MyComponent (props, children, context) {
return (
<div>External data: { context }</div>
);
}
String(vdo.with(1, ()=> <MyComponent/>));
//-> "<div>External Data: 1</div>"
// Automatically called when using JSX.
let vNode = vdo.createElement("div", { editable: true }, "Hello World");
// Or call vdo directly
let vNode = vdo("div", { editable: true }, "Hello World");
// Render to string on the server.
vNode.toString(); // '<div editable="true">Hello World</div>';
/**
* @params type can also be a function (shown in example above).
*/
Please feel free to create a PR!
FAQs
Minimal JSX compatible html focused templating engine.
The npm package vdo receives a total of 3 weekly downloads. As such, vdo popularity was classified as not popular.
We found that vdo demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.