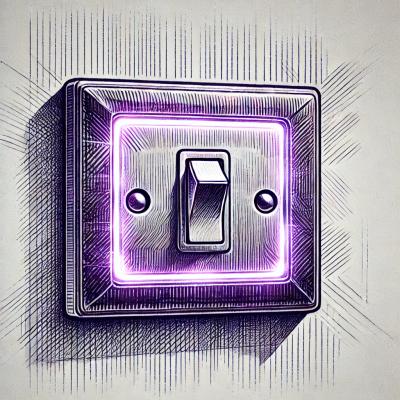
Research
Security News
Kill Switch Hidden in npm Packages Typosquatting Chalk and Chokidar
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
vite-plugin-mock-server
Advanced tools
Provide local mocks for Vite.
A mock server plugin for Vite, developed based on TypeScript. And support using TypeScript and JavaScript to write Mock API. When the Mock API file is modified, it will be hot updated automatically.
node version: >=12.0.0
vite version: >=2.0.0
# if using npm
npm i vite-plugin-mock-server -D
# if using yarn
yarn add vite-plugin-mock-server -D
cd ./example
npm install
npm run dev
import { defineConfig } from 'vite'
import vue from '@vitejs/plugin-vue'
import mockServer from 'vite-plugin-mock-server'
export default defineConfig({
plugins: [
vue(),
mockServer({
logLevel: 'info'
})
]
})
mockModules
Ignore manual configuration, it will be filled in automatically.
export type MockOptions = {
logLevel?: 'info' | 'error' | 'off'
urlPrefixes?: string[]
mockJsSuffix?: string
mockTsSuffix?: string
mockRootDir?: string
mockModules?: string[]
noHandlerResponse404?: boolean
}
// default options
const options: MockOptions = {
logLevel: 'info',
urlPrefixes: [ '/api/' ],
mockRootDir: './mock',
mockJsSuffix: '.mock.js',
mockTsSuffix: '.mock.ts',
noHandlerResponse404: true,
mockModules: []
}
export type MockFunction = {
(req: Connect.IncomingMessage, res: http.ServerResponse, urlVars?: { [key: string]: string }): void
}
export type MockHandler = {
pattern: string,
method?: string,
handle: MockFunction
}
The pattern
is an ant-style path pattern string, use @howiefh/ant-path-matcher to match the pattern
and request URL
.
// example/mock/es.mock.ts
const mocks: MockHandler[] = [
{
pattern: '/api/test1/1',
handle: (req, res) => {
res.end('Hello world!' + req.url)
}
},
{
pattern: '/api/test1/*',
handle: (req, res) => {
res.end('Hello world!' + req.url)
}
},
{
pattern: '/api/test1/users/{userId}',
handle: (req, res, pathVars) => {
const data = {
url: req.url,
pathVars: pathVars
}
res.setHeader('Content-Type', 'application/json')
res.end(JSON.stringify(data))
}
},
{
pattern: '/api/test1/users/{userId}/{blogId}',
handle: (req, res, pathVars) => {
const data = {
url: req.url,
pathVars: pathVars
}
res.setHeader('Content-Type', 'application/json')
res.end(JSON.stringify(data))
}
}
]
export default mocks
// example/mock/apis/es2.mock.ts
import { MockHandler } from 'vite-plugin-mock-server'
export default (): MockHandler[] => [
{
pattern: '/api/test2/1',
handle: (req, res) => {
res.end('Hello world!' + req.url)
}
},
{
pattern: '/api/test2/2',
handle: (req, res) => {
res.end('Hello world!' + req.url)
}
}
]
// example/mock/cjs.mock.js
module.exports = [
{
pattern: '/api/merchant1',
method: 'GET',
handle: (req, res) => {
res.end('merchant1:' + req.url)
}
},
{
pattern: '/api/merchant2',
method: 'GET',
handle: (req, res) => {
res.end('merchant2:' + req.url)
}
},
{
pattern: '/api/merchant2',
method: 'GET',
handle: (req, res) => {
res.end('merchant3:' + req.url)
}
}
]
// example/mock/apis/cjs2.mock.js
module.exports = [
{
pattern: '/api/hello1',
method: 'GET',
handle: (req, res) => {
res.end('hello1:' + req.url)
}
},
{
pattern: '/api/hello2',
method: 'GET',
handle: (req, res) => {
res.end('hello2:' + req.url)
}
},
{
pattern: '/api/hello3',
method: 'GET',
handle: (req, res) => {
res.end('hello2:' + req.url)
}
}
]
MIT
FAQs
Vite mock server plugin
The npm package vite-plugin-mock-server receives a total of 3,097 weekly downloads. As such, vite-plugin-mock-server popularity was classified as popular.
We found that vite-plugin-mock-server demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.