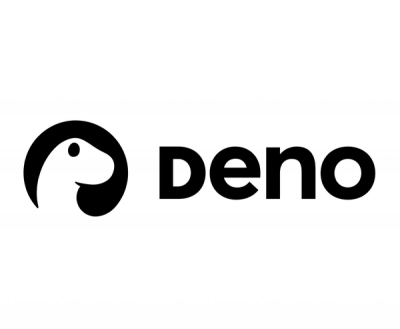
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
vite-plugin-fake-server
Advanced tools
A fake server plugin for Vite. Live Demo
ts
, js
, mjs
, cjs
, cts
, mts
files.npm install --save-dev vite-plugin-fake-server
Configure plugins in the configuration file of Vite, such as vite.config.ts
:
// vite.config.ts
import react from "@vitejs/plugin-react";
import { defineConfig } from "vite";
import { vitePluginFakeServer } from "vite-plugin-fake-server";
// https://vitejs.dev/config/
export default defineConfig({
plugins: [
react(),
// here
vitePluginFakeServer(),
],
});
By default, it is only valid in the development environment (enableDev = true
), and monitors in real time (watch = true
) all xxx.fake.{ts,js,mjs,cjs,cts,mts}
files in the fake(include = fake
) folder under the current project. When the browser has For the real requested link, the terminal will automatically print the requested URL (logger = true
).
For case details, please click this link to view packages/playground/react-sample/src
The most recommended way is to use a TypeScript file so you can use the defineFakeRoute
function to get good code hints.
The defineFakeRoute function parameters require the user to enter the route type as follows:
export interface FakeRoute {
url: string;
method?: HttpMethodType;
timeout?: number;
statusCode?: number;
statusText?: string;
headers?: OutgoingHttpHeaders;
response?: (processedRequest: ProcessedRequest, req: IncomingMessage, res: ServerResponse) => any;
rawResponse?: (req: IncomingMessage, res: ServerResponse) => void;
}
export type FakeRouteConfig = FakeRoute[] | FakeRoute;
export function defineFakeRoute(config: FakeRouteConfig) {
return config;
}
It should be noted that this way of introduction will cause
vite build
to fail.import { defineFakeRoute } from "vite-plugin-fake-server";
xxx.fake.ts
fileimport { faker } from "@faker-js/faker";
import Mock from "mockjs";
import { defineFakeRoute } from "vite-plugin-fake-server/client";
const adminUserTemplate = {
id: "@guid",
username: "@first",
email: "@email",
avatar: '@image("200x200")',
role: "admin",
};
const adminUserInfo = Mock.mock(adminUserTemplate);
export default defineFakeRoute([
{
url: "/api/get-user-info",
response: () => {
return adminUserInfo;
},
},
{
url: "/fake/get-user-info",
response: () => {
return {
id: faker.string.uuid(),
avatar: faker.image.avatar(),
birthday: faker.date.birthdate(),
email: faker.internet.email(),
firstName: faker.person.firstName(),
lastName: faker.person.lastName(),
sex: faker.person.sexType(),
role: "admin",
};
},
},
]);
xxx.fake.js
file/** @type {import("vite-plugin-fake-server").FakeRouteConfig} */
export default [
{
url: "/api/esm",
response: ({ query }) => {
return { format: "ESM", query };
},
},
{
url: "/api/response-text",
response: (_, req) => {
return req.headers["content-type"];
},
},
{
url: "/api/post",
method: "POST",
response: ({ body }) => {
return { ...body, timestamp: Date.now() };
},
},
];
xxx.fake.mjs
fileexport default {
url: "/api/mjs",
method: "POST",
statusCode: 200,
statusText: "OK",
response: () => {
return { format: "ESM" };
},
};
Type: string
Default: "fake"
Set the folder where the fake xxx.fake.{ts,js,mjs,cjs,cts,mts}
files is stored.
Type: string[]
Default: []
Exclude files in the include
directory.
document: https://github.com/mrmlnc/fast-glob#ignore
Type: string | boolean
Default: "fake"
Set the infix name used in the fake file name.
Type: boolean
Default: true
Set whether to listen to include
files.
Type: boolean
Default: true
Set whether to display the request log on the console.
In order to maintain logs and screen clearing strategies consistent with Vite style on the terminal, the vite-plugin-fake-server plugin will automatically read the following three parameters in the Vite configuration file or in the Vite command line.
A preview image:
Type: string[]
Default: ["ts", "js", "mjs", "cjs", "cts", "mts"]
Set the fake files extensions.
Type: number
Default: undefined
Set default response delay time.
Type: string
Default: ""
Set the root address of the request URL.
Type: OutgoingHttpHeaders
Default: {}
Set default headers for responses.
Type: boolean
Default: true
Set up the service simulator in the development environment.
Powered by Connect technology.
Type: boolean
Default: false
Set up the service simulator in the production environment.
Powered by XHook technology.
⚠️ The node module cannot be used in the fake file, otherwise the production environment will fail.As an alternative to keep consistent with the development environment, you can build a standalone deployment server, see build option.
Compared with the development environment, the API interface defined in the production environment does not have a rawResponse
function. The response function does not have the second parameter request
and the third parameter response
.
export interface FakeRoute {
url: string;
method?: HttpMethodType;
timeout?: number;
statusCode?: number;
statusText?: string;
headers?: OutgoingHttpHeaders;
response?: (processedRequest: ProcessedRequest) => any;
}
Type: boolean | ServerBuildOptions
Default: false
Set whether to export a independently deployable fake service(only valid in build mode).
interface ServerBuildOptions {
/**
* @description Server port
* @default 8888
*/
port?: number;
/**
* Directory relative from `root` where build output will be placed. If the
* directory exists, it will be removed before the build.
* @default "fakeServer"
*/
outDir?: string;
}
# packages/vite-plugin-fake-server
npm run build:watch
# packages/playground/react-sample
npm run dev
# packages/vite-plugin-fake-server
npm run build:watch
# packages/playground/react-sample
npm run build
npm run preview
MIT License © 2023-Present Condor Hero
FAQs
A fake server plugin for Vite.
The npm package vite-plugin-fake-server receives a total of 1,123 weekly downloads. As such, vite-plugin-fake-server popularity was classified as popular.
We found that vite-plugin-fake-server demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.