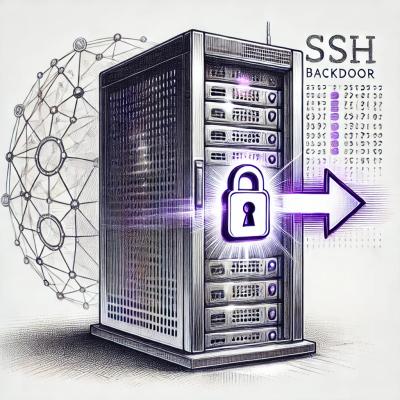
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
node's fs
module with sugar on top + native support.
This document describes only the sugar. For the meat and potatoes, refer to the nodejs docs.
This module respects the semver versioning methodology
npm install vigour-fs
Same as node's fs
module, e.g.
var fs = require('vigour-fs');
fs.readFile('somefile.txt', 'utf8', function(err, data) {
console.log(data, err)
})
fs
"API"All methods provided in vigour-fs
that also exist in fs
should behave exactly the same, except for these extra features:
fs.writeFile
accepts a mkdirp
option, which will create any missing subdirectories
fs.writeFile("path/with/inexistent/subdirectories/file.txt"
, "Hello World"
, { mkdirp: true }
, function (err) {
if (!err) {
console.log("File will be written to specified path, with subdirectories created as needed")
}
})
fs.readFile
and fs.writeFile
can accept a URL as path, in which case they will perform a GET request to that url.
fs.readFile('http://perdu.com', 'utf8', function (err, data) {
if (!err) {
console.log("html from perdu.com", data)
}
})
fs.writeFile('perdu.html', 'http://perdu.com', 'utf8', function (err) {
if (!err) {
console.log("perdu.html now contains the html from perdu.com")
}
})
This means fs.readFile
and fs.writeFile
come with extra options.
Option | Possible values | Default | Description |
---|---|---|---|
url |
| true | Whether to treat path as a url. If false, treats path as a local file path. Otherwise, treats path as a url if and only if it starts with http:// or https:// |
followRedirects |
| true | Whether to follow redirects (301, 302, 303, 305, 307, 308) |
maxTries | Positive integer above 0 | 1 | Number of attempts to make in total. If over 1, will retry the request if the response's status code is 500 or 503. Use the retryOn404 option to retry on 404 as well (not recommended). |
retryDelay | Positive integer | 500 | Time to wait before retrying the first time, in milliseconds. Subsequent attempts may use a different delay, dependant on the retryDelayType option. The delay may also be given by a 'retry-after' header accompanying a 503 response (see the respectRetryAfter option). In any case, the delay is capped by the maxRetryDelay option. |
retryDelayType |
| exp | Time to wait before retrying, in milliseconds, as a function of the attempt number (tryNb ) and the original delay (retryDelay ) specified in the retryDelay option
|
respectRetryAfter |
| true | Whether to respect the delay provided in a retry-after header when receiving a 503 response. True will respect the received delay, false will ignore it and use the retryDelayType and retryDelay options to determine the delay. In any case, the delay is capped by the maxRetryDelay option. |
maxRetryDelay | Positive integer above 0 | 60000 | Maximum time to wait before retrying, in milliseconds. Overrides Retry-After response-headers (see the respectRetryAfter ) option and normal retry delay increments (see the retryDelay ) option. |
retryOn404 |
| false | Whether to retry when response status code is 404. This looks stupid, and most of the time it will be. It is recommended to leave the default in for this one. |
fs.readFile('http://perdu.com'
, {
encoding: 'utf8'
, maxTries: 5
, retryDelayType: 'exp'
, retryDelay: 100
, retryOn404: true
, respectRetryAfter: true
}
, function(err, str) {
if (!err) {
console.log('Contents:', str)
}
})
fs.writeFile('file.txt'
, 'http://perdu.com'
, {
encoding: 'utf8'
, maxTries: 5
, retryDelayType: 'exp'
, retryDelay: 100
, retryOn404: true
, respectRetryAfter: true
}
, function(err) {
if (!err) {
console.log("file.txt now contains the html from perdu.com")
}
})
fs.writeFile('file.txt'
,'http://perdu.com'
, { url: false }
, function(err) {
if (!err) {
console.log('file.txt now contains the string "http://perdu.com"')
}
})
Remove a file or directory recursively
Argument | Type | Description |
---|---|---|
path | String | path |
callback | function (err) | Callback |
fs.remove('someDirectory', function(err) {
if (!err) {
console.log('success!')
}
})
fs.remove('someFile.txt', function(err) {
if (!err) {
console.log('success!')
}
})
Create any necessary subdirectories to allow path to exist. Also see fs.writeFile
's mkdirp option.
Argument | Type | Default | Description |
---|---|---|---|
path | String | path to create | |
options | Mode | 0777 | |
callback | function (err) | Callback |
fs.mkdirp('path/with/inexistent/subdirectories', function (err) {
if (!err) {
console.log("All subdirectories have been created")
}
})
Reads a file and JSON.parse
s it
fs.readJSON('somefile.json', function (err, obj) {
if (!err) {
console.log(obj.key)
}
})
JSON.stringify
s data and writes the resulting string to path
fs.writeJSON('somefile.json', { key: 'value' }, function (err) {
if (!err) {
console.log('somefile.json contains `{"key":"value"}`')
}
})
Reads the file found at path, JSON.parse
s it, passes the result as a single parameter to fn, JSON.stringify
s whatever fn returns, saves the resulting string to that same file and calls callback.
fs.editJSON('somefile.json'
, function (obj) {
obj.x += 1
return obj
}
, function (err) {
if (!err) {
console.log("done")
}
})
fn can also return a promise
var Promise = require('promise')
fs.editJSON('somefile.json'
, function (obj) {
return new Promise(function (resolve, reject) {
setTimeout(function () {
obj.x += 1
resolve(obj)
}, 500)
})
}
, function (err) {
if (!err) {
console.log("done")
}
})
Root directory of the filesystem
console.log(fs.rootDir)
platform | support |
---|---|
node | full |
iOS | partial |
Android | partial |
Windows Phone | partial |
other | none |
fs.readFile
fs.writeFile
fs.readdir
fs.mkdir
fs.rmdir
fs.rename
fs.unlink
fs.exists
fs.stat
(Only supports creation date, modification date and accessed date, all of which are Date objects)fs.remove
vigour-fs
is based on graceful-fs
fs.mkdirp
and the mkdirp
option available on fw.writeFile
and fs.writeJSON
use node-mkdirp) internallyfs.remove
uses rimraf internallyISC
FAQs
node's `fs` module with sugar on top + native support.
The npm package vigour-fs receives a total of 8 weekly downloads. As such, vigour-fs popularity was classified as not popular.
We found that vigour-fs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.