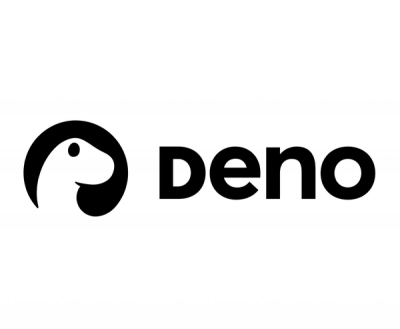
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
vscode-nls
Advanced tools
The vscode-nls package is a localization library designed for use with Visual Studio Code extensions. It provides a simple way to localize strings in your extension, making it easier to support multiple languages.
Initialization
This feature allows you to initialize the localization library with a specific locale. The `config` method sets up the locale and returns a function that can be used to localize strings.
const nls = require('vscode-nls');
const localize = nls.config({ locale: 'en' })();
Localizing Strings
This feature allows you to localize strings using a key and a default message. The `localize` function takes a key and a default message, and returns the localized string based on the current locale.
const message = localize('key', 'Default message');
Loading Message Bundles
This feature allows you to load message bundles for localization. The `loadMessageBundle` method loads the message bundle for the current locale, which can then be used to localize strings.
const nls = require('vscode-nls');
const localize = nls.loadMessageBundle();
i18next is a popular internationalization framework for JavaScript. It provides a comprehensive set of features for localization, including support for multiple languages, pluralization, and interpolation. Compared to vscode-nls, i18next is more feature-rich and can be used in a variety of environments, not just Visual Studio Code extensions.
react-intl is a library for internationalizing React applications. It provides components and APIs for formatting dates, numbers, and strings, as well as handling pluralization and translations. While vscode-nls is focused on Visual Studio Code extensions, react-intl is specifically designed for use with React applications.
Globalize is a library for internationalization and localization in JavaScript. It provides support for formatting and parsing dates, numbers, and currencies, as well as message translation. Globalize is more general-purpose compared to vscode-nls, which is tailored for Visual Studio Code extensions.
FAQs
NPM module to externalize and localize VSCode extensions
The npm package vscode-nls receives a total of 910,922 weekly downloads. As such, vscode-nls popularity was classified as popular.
We found that vscode-nls demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 10 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.