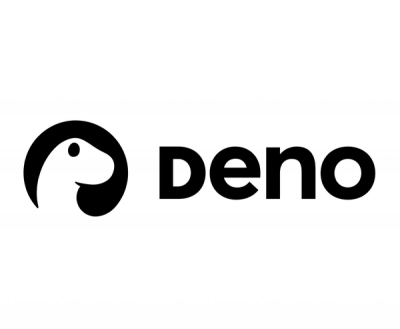
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Vo is a control flow library for minimalists.
Vo supports 2 kinds of asynchronous flows:
Both of these flows support both parallel and serial execution
function * get (url) {
return yield fetch(url)
}
function map (responses) {
return responses.map(res => res.status)
}
vo([
fetch('https://standupjack.com'),
fetch('https://google.com')
], map).then(function (statuses) {
assert.deepEqual([ 200, 200 ])
})
Coming soon! Check out the comprehensive test suite for now.
Now you can run generators top-level with the runtime:
index.js
var res = yield superagent.get('http://google.com')
console.log(res.status) // 200
vo index.js
Use co-bind just to be safe.
We have a comprehensive test suite. Here's how you run it:
npm install
make test
MIT
FAQs
Vo is a control flow library for minimalists
The npm package vo receives a total of 85 weekly downloads. As such, vo popularity was classified as not popular.
We found that vo demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.