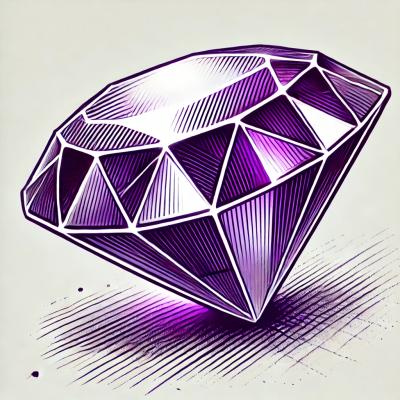
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
vigour-util
Advanced tools
npm install vigour-util
This is a collection of small utility functions which can be required individually.
npm test
expects vtest
to exist, so if you want to run the tests, you'll need to npm i -g vigour-test
first, which will allow the tests to be run in the context of a browser (Nightmare). See vigour-test
Checks whether provided parameter looks like a valid e-mail address
true
if val
is a valid e-mail address, false
otherwisevar isEmail = require('vigour-util/is/email')
isEmail('dev@vigour..io') // false
isEmail('dev@vigour.io') // true
Checks if a string looks like a hash generated by the hash
utility
true
if val
looks like a hash, false
otherwisevar isHash = require('vigour-util/is/hash')
isHash('asd654') // true
true
if in node context, false
otherwisevar isNode = require('vigour-util/is/node')
isNode() // true or false
This just calls lodash.isfinite
internally. See those docs
true
if value is a finite number, false
otherwisevar isNumber = require('vigour-util/is/number')
isNumber(2) // true
isNumber('2') // false
Checks whether provided parameter looks like a number
true
if val
looks like a number, false
otherwisevar isNumberLike = require('vigour-util/is/numberlike')
isNumberLike('2') // true
isNumberLike('a') // false
Checks whether provided argument is a stream
true
if val
is a stream, false
otherwisevar stream = require('stream')
var rs = new stream.Readable()
var isStream = require('vigour-util/is/stream')
isStream(rs) // true
Checks whether provided argument is a readable stream
true
if val
is a readable stream, false
otherwisevar stream = require('stream')
var rs = new stream.Readable()
var isStream = require('vigour-util/is/stream')
isStream.readable(rs) // true
Checks whether provided argument is a writable stream
true
if val
is a writable stream, false
otherwisevar stream = require('stream')
var rs = new stream.Readable()
var isStream = require('vigour-util/is/stream')
isStream.writable(rs) // false
Checks if we're running in a touch-enabled context
true
if we're in a touch-enabled context, false
otherwisevar isTouch = require('vigour-util/is/touch')
isTouch() // false unless you're using a touch-enabled device
Checks if a string is a valid url
val (string) - the string to check
[options] (object) - defaults to {}
options.requireProtocol {boolean} - set to true if you only want URLs with a protocol to be considered valid. Defaults to false
returns (boolean) valid - true
if val is a valid url, false
otherwise
var isUrl = require('vigour-util/is/url')
isUrl('http://perdu.com') // true
isUrl('boom') // false
Checks whether an object is a plain object (excludes streams, buffers, base and null) (Compatible with vigour-base
)
true
if obj is a plain object, false
otherwisevar isPlainObj = require('vigour/util/is/plainobj')
isPlainObj({}) // true
isPlainObj(new Base({})) // false
Checks if a property has been removed (Specific to vigour-base
)
true
if base property has been removed, false
otherwisevar isRemoved = require('vigour-util/is/removed')
var Base = require('vigour-base')
var base = new Base({ bad: true })
base.bad.remove()
isRemoved(base.bad) // true
Checks if a Base
object is empty (Specific to vigour-base
)
true
if obj
is considered empty, false
otherwisevar isEmpty = require('vigour-util/is/empty')
var Base = require('vigour-base')
isEmpty(new Base({})) // true
isEmpty(new Base({ awesome: true })) // false
Checks whether a key is part of an array, allowing for prefixed keys
Checks whether a key is part of an array, allowing for prefixed keys
true
if key is found in array, false
otherwisevar pathContains = require('vigour-util/path/contains')
pathContains(['a','awesome:b','c'], 'b') // true
A process-specific unique ID, generated on require
var uuid = require('vigour-util/uuid')
uuid.val // 'oj0beu'
Generates a unique ID
var uuid = require('vigour-util/uuid')
uuid.generate() // '14hdmru'
Defines new (or modifies existing) properties (using Object.defineProperty
) on an object passed to define
as this
, setting configurable: true
by default
var define = require('vigour-util/define')
var subject = {}
var props = [
{ one: true },
{ two: function () {
console.log('do nothing')
}
}
]
define.apply(subject, props)
Like Object.getOwnPropertyDescriptor
, but goes along the prototype chain and gets the descriptors for all properties.
var descriptors = require('vigour-util/descriptors')
descriptors({ a: 'a', b: 'b' })
/*
{
a: {
value: 'a',
writable: true,
enumerable: true,
configurable: true
},
b: {
value: 'a',
writable: true,
enumerable: true,
configurable: true
}
}
*/
Transforms a deep object into an object of single depth where keys are a path and values are leafs of the original object.
'.'
var flatten = require('vigour-util/flatten')
flatten({ a: { b: 'c', d: 'e' } }) // { 'a.b': 'c', 'a.d': 'e'}
flatten({ a: { b: 'c', d: 'e' } }, '/') // { 'a/b': 'c', 'a/d': 'e'}
Hashing utility optimized for speed, not collision avoidance. Produces alpha-numeric hashes between 5 and 7 characters long inclusively.
var hash = require('vigour-util')
hash('Any sting in the world!!!') // '16hck72'
Distinctly adds one or multiple items (in an object or array) to a target array. Doesn't duplicate items.
var include = require('vigour-util/include')
var target = [1]
include(target, [2, 1, 3]) // returns [2, 3]
console.log(target) // logs [1, 2, 3]
Obsolete: This function has been removed in version 2. Use lodash.merge
instead.
Like Babel's regenerator, but much more compact. Brought to you by Facebook, but bundled in vigour-util
for ease-of-use. See the docs
Obsolete: This function has been removed in version 2. Use lodash.set
instead.
Opposite of flatten
. Unflattens an object with delimited keys
var unflatten = require('vigour-util')
unflatten({
'a.b.c': 'd'
})
/*
{
a: {
b: {
c: 'd'
}
}
}
*/
Get's the referenced object (Specific to vigour-base
)
undefined
var Base = require('vigour-base')
var getReference = require('../../get/reference')
var a = new Base({})
var b = new Base(a)
getReference(b) // a
In node, modifies the behaviour of require
so that it ignores paths containing .css
, .less
, .scss
, .sass
, and any other paths indicated via the exclude
option.
Outside of node (browserify, webpack, etc.), this function does nothing.
[options] (object) - Options to further define the behaviour of require
:
true
to convert require('package.json')
to JSON.parse(require(process.cwd() + '/package.json'))
true
, will be excluded. If an array is provided, each element is treated exactly the same as options.exclude
and only paths which aren't excluded by any item will be require
d.var enhanceRequire = require('vigour-util/require')
enhanceRequire({
package: true,
exclude: '/scratch/'
})
require('styles.less') // ignored in node, processed elsewhere
// Don't forget to add a browserify transform or similar for non-node
var pkg = require('package.json') // pkg is the parsed package from the current working directory
require('')
enhanceRequire.restore()
FAQs
Utils from vigour
We found that vigour-util demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.