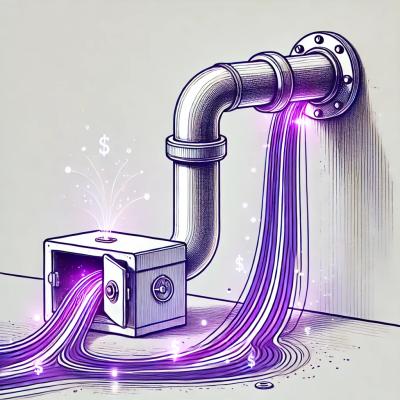
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
veritone-widgets
Advanced tools
As of v5.0.0, this package exports both React "smart components" and framework agnostic "widgets" for most components. When both are available, the smart component is the default export and the widget is a named export. For example, `import FilePicker, {
As of v5.0.0, this package exports both React "smart components" and framework agnostic "widgets" for most components. When both are available, the smart component is the default export and the widget is a named export. For example, import FilePicker, { FilePickerWidget } from 'veritone-widgets'
imports the smart component as FilePicker and the widget as FilePickerWidget. Smart components usually require redux reducers and sagas to be imported as well, see Using Smart Components
import { VeritoneApp, OAuthLoginButtonWidget, AppBarWidget } from 'veritone-widgets'
const app = VeritoneApp();
// a "log in with veritone" button
const oauthButton = new OAuthLoginButtonWidget({
// the ID of an existing element in your document where the button will appear
elId: 'login-button-widget',
// the ID of your Veritone application (found in Developer App)
clientId: 'my-client-id',
// the route in your app that will handle OAuth responses (more details below)
redirectUri: 'https://my-app.com/handle_oauth_callback',
// optional callbacks to retrieve the OAuth token for using outside of VeritoneApp:
onAuthSuccess: ({ OAuthToken }) => console.log(OAuthToken),
onAuthFailure: (error) => console.log(error)
});
// the Veritone app bar, which will receive auth after the user completes
// the oauth flow using the OAuthLoginButton
const appBar = new AppBarWidget({
elId: 'appBar-widget',
title: 'My App',
backgroundColor: '#4caf50',
profileMenu: true,
appSwitcher: true
});
import { OAuthLoginButton } from 'veritone-widgets'
render(
<OAuthLoginButton
clientId="my-client-id"
redirectUri="https://my-app.com/handle_oauth_callback"
/>
)
Because smart components are not rendered within the VeritoneApp widget framework, they require the user's app to have its own redux store with the components' reducers installed. Often, sagas are also included and must be started as part of the app's root saga.
reducer:
import {filePickerReducer} from 'veritone-widgets'
saga:
import {filePickerSaga} from 'veritone-widgets'
import {notificationsReducer} from 'veritone-widgets'
import {engineOutputExportReducer} from 'veritone-widgets'
User
, Auth
and Config
reducersIn your app, import the VeritoneSDKThemeProvider
from veritone-react-common
:
import {VeritoneSDKThemeProvider } from 'veritone-react-common'
then wrap your root component with <VeritoneSDKThemeProvider>
. If this is not possible, the @withVeritoneSDKThemeProvider
decorator is provided to wrap individual components.
Components can be customized using material-ui themes by passing a theme into the theme
prop of the provider. Passed-in themes will be merged with the default Veritone theme.
VeritoneApp
is a container for all the widgets and widget data in an app. Before using any widgets, you need to import and call it. Typically this will be done when your application is loaded and initialized.
import { VeritoneApp } from 'veritone-widgets'
const app = VeritoneApp();
Note: VeritoneApp
creates a singleton, so you do not need to manage the constructed app instance yourself. As long as VeritoneApp() has been imported and called at least once, you can retrieve the same instance by importing and calling VeritoneApp() again elsewhere in your app, if needed.
If you already have an OAuth token (because your app handles the client-side OAuth flow on its own), provide it by calling login()
on the returned app instance.
import { VeritoneApp } from 'veritone-widgets'
const app = VeritoneApp().login({
OAuthToken: 'my-token-here'
});
Calling login()
is usually not necessary; we provide an OAuthLoginButton
widget, which handles the OAuth token exchange automatically. See the instructions in the next section for more information.
After you've initialized the app framework by calling VeritoneApp()
, you can begin using widgets.
A "widget" is a self-contained frontend component. Widgets always render into an empty element which you provide. They are self-contained and handle rendering a UI, responding to user interactions, making api calls, and so on.
For example, the AppBar
widget renders the Veritone application toolbar, fetches the required data to render the Veritone app switcher and profile menu, and allows the developer to configure their application logo and title. When the user uses the app switcher or uses the profile menu to log out, the AppBar widget handles those events.
Widgets typically can be configured with a variety of options, but always require at least elId
. This is the id (string) of an element in your document, often a div
, into which the widget will be rendered. By applying styling to that div, you can position the widget around your app. The element specified in elId must exist in the document before you create the widget that will use it.
The first widget you are likely to include is OAuthLoginButton
. OAuthLoginButton
renders a "log in with Veritone" button that your users can click to start the OAuth authentication flow. At the end of that flow, your VeritoneApp instance will be authenticated and able to make requests to our API. You can also retrieve the oauth token to use in your own app.
Note: Unless you are handling the OAuth flow on your own and providing the token to VeritoneApp manually, the OAuthLoginButton
widget is required for Veritone widgets to work.
The actual code you write to use widgets will vary based on your framework of choice, but in general, it should be as follows.
// assuming VeritoneApp has already been initialized as described earlier, and given a document like:
<body>
...
<div id="login-button-widget" />
</body>
// you can render the login button to the document like this:
import { OAuthLoginButtonWidget } from 'veritone-widgets'
const oauthButton = new OAuthLoginButtonWidget({
// the ID of an existing element in your document where the button will appear
elId: 'login-button-widget',
// the ID of your Veritone application (found in Developer App)
clientId: 'my-client-id',
// the route in your app that will handle OAuth responses (more details below)
redirectUri: 'https://my-app.com/handle_oauth_callback',
// optional callbacks to retrieve the OAuth token for using outside of VeritoneApp:
onAuthSuccess: ({ OAuthToken }) => console.log(OAuthToken),
onAuthFailure: (error) => console.log(error)
});
When new OAuthLoginButtonWidget({ ... })
runs, the widget will appear on your page.
All Veritone apps should also include the AppBar widget.
<body>
<div id="appBar-widget" />
...
</body>
import { AppBarWidget } from 'veritone-widgets'
const appBar = new AppBarWidget({
elId: 'appBar-widget',
title: 'My App',
backgroundColor: '#4caf50',
profileMenu: true,
appSwitcher: true
});
For environments that do not support javascript module imports, widgets can also be included in an app via script tags.
<body>
<div id="appBar-widget"></div>
<script src="https://unpkg.com/veritone-widgets@^5/dist/umd/VeritoneApp.js"></script>
<script src="https://unpkg.com/veritone-widgets@^5/dist/umd/AppBar.js"></script>
<script>
const app = VeritoneApp.default();
const appBar = new AppBar.AppBarWidget({
elId: 'appBar-widget',
title: 'AppBar Widget',
profileMenu: true,
appSwitcher: true
});
</script>
</body>
As you can see, each widget is bundled individually to keep file sizes small. In addition, VeritoneApp is separately bundled and required to use widgets. Scripts can be accessed from the the unpkg cdn or downloaded and hosted on your own infrastructure. Unpkg supports semver ranges, or a specific version can be specified exactly.
Note that the OAuthLoginButton widget in the example above is being configured with five properties: elId, clientId, OAuthURI, onAuthSuccess and onAuthFailure. As mentioned earlier, an elId is required for every widget. OAuthURI, clientId, onAuthSuccess and onAuthFailure are specific configurable properties on the OAuthLoginButton. As it is in the example, configuration is always provided to the widget constructor.
Some widgets have methods which can be called on an instance of that widget. For example, the FilePicker widget has the methods pick()
and cancel()
to open and close the picker dialog, respectively.
this._picker = new FilePickerWidget({
elId: 'file-picker-widget',
accept: ['image/*'],
multiple: true
});
...
// call the pick() instance method on the widget
this._picker.pick(files => console.log(files))
In practice, a widget is just a wrapper around a React component. The easiest way to determine all the possible options for a given widget is to look at the PropTypes of its component. Remember to watch for console warnings which will indicate when an option was configured incorrectly, or when a required option was not provided.
The "storybook" dev environments in veritone-widgets and veritone-react-common have live examples of the various widgets and components.
The story.js
file in the root of each widget folder in veritone-widgets and in the root of each component folder in veritone-react-common show the code used to create the storybook pages. If you are using React to write your application, these can be used directly as a basis for your own implementation.
The PropTypes in the source files of the various widgets (in veritone-widgets) and their respective components (in veritone-react-common) generally correspond to configurable options. Likewise, instance methods for a widget will be defined on its widget class in veritone-widgets.
To remove a widget, call destroy()
on the instance
const oauthButton = new OAuthLoginButtonWidget({ ... });
...
oauthButton.destroy();
The current widgets are:
AppBar
The title and navigation bar common between all Veritone applications. Includes a logo, title, the Veritone app switcher and profile menu.
Options:
OAuthLoginButton The "Log in with Veritone" button and corresponding frontend logic to handle the OAuth2 authentication flow.
Options:
authCode
mode), the URL of your app server's OAuth callback endpoint.implicit
mode), the URL of your app frontend's OAuth callback endpoint.Requirements
Veritone provides two OAuth modes to suit different app requirements:
Implicit
, the default mode, is a frontend-only grant flow with no serverside requirement. Most apps will use this mode. This flow is much easier for apps to implement but has the downside of not returning a refresh token; users will need to log in again each time their token expires.
Auth Code
is a server-based grant flow, which uses your Veritone app's OAuth secret to retrieve both an auth token and refresh token. Auth code flow requires a server because the OAuth secret cannot be exposed to the client; the veritone-widets-server package is an example implementation, and more information can be found in its readme.
FilePicker
The Veritone file upload dialog. Handles selecting and uploading files to S3 for use on the Veritone platform.
Options:
Instance methods
pick(callback): open the filepicker dialog.
false
, the error that prevented the file from uploading, if anygetUrl
will be valid, in seconds.null
, the resulting S3 URL, if successful. Includes credentials that are valid for expires
seconds.null
, the resulting S3 URL, if successful. Does not include credentials and will need to be signed to be used.false
, a warning message if some (but not all) files failed to upload. A warning indicates that result
contains some successful upload result objects, and some that were not successful (unsuccessful objects will have error
populated with an error message, as noted above)false
, an error message, if all files failed to upload.cancel(): close the filepicker dialog. The callback provided to pick() will be called with (null, { cancelled: true })
.
Table
A Veritone table to display data.
Options:
(cellValue) => {}
menu
is true
, specifies action(s) to take when a menu option is clicked
(menuAction) => {}
['left', 'right', 'center']
(cellRow, cellColumn, cellData) => {}
focusedRow
(focusedRowData) => (string | react component)
({ start: firstRowIndex, end: lastRowIndex }) => {}
() => {}
EngineOutputExport
The Veritone export engine outputs full screen dialog. This will fetch the engines ran on a tdo/recording and allow the user to configure what file types are included in the export
Options:
(response) => {}
() => {}
Instance methods
config.dev.json
, fill in the missing fields with your own app's information (see our application quick-start guide for more info on how to create an app). Your app's URL
and redirect URL
should be set to the same value for the purposes of dev in this package. This default allows the environment to run with minimal config.yarn start
.Copyright 2019, Veritone Inc.
Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License.
FAQs
As of v5.0.0, this package exports both React "smart components" and framework agnostic "widgets" for most components. When both are available, the smart component is the default export and the widget is a named export. For example, `import FilePicker, {
The npm package veritone-widgets receives a total of 88 weekly downloads. As such, veritone-widgets popularity was classified as not popular.
We found that veritone-widgets demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 10 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.