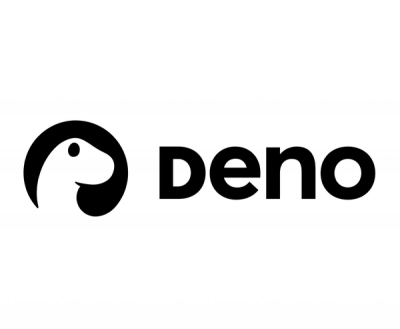
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
veritone-client-js
Advanced tools
We provide bundles for the browser and for node.
Install the package:
npm install --save veritone-client-js
in your code, import either veritone-client-js/dist/bundle-browser
or veritone-client-js/dist/bundle-node
, as described below.
See the docs on authentication at https://veritone-developer.atlassian.net/
// in a browser:
import veritoneApi from 'veritone-client-js/dist/bundle-browser'
// in Node:
import veritoneApi from 'veritone-client-js/dist/bundle-node'
const client = VeritoneApi({
// requires either session AND api tokens (from user object):
token: 'my-session-token',
apiToken: 'my-api-token',
// OR an oauth token (from oauth grant flow)
oauthToken: 'my-oauth-token',
// optional (defaults shown):
baseUrl: 'https://api.veritone.com',
maxRetries: 1,
retryIntervalMs: 1000
})
// handlers support both promise and callback styles:
// Callbacks are node-style (err, data).
// Verify err === null before accessing data.
// https://nodejs.org/api/errors.html#errors_node_js_style_callbacks
client.recording.getRecordings(function (err, recordings) {
if (err) {
// err is an Error or ApiError instance (see ApiError below)
return console.warn(err);
}
recordings.forEach(function (recording) {
console.log(recording);
})
});
// promise style
client.recording.getRecordings()
.then(function (recordings) {
recordings.forEach(function (recording) {
console.log(recording);
})
})
.catch(function (err) {
// err is an Error or ApiError instance (see ApiError below)
console.warn(err);
});
If the handler has options, provide them as specified:
client.recording.getRecordings({ offset: 5, limit: 10 }, function (err, recordings) {
// ...
})
The callback is always the final (optional) argument
Each handler supports overriding API client options and some request options on a per-call basis. The options object is always the last (when using promise style), or second to last (when a callback is provided) argument.
client.recording.getRecordings(
{ offset: 5, limit: 10 },
{
maxRetries: 0,
headers: {
'My-Additional-Header': 'hi'
}
},
function (err, recordings) {
// ...
}
)
Errors related to API calls, including responses with status > 300 and network error are wrapped by ApiError.
(WIP)
const {
method,
path,
data,
query,
headers,
// default options for this request, if different from defaults
_requestOptions = {
// maxRetries,
// retryIntervalMs,
// timeoutMs,
// headers,
// transformResponseData,
// validateStatus
// tokenType
// version
}
} = request;
Copyright (c) Veritone Corporation. All rights reserved.
Licensed under the Apache 2.0 License.
FAQs
## Installation We provide bundles for the browser and for node.
The npm package veritone-client-js receives a total of 20 weekly downloads. As such, veritone-client-js popularity was classified as not popular.
We found that veritone-client-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 12 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.